Snapshots
The main building block of the Report component is a Snapshot
. A snapshot is a capture of
information from your application at a given setup.
A snapshot contains either an image (for instance a snapshot of the view), a 3D model with results and feature
extractions, a 2D plot/graph or a tabular result listing. Each snapshot also has a title, a description and a set of
field values.
A collection of captured snapshots can be saved to a Repository
for later access and
further work.
This can also be used when comparing analysis cases, where a stored repository can be used as a reference case.
All snapshots contain a title, a description and a set of field values. In addition, it contains data of one of three types; Image, 3D model or tabular data. You specify which of these three types the snapshot is in the Snapshot constructor.
Image Snapshot
The data carrier in an image snapshot is a cee::Image. This can be a snapshot of the view or, for instance, an imported picture.
cee::PtrRef<cee::Image> image = new cee::Image();
m_viewer->view()->renderToImage(image.get());
cee::PtrRef<cee::rep::Snapshot> snapshot = new cee::rep::Snapshot(cee::rep::Snapshot::OBJECT_IMAGE);
snapshot->setImage(image.get());
snapshot->setTitle("My Image Title");
3D Model Snapshot
The data carrier for a 3D model is a cee::ug::MemoryFile (memory VTFx file). The 3D model is shown in PowerPoint and Word using the free Ceetron 3D Plugin and in HTML using the Ceetron Cloud technology.
Read more about the Ceetron VTFx file format and Ceetron’s free Viewers: Share Everywhere with VTFx Files and Ceetron Viewers
The VTFx model file is created in memory and embedded into the Word document/PowerPoint presentation. This enables you
to easily share your Office document without being afraid of broken links. (The VTFx file will also be embedded into the
repository file if this is saved.) If the user does not want to embed the VTFx files into the presentation/document,
this feature can be turned off with
ReportCreatorWord::setEmbedVTFx(false)
. Default is true.
cee::exp::ExportVTFx exporter(cee::exp::ExportVTFx::DISPLAY_MODEL_ONLY, model);
// Add properties and resources from model and view
cee::PtrRef<cee::PropertySetCollection> propColl = new cee::PropertySetCollection;
cee::PtrRef<cee::ImageResources> resources = new cee::ImageResources;
cee::exp::PropertyBuilderVTFx propBuilder(propColl.get(), resources.get());
propBuilder.addFromModel(*model);
propBuilder.addFromView(*view);
exporter.addProperties(*propColl);
exporter.addResources(*resources);
cee::PtrRef<cee::ug::VTFxMemoryFile> memoryFile = new cee::ug::VTFxMemoryFile();
exporter.saveCase(memoryFile.get());
cee::PtrRef<cee::rep::Snapshot> snapshot = new cee::rep::Snapshot(cee::rep::Snapshot::OBJECT_VTFX);
snapshot->setModelVTFx(memoryFile.get());
snapshot->setTitle("My VTFx Model Title");
Table Snapshot
A Table
contains a two dimensional array of strings. A table can store, for instance,
a series of pick information or plot data. The size of the table is defined upon construction and cannot be changed
afterwards.
The table data can be used either in a plain textual table or, if it contains plot data, it can be used to create a
chart object in Word or PowerPoint. The figure is showing one table snapshot as both a table and a chart in a Word
document.
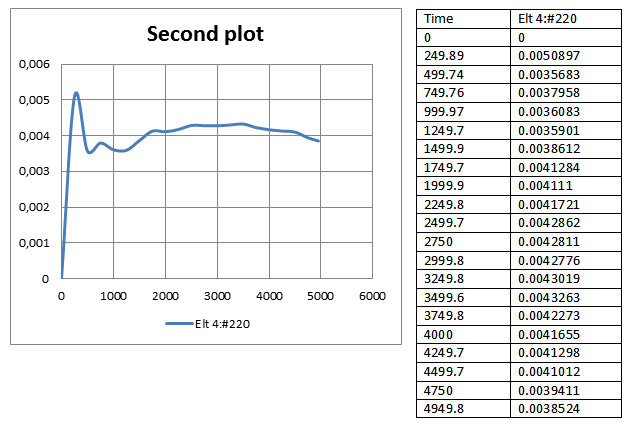
The first row will be treated as column titles when inserted into a table.
When used to populate a chart, the first column will be used on the x-axis and each of the
remaining columns will represent a new data series. The elements in the first row will be
set as the names of each data series. You can specify optional x and y axis titles
using setChartYAxisTitle()
and
setChartXAxisTitle()
.
Note! If the table are to be used as chart data, the number value MUST be passed along using a ‘.’ as the decimal point for the MS Office chart object to recognize it as a number!
cee::PtrRef<cee::rep::Table> table = new cee::rep::Table(7, 2);
table->setChartXAxisTitle("Time steps");
table->setChartYAxisTitle("Temperature");
table->setValue(0, 1, "Heat");
table->setValue(1, 0, "1");
table->setValue(1, 1, "15");
table->setValue(2, 0, "2");
table->setValue(2, 1, "35");
table->setValue(3, 0, "3");
table->setValue(3, 1, "66");
table->setValue(4, 0, "4");
table->setValue(4, 1, "42");
table->setValue(5, 0, "5");
table->setValue(5, 1, "25");
table->setValue(6, 0, "6");
table->setValue(6, 1, "20");
cee::PtrRef<cee::rep::Snapshot> snapshot = new cee::rep::Snapshot(cee::rep::Snapshot::OBJECT_TABLE);
snapshot->setTitle("My Chart Title");
snapshot->setTable(table.get());