Geometry: Create a Geometry Model
The geometry model
offers optimized part drawing with different parts and effects.
This example creates two triangle parts and two polyline parts. The second triangle and the second polyline will have the halo effect applied.
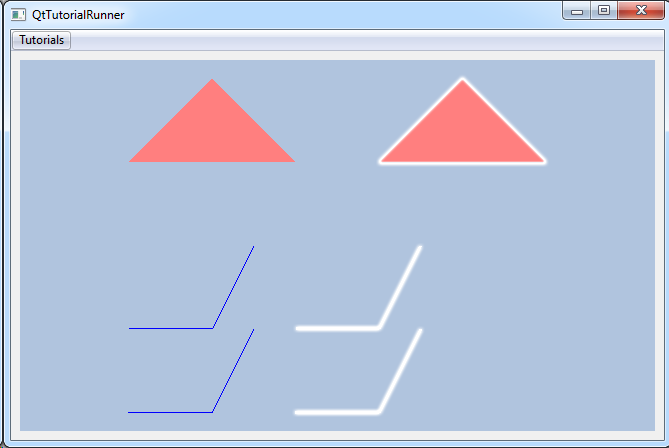
void TutorialRunnerMainWindow::createGeometryModel()
{
//--------------------------------------------------------------------------
// Create a geometry model with triangles and lines
//--------------------------------------------------------------------------
cee::vis::View* gcView = getTutorialView();
gcView->removeAllModels();
// Create the geometry model
cee::PtrRef<cee::geo::GeometryModel> gcgeoModel = new cee::geo::GeometryModel();
gcView->addModel(gcgeoModel.get());
//--------------------------------------------------------------------------
// Create vertices and indices for all parts
//--------------------------------------------------------------------------
// Triangle vertices and indices
std::vector<cee::Vec3d> triangleVertices;
triangleVertices.push_back(cee::Vec3d(0.0, 0.0, 2.0));
triangleVertices.push_back(cee::Vec3d(2.0, 0.0, 2.0));
triangleVertices.push_back(cee::Vec3d(1.0, 0.0, 3.0));
std::vector<unsigned int> triangleIndices = { 0, 1, 2 };
// First polyline vertices
std::vector<cee::Vec3d> firstPolylinesVertices;
firstPolylinesVertices.push_back(cee::Vec3d(0.0, 0.0, 0.0));
firstPolylinesVertices.push_back(cee::Vec3d(1.0, 0.0, 0.0));
firstPolylinesVertices.push_back(cee::Vec3d(1.5, 0.0, 1.0));
firstPolylinesVertices.push_back(cee::Vec3d(0.0, 0.0, -1.0));
firstPolylinesVertices.push_back(cee::Vec3d(1.0, 0.0, -1.0));
firstPolylinesVertices.push_back(cee::Vec3d(1.5, 0.0, 0.0));
// Second polyline vertices
std::vector<cee::Vec3d> secondPolylinesVertices;
secondPolylinesVertices.push_back(cee::Vec3d(2.0, 0.0, 0.0));
secondPolylinesVertices.push_back(cee::Vec3d(3.0, 0.0, 0.0));
secondPolylinesVertices.push_back(cee::Vec3d(3.5, 0.0, 1.0));
secondPolylinesVertices.push_back(cee::Vec3d(2.0, 0.0, -1.0));
secondPolylinesVertices.push_back(cee::Vec3d(3.0, 0.0, -1.0));
secondPolylinesVertices.push_back(cee::Vec3d(3.5, 0.0, 0.0));
// Polyline indices
std::vector<unsigned int> firstLineIndices = { 0, 1, 2 };
std::vector<unsigned int> secondLineIndices = { 3, 4, 5 };
std::vector<std::vector<unsigned int> > polylinesIndices;
polylinesIndices.push_back(firstLineIndices);
polylinesIndices.push_back(secondLineIndices);
//--------------------------------------------------------------------------
// Create all data parts
//--------------------------------------------------------------------------
// Create a triangle data part using the vertices and connectivities
cee::PtrRef<cee::geo::DataIndexedTriangles> triangleDataPart = new cee::geo::DataIndexedTriangles();
triangleDataPart->setVertices(triangleVertices);
triangleDataPart->setIndices(triangleIndices);
// Create first polyline data part using the vertices and connectivities
cee::PtrRef<cee::geo::DataIndexedPolylines> firstLinePartData = new cee::geo::DataIndexedPolylines();
firstLinePartData->setVertices(firstPolylinesVertices);
firstLinePartData->setPolylinesIndices(polylinesIndices);
// Create second polyline data part using the vertices and connectivities
cee::PtrRef<cee::geo::DataIndexedPolylines> secondLinePartData = new cee::geo::DataIndexedPolylines();
secondLinePartData->setVertices(secondPolylinesVertices);
secondLinePartData->setPolylinesIndices(polylinesIndices);
//--------------------------------------------------------------------------
// Create all parts
//--------------------------------------------------------------------------
// First triangle
cee::PtrRef<cee::geo::Part> trianglePart = new cee::geo::Part();
trianglePart->setData(triangleDataPart.get());
// Second triangle (transformed)
cee::PtrRef<cee::geo::Part> transformedTrianglePart = new cee::geo::Part();
transformedTrianglePart->setData(triangleDataPart.get());
transformedTrianglePart->setTransformation(cee::Mat4d::fromTranslation(cee::Vec3d(3.0, 0.0, 0.0)));
// First polyline
cee::PtrRef<cee::geo::Part> firstLinePart = new cee::geo::Part();
firstLinePart->setData(firstLinePartData.get());
// Second polyline
cee::PtrRef<cee::geo::Part> secondLinePart = new cee::geo::Part();
secondLinePart->setData(secondLinePartData.get());
//--------------------------------------------------------------------------
// Create effects
//--------------------------------------------------------------------------
// Create a color effect
cee::PtrRef<cee::geo::EffectColor> triangleColorEffect = new cee::geo::EffectColor();
triangleColorEffect->setColor(cee::Color3f(1.0f, 0.0f, 0.0f));
// Create a color effect
cee::PtrRef<cee::geo::EffectColor> lineColorEffect = new cee::geo::EffectColor();
lineColorEffect->setColor(cee::Color3f(0.0, 0.0, 1.0));
// Create a halo effect
cee::PtrRef<cee::geo::EffectHalo> haloEffect = new cee::geo::EffectHalo();
//--------------------------------------------------------------------------
// Add effects to parts
//--------------------------------------------------------------------------
// First triangle has color effect
trianglePart->settings().addEffect(triangleColorEffect.get());
// Second triangle has color and a halo effect
transformedTrianglePart->settings().addEffect(triangleColorEffect.get());
transformedTrianglePart->settings().addEffect(haloEffect.get());
// First polyline has line effect
firstLinePart->settings().addEffect(lineColorEffect.get());
// Second polyline has line and halo effect
secondLinePart->settings().addEffect(lineColorEffect.get());
secondLinePart->settings().addEffect(haloEffect.get());
//--------------------------------------------------------------------------
// Add all parts to model
//--------------------------------------------------------------------------
gcgeoModel->addPart(trianglePart.get());
gcgeoModel->addPart(transformedTrianglePart.get());
gcgeoModel->addPart(firstLinePart.get());
gcgeoModel->addPart(secondLinePart.get());
cee::BoundingBox bb = gcView->boundingBox();
gcView->camera().fitView(bb, cee::Vec3d(0, 1, 0), cee::Vec3d(0, 0, 1));
gcView->camera().inputHandler()->setRotationPoint(bb.center());
gcView->requestRedraw();
}