6. Drawing Functions - DrawFun
-
Begin and end an instance of an object, generic object functions
*vgl_DrawFunBegin - create an instance of a DrawFun object vgl_DrawFunEnd - destroy an instance of a DrawFun object vgl_DrawFunError - return DrawFun object error flag vgl_DrawFunCopy - make a copy of a DrawFun object
-
Set function pointers
vgl_DrawFunSet - set function pointers Get - get function pointers vgl_DrawFunSetObj - set auxiliary object GetObj - get auxiliary object vgl_DrawFunAPI - set built-in drawing functions
-
Window operations
vgl_DrawFunCloseWindow - close window vgl_DrawFunConfigureWindow - request window position, size, state vgl_DrawFunConnectWindow - connect to preexisting window vgl_DrawFunDisconnectWindow - disconnect from window vgl_DrawFunOpenWindow - open window vgl_DrawFunParentWindow - specify parent window vgl_DrawFunPositionWindow - request initial window position and size vgl_DrawFunQueryWindow - query current window vgl_DrawFunSetWindow - set currently active window for drawing vgl_DrawFunVisualWindow - request window visual properties
-
Frame buffer control
vgl_DrawFunBackColor - set current clear RGB color vgl_DrawFunBackColorIndex - set current clear color index vgl_DrawFunBell - ring bell vgl_DrawFunClear - clear frame buffer vgl_DrawFunDelay - time delay vgl_DrawFunFlush - flush all pending graphics primitives vgl_DrawFunRender - set rasterization mode vgl_DrawFunResize - resize frame buffer vgl_DrawFunSetFactors - set factors to control shader modes vgl_DrawFunSetMode - set current graphics mode GetMode - return current graphics modes vgl_DrawFunSetSwitch - enable, disable lights, clipping planes vgl_DrawFunSwap - swap frame buffers
-
Query, Selection, Extent, Buffer
vgl_DrawFunBufferSize - specify color buffer size vgl_DrawFunExtentQuery - query extent box vgl_DrawFunGetFloat - return selected floating point parameters vgl_DrawFunGetInteger - return selected integer parameters vgl_DrawFunGetString - return selected string parameters vgl_DrawFunSelectBuffer - specify selection hit buffers vgl_DrawFunSelectQuery - query number of selection hits vgl_DrawFunSelectRegion - specify selection region
-
Projection and viewport transformations
vgl_DrawFunClip - define clipping rectangle vgl_DrawFunClipPlane - define clipping plane vgl_DrawFunDepthRange - define z buffer mapping vgl_DrawFunProjFrustum - define a perspective projection vgl_DrawFunProjOrtho - define an orthographic projection vgl_DrawFunProjPop - pop projection matrix from stack vgl_DrawFunProjPush - push projection matrix onto stack vgl_DrawFunProjLoad - load projection matrix onto stack vgl_DrawFunViewport - define viewport transformation
-
Modelling and viewing transformations
vgl_DrawFunXfmLoad - load modelview matrix onto stack vgl_DrawFunXfmMult - multiply modelview matrix vgl_DrawFunXfmPop - pop modelview matrix from stack vgl_DrawFunXfmPush - push modelview matrix on stack
-
Lighting
vgl_DrawFunLight - define a light source vgl_DrawFunLightModel - define overall lighting model properties
-
Raster Fonts, Bitmaps and Texture Maps
vgl_DrawFunBitmapDefine - define a bitmap vgl_DrawFunBitmapSelect - select current bitmap vgl_DrawFunRasFontDefine - define a raster font vgl_DrawFunRasFontSelect - select current raster font vgl_DrawFunTextureDefine - define a texture map vgl_DrawFunTextureSelect - select current texture map
-
Graphics attributes
vgl_DrawFunAttPop - pop attributes from stack vgl_DrawFunAttPush - push attributes onto stack vgl_DrawFunColor - set current RGB color vgl_DrawFunColorIndex - set current color index vgl_DrawFunData - set current data values vgl_DrawFunDataIndex - load index values vgl_DrawFunLineStyle - set line style vgl_DrawFunLineWidth - set line width vgl_DrawFunPointSize - set point size vgl_DrawFunPointStyle - set point style vgl_DrawFunPolygonMode - specify polygon drawing mode vgl_DrawFunPolygonOffset - specify polygon factor and bias vgl_DrawFunSpecularity - set specularity vgl_DrawFunTextPixelSize - specify raster font pixel size vgl_DrawFunTextPlane - specify raster font plane vgl_DrawFunTrans - set transparency factor vgl_DrawFunTransIndex - set transparency index
-
Graphics vertex buffers
vgl_DrawFunInitBuffer - create a vertex buffer vgl_DrawFunTermBuffer - delete a vertex buffer vgl_DrawFunCopyBuffer - copy vertex data to a vertex buffer
-
Graphics primitives
vgl_DrawFunPolygon - draw polygon vgl_DrawFunPolygonArray - draw arrays of polygons vgl_DrawFunPolygonBuffer - draw vertex buffers of polygons vgl_DrawFunPolygonColor - draw Gouraud shaded polygon vgl_DrawFunPolygonData - draw polygon with data vgl_DrawFunPolygonDC - draw device oriented polygon vgl_DrawFunPolyLine - draw polyline vgl_DrawFunPolyLineArray - draw arrays of polylines vgl_DrawFunPolyLineBuffer - draw vertex buffers of polylines vgl_DrawFunPolyLineColor - draw Gouraud shaded polyline vgl_DrawFunPolyLineData - draw polyline with data vgl_DrawFunPolyLineDC - draw device oriented polyline vgl_DrawFunPolyPoint - draw points vgl_DrawFunPolyPointArray - draw arrays of points vgl_DrawFunPolyPointBuffer - draw vertex buffers of points vgl_DrawFunPolyPointColor - draw colored points vgl_DrawFunPolyPointData - draw points with data vgl_DrawFunPolyPointDC - draw device oriented points vgl_DrawFunPolyArray - draw arrays of primitives vgl_DrawFunPolyBuffer - draw vertex buffers of primitives vgl_DrawFunPolyElemArray - draw indexed arrays of primitives vgl_DrawFunPolyElemBuffer - draw indexed vertex buffers of primitives vgl_DrawFunText - draw raster font text vgl_DrawFunTextDC - draw screen offset raster font text
-
Pixel level operations
