Append Case to VTFx
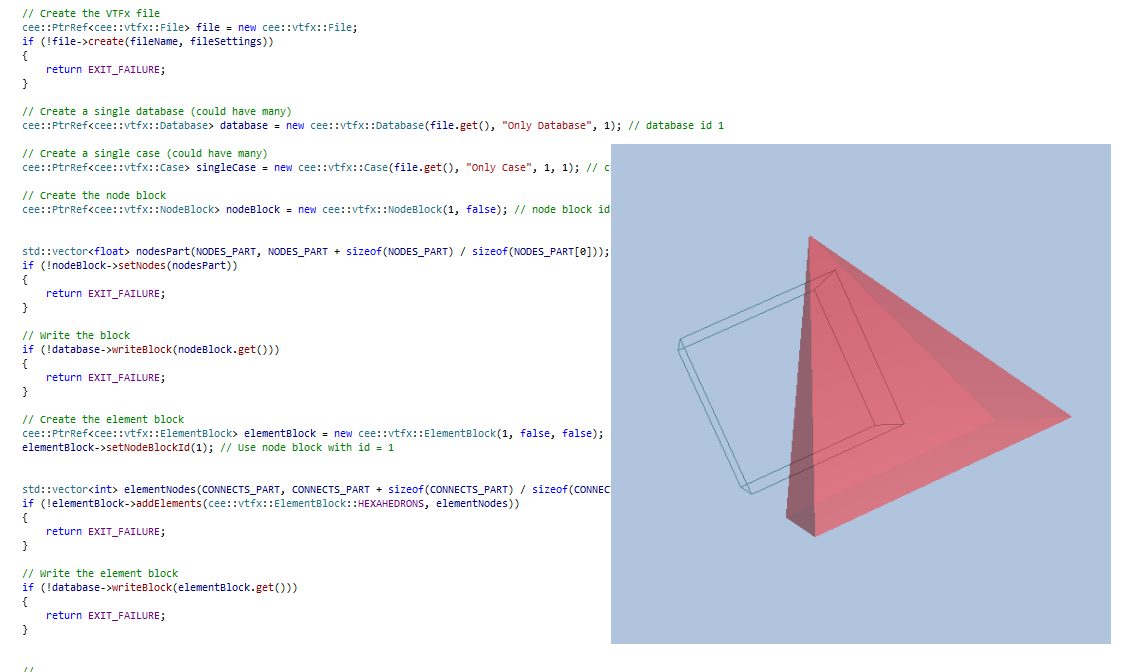
This example opens an existing VTFx file in append mode and adds an additional case to it.
The appended case uses the same database, and sets the following properties:
Part 1: draw style set to line
Part 2: set color and opacity
The VTFx file can now be opened in a viewer supporting multiple cases (for instance CEETRON Envision Demo Viewer). It will contain two cases. The first (unchanged) case and in addition the newly appended case with modified draw style and opacity.
//--------------------------------------------------------------------------------------------------
// Creates case properties
//--------------------------------------------------------------------------------------------------
bool createProperties(cee::vtfx::Case* currentCase)
{
cee::PtrRef<cee::PropertySetCollection> vtfxProps = new cee::PropertySetCollection;
// Set part (id = 1) to use line as draw style
cee::PtrRef<cee::PropertySet> partSettings = new cee::PropertySet("part_settings");
partSettings->setValue("context_geometry_index", static_cast<unsigned int>(0));
partSettings->setValue("context_part_id", 1);
partSettings->setValue("draw_style", "line");
vtfxProps->addPropertySet(partSettings.get());
// Set part (id = 2) to use red color and opacity
cee::PtrRef<cee::PropertySet> partSettings2 = new cee::PropertySet("part_settings");
partSettings2->setValue("context_geometry_index", static_cast<unsigned int>(0));
partSettings2->setValue("context_part_id", 2);
partSettings2->setValue("color", cee::Color3f(1.0f, 0.0f, 0.0f));
partSettings2->setValue("opacity", 0.3f);
vtfxProps->addPropertySet(partSettings2.get());
// Set the properies
return currentCase->setProperties(vtfxProps.get());
}
cee::PtrRef<cee::Instance> g_componentInstance;
//--------------------------------------------------------------------------------------------------
// Main function, program entry point
//--------------------------------------------------------------------------------------------------
int main()
{
// Initialize components and set up logging to console
// -------------------------------------------------------------------------
g_componentInstance = cee::CoreComponent::initialize(HOOPS_LICENSE);
cee::vtfx::VTFxComponent::initialize(g_componentInstance.get());
cee::PtrRef<cee::LogDestinationConsole> log = new cee::LogDestinationConsole();
cee::CoreComponent::logManager()->setDestination(log.get());
cee::CoreComponent::logManager()->setLevel("", 3); // Log errors, warnings and info
// Create the VTFx file
// -------------------------------------------------------------------------
cee::Str fileName = "ExampleAppend.vtfx";
// Make copy original file
std::ifstream src("../../DemoFiles/SimpleExample.vtfx", std::ios::binary);
if (!src.good())
{
return EXIT_FAILURE;
}
std::ofstream dst(fileName.toStdString().c_str(), std::ios::binary);
dst << src.rdbuf();
src.close();
dst.close();
cee::PtrRef<cee::vtfx::File> file = new cee::vtfx::File;
if (!file->openForAppend(fileName))
{
return EXIT_FAILURE;
}
// Get the first database from file
cee::PtrRef<cee::vtfx::Database> db = file->database(0);
// Create a single case (could have many)
// -------------------------------------------------------------------------
cee::PtrRef<cee::vtfx::Case> newCase = new cee::vtfx::Case(file.get(), "Appended Case", file->unusedFileCaseId(), db->id());
if (!createProperties(newCase.get()))
{
return EXIT_FAILURE;
}
// Finally close the file
// -------------------------------------------------------------------------
if (!file->close())
{
return EXIT_FAILURE;
}
std::cout << "Exported successfully to file: " << fileName.toStdString() << std::endl;
std::cout << std::endl << "Press enter to exit..." << std::endl;
std::cin.ignore();
return EXIT_SUCCESS;
}