Plot2d: Create a Simple Overlay Plot
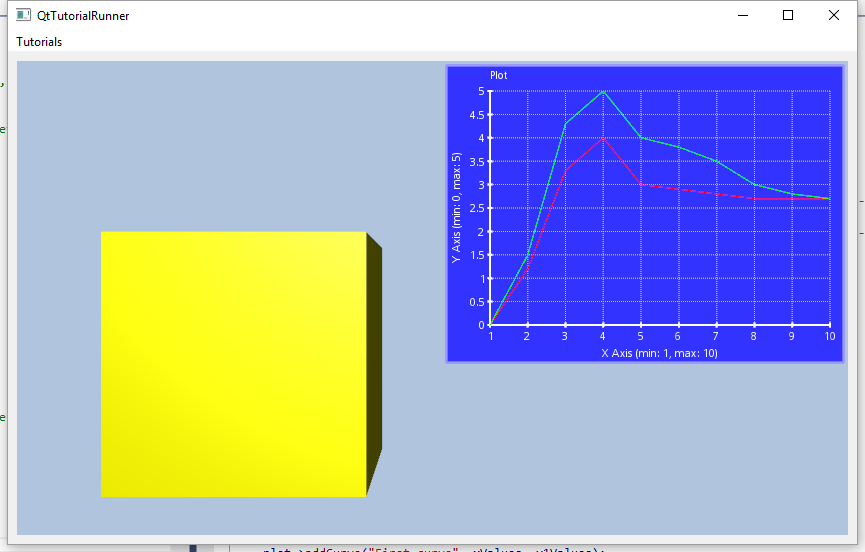
The Plot2d component enables the user to do basic 2D plotting as an overlay item in the view.
This tutorial creates a simple overlay plot to the view and adds two curves.
Note
This tutorial expect the application to have a correctly configured cee::vis::View
in place. See demo applications on how to set up a cee::vis::View
in your application.
Create plot data
Create the value arrays for the two curves. In this case, the x-values are the same for both curves and are reused.
// X Values (same for both curves)
std::vector<double> xValues = { 1.0, 2.0, 3.0, 4.0, 5.0, 6.0, 7.0, 8.0, 9.0, 10.0 };
// Y Values
std::vector<double> y1Values = { 0.0, 1.2, 3.3, 4.0, 3.0, 2.9, 2.8, 2.7, 2.7, 2.7 };
std::vector<double> y2Values = { 0.0, 1.5, 4.3, 5.0, 4.0, 3.8, 3.5, 3.0, 2.8, 2.7 };
Create plot
Create the plot as an overlay item and add both curves.
cee::PtrRef<cee::vis::Font> font = cee::vis::Font::createNormalFont();
cee::PtrRef<cee::plt::OverlayPlot> plot = new cee::plt::OverlayPlot(font.get());
plot->setSize(400, 300);
plot->addCurve("First curve", xValues, y1Values);
plot->addCurve("Second curve", xValues, y2Values);
Add to view
Add the overlay plot item to the view.
cee::vis::View* gcView = getTutorialView();
gcView->overlay().addItem(plot.get(), cee::vis::OverlayItem::TOP_RIGHT, cee::vis::OverlayItem::HORIZONTAL);
gcView->requestRedraw();
See the complete source code here: