UnstructGrid: Create an Isovolume
An isovolume is defined as the combined volume of the element model where the scalar field is between a given minimum and maximum value. The surface of the isovolume will be the hull of this volume. Several visual settings can be applied to the isovolume, such as mapped fringes, visibility and highlighting.
This example shows how to create an isovolume based on a scalar result id and a minimum and a maximum scalar value. In addition, the scalar result will also be shown as fringes on the isovolume.
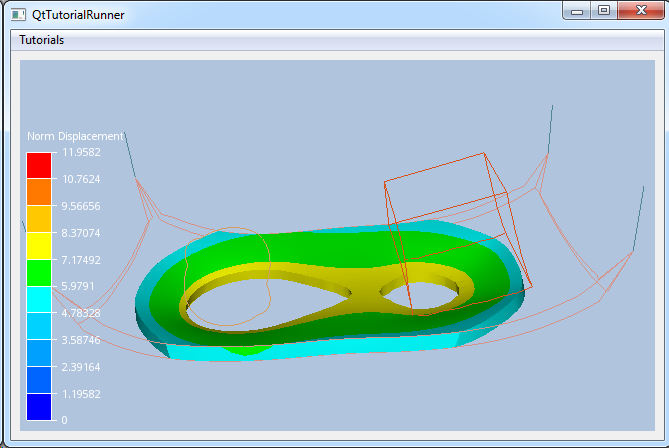
void TutorialRunnerMainWindow::addIsovolume()
{
//--------------------------------------------------------------------------
// Load an UnstructGridModel from file
// Create an isovolume
//--------------------------------------------------------------------------
// Create the model and data source
cee::PtrRef<cee::ug::UnstructGridModel> ugModel = new cee::ug::UnstructGridModel();
cee::PtrRef<cee::ug::DataSourceVTFx> source = new cee::ug::DataSourceVTFx(42);
// Open the VTFx file
cee::Str vtfxFile = TutorialUtils::testDataDir() + "contact.vtfx";
if (!source->open(vtfxFile, 0))
{
// VTFx file not found
return;
}
// Add the data source to the model
ugModel->setDataSource(source.get());
// Set the fourth state as current.
std::vector<cee::ug::StateInfo> stateInfos = source->directory()->stateInfos();
int stateId = stateInfos[3].id();
ugModel->modelSpec().setStateId(stateId);
// Create a new isovolume object and set the scalar result id and iso scalar value used to calculate the isovolume topography.
cee::PtrRef<cee::ug::Isovolume> isoVolume = new cee::ug::Isovolume();
// User "displacements" scalar result
std::vector<cee::ug::ResultInfo> resultInfos = source->directory()->scalarResultInfos();
int scalarId = resultInfos[0].id();
isoVolume->setIsoScalarResultId(scalarId);
isoVolume->setMinimumIsoValue(5.5);
isoVolume->setMaximumIsoValue(7.5);
// Set the same result mapped on the volume also
isoVolume->setMapScalarResultId(scalarId);
// Add the isovolume to the model
ugModel->addIsovolume(isoVolume.get());
// To be able to see the isovolume inside the part, set draw styles to LINES for all parts
cee::ug::PartSettingsIterator it(ugModel.get());
while (it.hasNext())
{
cee::ug::PartSettings* partSettings = it.next();
partSettings->setDrawStyle(cee::ug::PartSettings::OUTLINE);
}
// Add model to view. Ensure that old models are removed first
cee::vis::View* gcView = getTutorialView();
gcView->removeAllModels();
gcView->addModel(ugModel.get());
ugModel->updateVisualization();
cee::BoundingBox bb = gcView->boundingBox();
gcView->camera().fitView(bb, cee::Vec3d(0, 0, -1), cee::Vec3d(0, 1, 0));
gcView->camera().inputHandler()->setRotationPoint(bb.center());
gcView->requestRedraw();
}