Visualization: Draw Bounding Box Using Markup Model
The MarkupModel enables the user to draw custom markup figures and texts into the model. Markup can, for instance, be used to:
Draw measure lines (distance/angle/…)
Add annotations as labels
Position 2D symbols in the model
This example loads the demo file and draws the models bounding box as a markup model in the view. The bounding box is draw as yellow lines.
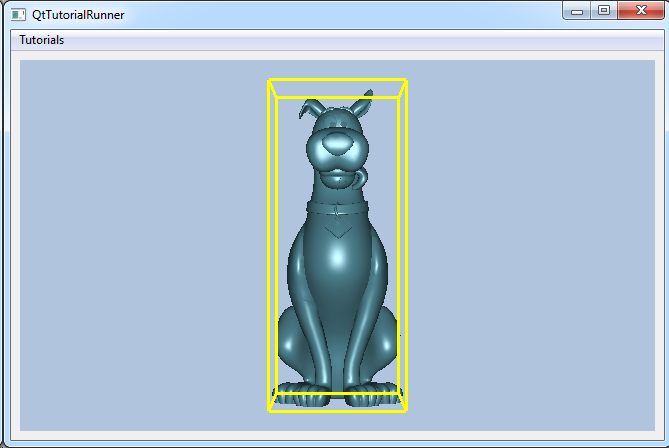
void TutorialRunnerMainWindow::createMarkup()
{
//--------------------------------------------------------------------------
// Load an UnstructGridModel from file and draw a bounding box
//--------------------------------------------------------------------------
cee::vis::View* gcView = getTutorialView();
// Create the model and data source
cee::PtrRef<cee::ug::UnstructGridModel> ugModel = new cee::ug::UnstructGridModel();
gcView->setModelOpenGLCapabilitiesFromView(ugModel.get());
cee::PtrRef<cee::ug::DataSourceVTFx> source = new cee::ug::DataSourceVTFx(42);
// Open the vtfx file
cee::Str vtfxFile = TutorialUtils::testDataDir() + "scoobs.vtfx";
if (!source->open(vtfxFile))
{
// VTFx file not found
return;
}
// Add the data source to the model
ugModel->setDataSource(source.get());
// Set the last state as current
std::vector<cee::ug::StateInfo> stateInfos = source->directory()->stateInfos();
if (stateInfos.size() > 0)
{
int lastStateId = stateInfos[stateInfos.size() - 1].id();
ugModel->modelSpec().setStateId(lastStateId);
}
// Add model to view. Ensure that old models are removed first
gcView->removeAllModels();
gcView->addModel(ugModel.get());
ugModel->updateVisualization();
// Create createMarkup model for drawing the bounding box
cee::PtrRef<cee::vis::MarkupModel> markupModel = new cee::vis::MarkupModel();
gcView->setModelOpenGLCapabilitiesFromView(markupModel.get());
// Get all corner points of the bounding box
cee::BoundingBox bb = gcView->boundingBox();
cee::Vec3d p1(bb.minimum().x(), bb.minimum().y(), bb.minimum().z());
cee::Vec3d p2(bb.minimum().x(), bb.maximum().y(), bb.minimum().z());
cee::Vec3d p3(bb.minimum().x(), bb.maximum().y(), bb.maximum().z());
cee::Vec3d p4(bb.minimum().x(), bb.minimum().y(), bb.maximum().z());
cee::Vec3d p5(bb.maximum().x(), bb.minimum().y(), bb.minimum().z());
cee::Vec3d p6(bb.maximum().x(), bb.maximum().y(), bb.minimum().z());
cee::Vec3d p7(bb.maximum().x(), bb.maximum().y(), bb.maximum().z());
cee::Vec3d p8(bb.maximum().x(), bb.minimum().y(), bb.maximum().z());
// All all lines needed for visualizing the box
cee::PtrRef<cee::vis::MarkupPartLines> part = new cee::vis::MarkupPartLines(cee::Color3f(1.0, 1.0, 0.0), 3, true, 0.0);
part->add(p1, p2);
part->add(p2, p3);
part->add(p3, p4);
part->add(p4, p1);
part->add(p1, p5);
part->add(p2, p6);
part->add(p3, p7);
part->add(p4, p8);
part->add(p5, p6);
part->add(p6, p7);
part->add(p7, p8);
part->add(p8, p5);
markupModel->addPart(part.get());
// Add the box to the view
gcView->addModel(markupModel.get());
gcView->camera().fitView(bb, cee::Vec3d(0, 0, -1), cee::Vec3d(0, 1, 0));
gcView->camera().inputHandler()->setRotationPoint(bb.center());
gcView->requestRedraw();
}