UnstructGrid: Apply Part Settings to a Model
Each part has part settings that can be configured to make a better visual appearance of your data, for instance settings such as visibility, draw style, coloring, highlighting and result visibility.
The demo file (contact.vtfx) contains a geometry with four parts. This example shows how to set the following settings on these parts:
Part 1: Set invisible
Part 2: Set color to red
Part 3: Set draw style surface mesh
Part 4: Set transparent
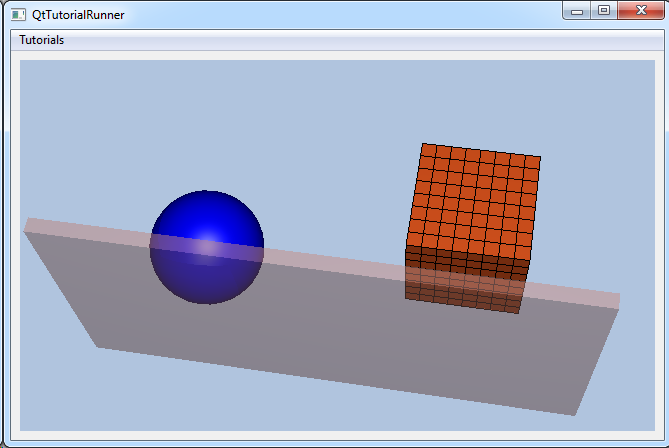
void TutorialRunnerMainWindow::setPartSettings()
{
//--------------------------------------------------------------------------
// Load an UnstructGridModel from file
// Apply some part settings
//--------------------------------------------------------------------------
// Create the model and data source
cee::PtrRef<cee::ug::UnstructGridModel> ugModel = new cee::ug::UnstructGridModel();
cee::PtrRef<cee::ug::DataSourceVTFx> source = new cee::ug::DataSourceVTFx(42);
// Open the VTFx file
cee::Str vtfxFile = TutorialUtils::testDataDir() + "contact.vtfx";
if (!source->open(vtfxFile, 0))
{
// VTFx file not found
return;
}
// Add the data source to the model
ugModel->setDataSource(source.get());
// Set the first state as current
std::vector<cee::ug::StateInfo> stateInfos = source->directory()->stateInfos();
int stateId = stateInfos[0].id();
ugModel->modelSpec().setStateId(stateId);
// This model contains 4 parts
// Part 1: Set invisible
// Part 2: Set color to blue
// Part 3: Set draw style surface mesh
// Part 4: Set transparent
// Get the id for all the parts through the meta data directory
// Get the corresponding part settings
// Set the part setting as listed above
std::vector<cee::ug::PartInfo> partInfos = source->directory()->partInfos(0);
int firstPartId = partInfos[0].id();
int secondPartId = partInfos[1].id();
int thirdPartId = partInfos[2].id();
int fourthPartId = partInfos[3].id();
// Part 1: Set invisible
ugModel->partSettings(0, firstPartId)->setVisible(false);
// Part 2: Set color to blue
ugModel->partSettings(0, secondPartId)->setColor(cee::Color3f(0.0, 0.0, 1.0));
// Part 3: Set draw style surface mesh
ugModel->partSettings(0, thirdPartId)->setDrawStyle(cee::ug::PartSettings::SURFACE_MESH);
// Part 4: Set transparent
ugModel->partSettings(0, fourthPartId)->setOpacity(0.5f);
// Add model to view. Ensure that old models are removed first
cee::vis::View* gcView = getTutorialView();
gcView->removeAllModels();
gcView->addModel(ugModel.get());
ugModel->updateVisualization();
cee::BoundingBox bb = gcView->boundingBox();
gcView->camera().fitView(bb, cee::Vec3d(0, 0, -1), cee::Vec3d(0, 1, 0));
gcView->camera().inputHandler()->setRotationPoint(bb.center());
gcView->requestRedraw();
}