Minimal VTFx File
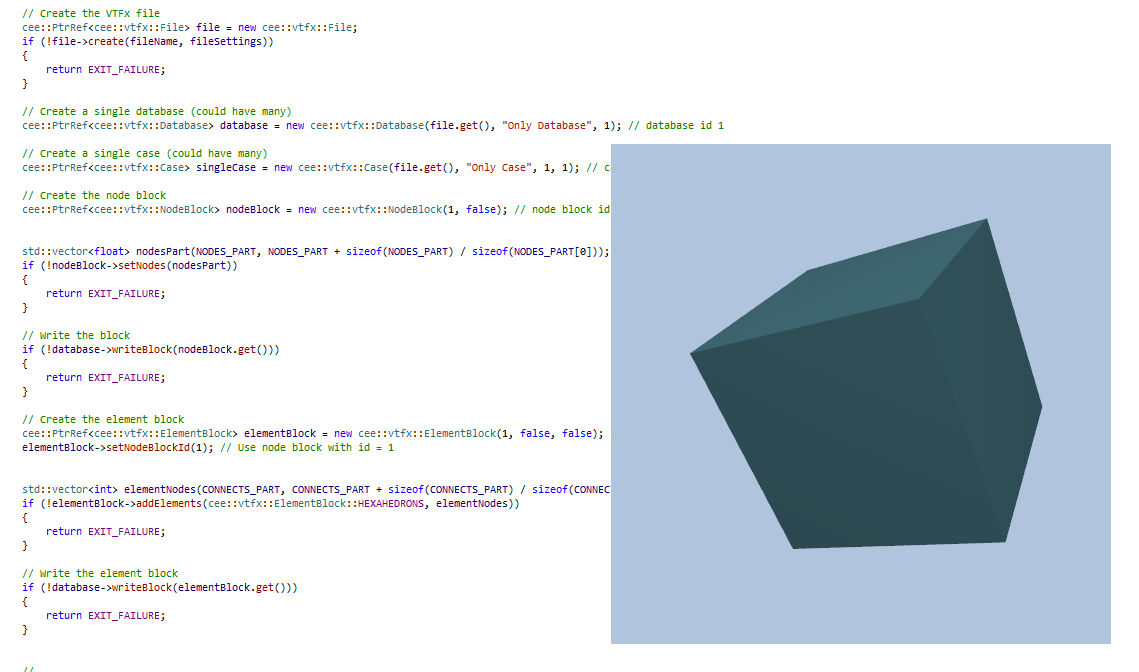
This is the most basic example of a VTFx.
The minimum requirements for a VTFx file are:
one database with
one node block
one element block, referencing the node block
one geometry block, referencing the element block
one state info block
one case with a reference to the database
This example shows how to create such a minimal VTFx file with a simple part consisting of just one hexahedron element. The VTFx file has only one state, no results of any kind nor any properties.
//--------------------------------------------------------------------------
// Create the VTFx file
// -------------------------------------------------------------------------
cee::PtrRef<cee::vtfx::File> file = new cee::vtfx::File;
// Create VTFx file settings struct
cee::vtfx::FileSettings fileSettings;
fileSettings.applicationName = "VTFx: VTFxMinimal";
fileSettings.vendorName = "My Company AS";
// Let the API create the VTFx file
const cee::Str fileName = "ExampleMinimal.vtfx";
if (!file->create(fileName, fileSettings))
{
return EXIT_FAILURE;
}
// Create a single database (could have many)
cee::PtrRef<cee::vtfx::Database> database = new cee::vtfx::Database(file.get(), "Only Database", 1, cee::vtfx::Database::SIMULATION_TYPE_STRUCTURAL, cee::vtfx::Database::SOLUTION_TYPE_TRANSIENT, "Database description"); // database id 1
// Create a single case (could have many)
cee::PtrRef<cee::vtfx::Case> singleCase = new cee::vtfx::Case(file.get(), "Only Case", 1, 1); // case id 1 using database with id 1
//--------------------------------------------------------------------------
// Add nodes
// -------------------------------------------------------------------------
// Create the node block
cee::PtrRef<cee::vtfx::NodeBlock> nodeBlock = new cee::vtfx::NodeBlock(1, false); // node block id 1, not using node ids
// Set the node data (Note: The VTFx component expects interleaved node coordinates (xyzxyzxyz...))
