UnstructGrid: Set Scalar Settings on a Loaded Model
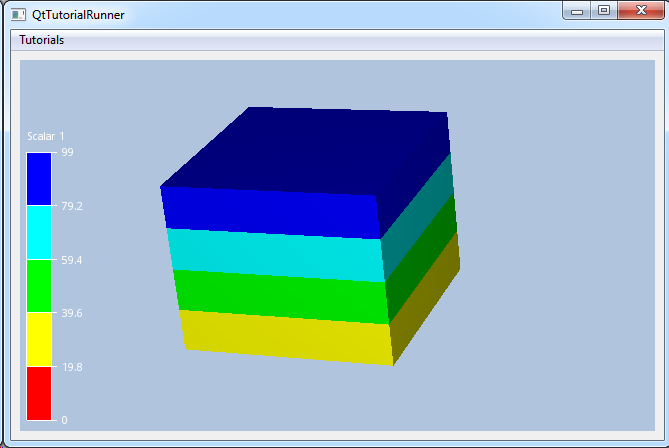
The scalar result can be configured to make a better visual appearance of your result data, for instance settings such as legend scheme, legend range and filtering.
Each scalar result has its own settings.
This tutorial shows how to change scalar settings for visualized result.
The demo model file (100x100x100.vtf) contains a simple geometry and a scalar result.
This tutorial will show the scalar as fringes on the model, and set some scalar settings for demonstration.
Set number of legend levels to 5
Set color scheme to “Normal Inverted”.
Filter away all values under 20.
Load the file and setup the model specification to show the result. For a more thorough explanation on creating a data source from a file interface and setup the model specification, see the Load VFT tutorial: UnstructGrid: Load Model from File and Set Up Model Specification
Note
This tutorial expect the application to have a correctly configured cee::vis::View
in place. See demo applications on how to set up a cee::vis::View
in your application.
Load model
Create the model and a VTF file interface data source. Open the file and set the created data source in the model.
cee::PtrRef<cee::ug::UnstructGridModel> ugModel = new cee::ug::UnstructGridModel();
cee::PtrRef<cee::ug::DataSourceVTF> source = new cee::ug::DataSourceVTF(42);
cee::Str vtfFile = TutorialUtils::testDataDir() + "100x100x100.vtf";
if (!source->open(vtfFile))
{
// VTF file not found
return;
}
Setup model specification
The model specification in this tutorial is set up with the first state and the model’s only scalar result set as fringes.
Use the information retrieved from the data source directory to set up the model specification.
std::vector<cee::ug::StateInfo> stateInfos = source->directory()->stateInfos();
int stateId = stateInfos[0].id();
ugModel->modelSpec().setStateId(stateId);
std::vector<cee::ug::ResultInfo> scalarResultInfos = source->directory()->scalarResultInfos();
int scalarId = scalarResultInfos[0].id();
ugModel->modelSpec().setFringesResultId(scalarId);
Set result visibility
Toggle on fringes visibility for all parts. For easy access
to the settings for all available parts in a model, use the cee::ug::PartSettingsIterator
.
cee::ug::PartSettingsIterator it(ugModel.get());
while (it.hasNext())
{
cee::ug::PartSettings* partSettings = it.next();
partSettings->setFringesVisible(true);
}
Set scalar settings
Available scalar settings:
Range
Number of levels
Color scheme
Legend lines/text colors
Filtering
In this tutorial we will set number of levels, color scheme and filtering.
Get scalar settings for a specific scalar result by calling
cee::ug::UnstructGridModel::scalarSettings()
with the requested scalar result id.
cee::ug::ScalarSettings* settings = ugModel->scalarSettings(scalarId);
Set number of legend levels to 5.
settings->colorMapper().setTypeFilledContoursUniform(5);
Set the color scheme to normal inverted.
settings->colorMapper().setColorScheme(cee::ug::ColorMapper::NORMAL_INVERTED);
Use filter to only show values elements where the result value is in the interval from 20 to 99. (The scalar result in this data source has a minimum value of 0 and a maximum value of 99.)
Toggle on filtering.
settings->setFringesElementFilteringVisibleRange(20, 99);
Set up the created model
The model is ready to use and can be added to the view. Exactly where the view exists
depends on the platform and solution. These examples uses Qt and the view is set up
in a cee::qt::ViewerWidget
.
cee::vis::View* gcView = getTutorialView();
gcView->addModel(ugModel.get());
ugModel->updateVisualization();
See the complete source code here: