UnstructGrid: Create a Cutting Plane with a Scalar Result as Fringes
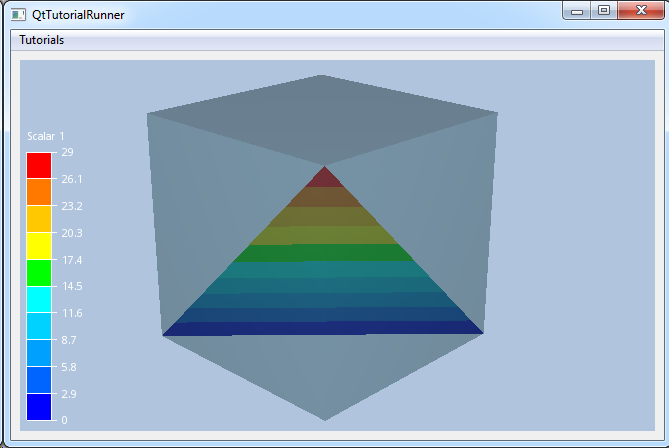
Cutting planes are slices through the structure onto which scalar and vector fields can be visualized. By dynamically changing the position and orientation of the cutting plane, you can quickly analyze the results inside the system.
Each cutting plane can have its own settings and be moved individually.
This tutorial shows how to create a cutting plane and show a scalar result mapped as fringes to the surface.
The demo file (30x30x30.vtf) contains a single part geometry with a synthetic scalar result.
Load the file and setup the model specification to show the result. For a more thorough explanation on creating a data source from a file interface and setup the model specification, see the Load VFT tutorial:
UnstructGrid: Load Model from File and Set Up Model Specification
Note
This tutorial expect the application to have a correctly configured cee::vis::View
in place. See the demo applications on how to set up a cee::vis::View
in your application.
Load model
Create the model and a VTF file interface data source. Open the file and set the created data source in the model.
cee::PtrRef<cee::ug::UnstructGridModel> ugModel = new cee::ug::UnstructGridModel();
cee::PtrRef<cee::ug::DataSourceVTF> source = new cee::ug::DataSourceVTF(42);
cee::Str vtfFile = TutorialUtils::testDataDir() + "30x30x30.vtf";
if (!source->open(vtfFile))
{
// VTF file not found
return;
}
ugModel->setDataSource(source.get());
Set the first state as current in the model specification.
std::vector<cee::ug::StateInfo> stateInfos = source->directory()->stateInfos();
int stateId = stateInfos[0].id();
ugModel->modelSpec().setStateId(stateId);
Find the id of the first scalar result from the data source’s directory.
std::vector<cee::ug::ResultInfo> scalarResultInfos = source->directory()->scalarResultInfos();
int scalarId = scalarResultInfos[0].id();
Create cutting plane
Create the cutting plane object. Remember that the object is reference counted and should not be created on the stack.
The cutting plane is defined by a point in the plane and the normal to the plane.
cee::PtrRef<cee::ug::CuttingPlane> cuttingPlane = new cee::ug::CuttingPlane();
cuttingPlane->setPoint(cee::Vec3d(10, 10, 10));
cuttingPlane->setNormal(cee::Vec3d(1, 1, 1));
Set the scalar result to be shown as mapped fringes on the cutting plane surface.
cuttingPlane->setMapScalarResultId(scalarId);
Add the cutting plane to the model.
ugModel->addCuttingPlane(cuttingPlane.get());
To get a better view of the cutting plane inside the model, set all parts to transparent.
cee::ug::PartSettingsIterator it(ugModel.get());
while (it.hasNext())
{
cee::ug::PartSettings* partSettings = it.next();
partSettings->setOpacity(0.5f);
}
Set up the created model
The model is ready to use and can be added to the view. Exactly where the view exists
depends on the platform and solution. These examples uses Qt and the view is set up
in a cee::qt::ViewerWidget
.
cee::vis::View* gcView = getTutorialView();
gcView->addModel(ugModel.get());
ugModel->updateVisualization();
See the complete source code here:
UnstructGrid: Create a Cutting Plane with a Scalar Result as Fringes