const float NODES_PART[] =
{
0.0f, 0.0f, 0.0f,
1.0f, 0.0f, 0.0f,
1.0f, 1.0f, 0.0f,
0.0f, 1.0f, 0.0f,
0.0f, 0.0f, 1.0f,
1.0f, 0.0f, 1.0f,
1.0f, 1.0f, 1.0f,
0.0f, 1.0f, 1.0f
};
std::vector<float> nodesPart(NODES_PART, NODES_PART + sizeof(NODES_PART) / sizeof(NODES_PART[0]));
if (!nodeBlock->setNodes(nodesPart))
{
return EXIT_FAILURE;
}
// Write the block
if (!database->writeBlock(nodeBlock.get()))
{
return EXIT_FAILURE;
}
//--------------------------------------------------------------------------
// Add elements
// -------------------------------------------------------------------------
// Create the element block
cee::PtrRef<cee::vtfx::ElementBlock> elementBlock = new cee::vtfx::ElementBlock(1, false, false); // element block id 1, not using element ids, referring nodes by index
elementBlock->setNodeBlockId(1); // Use node block with id = 1
// Set the element data
const int CONNECTS_PART[] =
{
0, 1, 2, 3, 4, 5, 6, 7
};
std::vector<int> elementNodes(CONNECTS_PART, CONNECTS_PART + sizeof(CONNECTS_PART) / sizeof(CONNECTS_PART[0]));
if (!elementBlock->addElements(cee::vtfx::ElementBlock::HEXAHEDRONS, elementNodes))
{
return EXIT_FAILURE;
}
// Write the element block
if (!database->writeBlock(elementBlock.get()))
{
return EXIT_FAILURE;
}
//--------------------------------------------------------------------------
// Add geometry
// -------------------------------------------------------------------------
// Create geometry block
cee::PtrRef<cee::vtfx::GeometryBlock> geoBlock = new cee::vtfx::GeometryBlock(1); // Only one geometry per state
size_t geoIndex = 0;
int partId = 1;
// Set to use element block with id 1
if (!geoBlock->addElementBlock(geoIndex, 1, partId)) // Element block with id = 1
{
return EXIT_FAILURE;
}
// Write the geometry block
if (!database->writeBlock(geoBlock.get()))
{
return EXIT_FAILURE;
}
// Create geometry info block
cee::PtrRef<cee::vtfx::GeometryInfoBlock> infoBlock = new cee::vtfx::GeometryInfoBlock(1); // Only one geometry per state
infoBlock->addPartInfo(geoIndex, partId, cee::Str("Only part"));
// Write the geometry info block
if (!database->writeBlock(infoBlock.get()))
{
return EXIT_FAILURE;
}
//--------------------------------------------------------------------------
// Add one state
// -------------------------------------------------------------------------
// Create state info block
cee::PtrRef<cee::vtfx::StateInfoBlock> stepInfo = new cee::vtfx::StateInfoBlock;
if (!stepInfo->addStateInfo(1, "Only step", 42.0f, cee::vtfx::StateInfoBlock::TIME))
{
return EXIT_FAILURE;
}
// Write the state info block
if (!database->writeBlock(stepInfo.get()))
{
return EXIT_FAILURE;
}
// Finally close the file
if (!file->close())
{
return EXIT_FAILURE;
}
std::cout << "Exported successfully to file: " << fileName.toStdString() << std::endl;
std::cout << std::endl << "Press enter to exit..." << std::endl;
std::cin.ignore();
return EXIT_SUCCESS;