UnstructGrid: Set Scalar Settings on a Loaded Model
The scalar result can be configured to make a better visual appearance of your result data, for instance settings such as legend scheme, legend range and filtering.
This example will show the scalar as fringes on the model, and set some scalar settings for demonstration.
Set number of legend levels to 5
Set color scheme to “Normal Inverted”.
Filter away all values under 20.
The demo model file (100x100x100.vtf) contains a simple geometry and a scalar result.
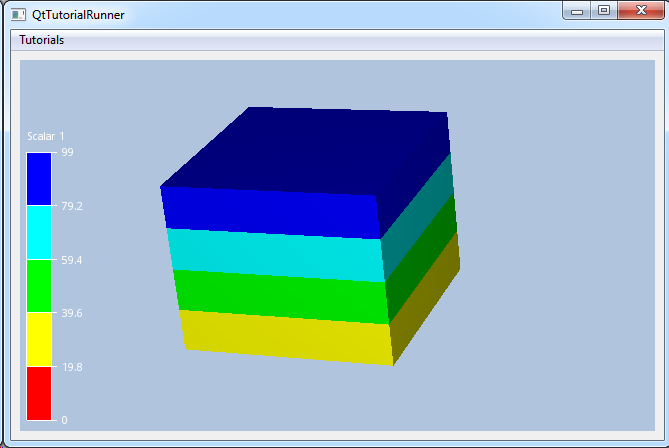
void TutorialRunnerMainWindow::setScalarSettings()
{
//--------------------------------------------------------------------------
// Load an UnstructGridModel from file and set a scalar result
// Apply some scalar settings
//--------------------------------------------------------------------------
// Create the model and data source
cee::PtrRef<cee::ug::UnstructGridModel> ugModel = new cee::ug::UnstructGridModel();
cee::PtrRef<cee::ug::DataSourceVTF> source = new cee::ug::DataSourceVTF(42);
// Open the vtf file
cee::Str vtfFile = TutorialUtils::testDataDir() + "100x100x100.vtf";
if (!source->open(vtfFile))
{
// VTF file not found
return;
}
// Add the data source to the model
ugModel->setDataSource(source.get());
// Set the first state as current
std::vector<cee::ug::StateInfo> stateInfos = source->directory()->stateInfos();
int stateId = stateInfos[0].id();
ugModel->modelSpec().setStateId(stateId);
// Show the scalar result as fringes on the model
std::vector<cee::ug::ResultInfo> scalarResultInfos = source->directory()->scalarResultInfos();
int scalarId = scalarResultInfos[0].id();
ugModel->modelSpec().setFringesResultId(scalarId);
// Set fringes visible for all parts
cee::ug::PartSettingsIterator it(ugModel.get());
while (it.hasNext())
{
cee::ug::PartSettings* partSettings = it.next();
partSettings->setFringesVisible(true);
}
// Get scalar settings for the current fringes result
cee::ug::ScalarSettings* settings = ugModel->scalarSettings(scalarId);
// Set number of legend levels to 0 to get a continuous legend
settings->colorMapper().setTypeFilledContoursUniform(5);
// Set the color scheme to the reverted normal
settings->colorMapper().setColorScheme(cee::ug::ColorMapper::NORMAL_INVERTED);
// Use filter to only show values above 20. Toggle on filtering.
settings->setFringesElementFilteringVisibleRange(20, 99);
// Add model to view. Ensure that old models are removed first
cee::vis::View* gcView = getTutorialView();
gcView->removeAllModels();
gcView->addModel(ugModel.get());
ugModel->updateVisualization();
cee::BoundingBox bb = gcView->boundingBox();
gcView->camera().fitView(bb, cee::Vec3d(0, 0, -1), cee::Vec3d(0, 1, 0));
gcView->camera().inputHandler()->setRotationPoint(bb.center());
gcView->requestRedraw();
}