Geometry: Highlight Clicked Parts
A highlighted part is shown with a white halo. This is useful for indicating for instance selection. The highlighting mechanism applies to all types of parts:
Data parts
Isosurfaces
Isovolumes
Cutting planes
Geometry parts (using halo effect)
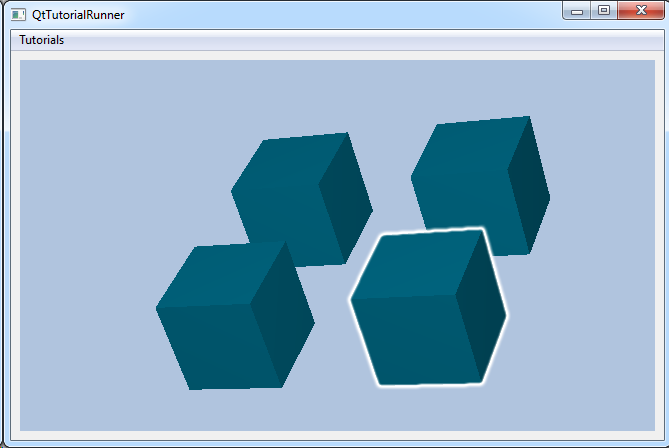
This example shows how highlight parts as a response to a mouse press event.
Pick event
Send a notification when a pick has occurred.
Attach to the mouse pressed event in the viewer. Create a cee::Ray
object from the mouse
event coordinates and emit a picked signal using this ray.
void TutorialRunnerViewer::mousePressEvent(QMouseEvent* event)
{
cee::vis::View* theView = view();
if (!theView) return;
// Picking!
cee::Ray ray = theView->camera().rayFromWindowCoordinates(event->pos().x(), height() - event->pos().y());
m_mainWindow->pick(ray);
cee::vis::CameraInputHandler* inputHandler = theView->camera().inputHandler();
if (inputHandler)
{
cee::vis::MouseEvent me = cee::qt::UtilsGui::toMouseEvent(*this, *event);
cee::vis::MouseButton buttonPressed = cee::qt::UtilsGui::toMouseButton(event->button());
inputHandler->mousePressEvent(buttonPressed, me);
update();
}
}
Create the model and connection
Create a geometry model with four boxes.
void TutorialRunnerMainWindow::highlighting()
{
cee::vis::View* gcView = getTutorialView();
cee::PtrRef<cee::geo::GeometryModel> geometryModel = new cee::geo::GeometryModel;
gcView->setModelOpenGLCapabilitiesFromView(geometryModel.get());
// Add four boxes
geometryModel->addPart(new cee::geo::Part(cee::geo::DataGenerator::createBox(cee::Vec3d(1.0, 1.0, 1.0), cee::Vec3d(1.0, 1.0, 1.0)).get()));
geometryModel->addPart(new cee::geo::Part(cee::geo::DataGenerator::createBox(cee::Vec3d(3.0, 1.0, 1.0), cee::Vec3d(1.0, 1.0, 1.0)).get()));
geometryModel->addPart(new cee::geo::Part(cee::geo::DataGenerator::createBox(cee::Vec3d(1.0, 3.0, 1.0), cee::Vec3d(1.0, 1.0, 1.0)).get()));
geometryModel->addPart(new cee::geo::Part(cee::geo::DataGenerator::createBox(cee::Vec3d(3.0, 3.0, 1.0), cee::Vec3d(1.0, 1.0, 1.0)).get()));
// Add model to view. Ensure that old models are removed first
gcView->removeAllModels();
gcView->addModel(geometryModel.get());
cee::BoundingBox bb = gcView->boundingBox();
gcView->camera().fitView(bb, cee::Vec3d(0, 0, -1), cee::Vec3d(0, 1, 0));
gcView->camera().inputHandler()->setRotationPoint(bb.center());
gcView->requestRedraw();
}
Respond to a picking event sent from the viewer
Highlight the last hit box
void TutorialRunnerMainWindow::pick(const cee::Ray& ray)
{
cee::vis::View* gcView = getTutorialView();
// Get the current model as a geometry model
cee::geo::GeometryModel* geometryModel = dynamic_cast<cee::geo::GeometryModel*>(gcView->model(0));
if (!geometryModel)
{
return;
}
// Do the picking
cee::geo::HitItem hitItem;
if (!geometryModel->rayIntersect(ray, *gcView, &hitItem))
{
// No parts were hit, return without doing any changes
return;
}
// Loop all parts. Toggle off highlighting for all parts except the currently hit
for (size_t i = 0; i < geometryModel->partCount(); i++)
{
cee::geo::Part* part = geometryModel->part(i);
part->settings().removeAllEffectsOfType<cee::geo::EffectHalo>();
if (part == hitItem.part())
{
part->settings().addEffect(new cee::geo::EffectHalo);
}
}
gcView->requestRedraw();
}