vgl_DrawFunFBufferRead - read frame buffer to client side vgl_DrawFunFBufferWrite - write frame buffer from client side vgl_DrawFunZBufferRead - read z buffer to client side vgl_DrawFunZBufferWrite - write z buffer from client side vgl_DrawFunPixelmapCreate - create server side pixmap vgl_DrawFunPixelmapDestroy - destroy server side pixmap vgl_DrawFunPixelmapRead - read into server side pixmap vgl_DrawFunPixelmapWrite - write from server side pixmap
-
Graphics input
vgl_DrawFunPollMouse - poll mouse location and buttons vgl_DrawFunPollModifiers - poll control and shift buttons vgl_DrawFunWarpMouse - move mouse location vgl_DrawFunSetCursor - set cursor style vgl_DrawFunReadQueue - read from event queue vgl_DrawFunTestQueue - test event queue vgl_DrawFunResetQueue - reset event queue
6.1 Window Operations
VglTools provides a number of functions for setting window attributes and opening and closing windows. Two basic options are available to the user. Either VglTools functions can be used to open and close the graphics window or the user can open and close and otherwise manage the graphics window himself. In the latter case VglTools must be passed the appropriate window id or handle in order to render to the window. In either case it is the responsibility of the user to perform global window management operations which are window system dependent. In the case of X windows, the user must open the display and select a screen. The following code fragment illustrates this process,#include <X11/Xlib.h> Display *display; int screen; /* open X display */ display = XOpenDisplay (0); screen = DefaultScreen (display);
Once the display and screen are established, each graphics device interface module must be passed this information. The display and screen are stored as "class" variables within each module. All subsequent objects instanced from a particular module will use the current display and screen class variables of the module. For example, to set the display and screen information in the OpenGL device interface module, OpenGLDev, use the following:
vgl_OpenGLDevConnectX (display,screen);Before rendering can occur to a graphics device, a device object and associated drawing function object must be instanced, window attributes set and a window opened. The following illustrates instancing OpenGLDev and DrawFun objects and setting OpenGL drawing functions.
vgl_OpenGLDev *ogldev; vgl_DrawFun *df; /* create drawing function */ df = vgl_DrawFunBegin(); /* create OpenGL device object */ ogldev = vgl_OpenGLDevBegin(); /* load drawing functions for OpenGL device */ vgl_OpenGLDevDrawFun (ogldev,df);At this point opening, closing and rendering to a graphics window can be accomplished abstractly using a DrawFun object generated in the manner above.
If the user wishes to open and close the graphics window, vgl_DrawFunConnectWindow must be used to inform the device interface object of the window id or handle of the window to render to. If the user opens the window using VglTools, set any window attributes such as parent, size, position, or visual class using vgl_DrawFunParentWindow, vgl_DrawFunConfigureWindow, vgl_DrawFunPositionWindow, and vgl_DrawFunVisualWindow. Then open the window using vgl_DrawFunOpenWindow. At this point the window is ready for rendering. To close a window and terminate VglTools, reverse the process described above. The following code fragment illustrates this process:
/* close window */ vgl_DrawFunCloseWindow (df); /* delete objects */ vgl_OpenGLDevEnd (ogldev); vgl_DrawFunEnd (df); /* disconnect from X server */ vgl_OpenGLDevDisconnect (); /* close X display */ XCloseDisplay (display);
6.2 Frame Buffer Control
Frame buffer control consists of a number of functions to initiate explicit frame buffer actions such as clearing to a background color and a single function to enable and disable certain frame buffer modes. VglTools is designed to support single and double buffered true color frame buffers, a z buffer for performing hidden surface removal and a stencil buffer. If the underlying graphics hardware does not provide a double buffered frame buffer and/or z buffer, VglTools attempts to implement the capability using off screen pixmaps, and software implementations of the z-buffer and stencil buffer. Stencil buffer control is conceptually identical to OpenGL. The stencil buffer depth is typically 8 bits.Frame buffer modes are enabled and disabled using vgl_DrawFunSetMode. Examples of frame buffer modes include z-buffering, XOR drawing, double buffering, front buffer drawing and reading and stencil buffer control. One particularly unique frame buffer mode is polygon depth mode. When enabled, polygon depth mode ensures that when points, lines and polygons are drawn coincidently in space that polygons are automatically given a slightly greater depth than points and lines. This results in points and lines being given visibility priority over polygons. This is useful when drawing the edges of polygons or contour lines across a polygon.
Frame buffer actions include setting the background or "clear" color, swapping buffers in a double buffered frame buffer, resizing the frame buffer, etc. The frame buffer must be resized with vgl_DrawFunResize whenever the dimensions of the graphics window change.
6.3 Query
VglTools allows the current state of any graphics device interface object to be queried. Frame buffer configuration, graphics primitive attributes, current transformation matrices may all be returned. Three functions are provided for this purpose. All frame buffer modes set with vgl_DrawFunSetMode may be retrieved using vgl_DrawFunGetMode. All other graphics state variables are returned using either vgl_DrawFunGetInteger, vgl_DrawFunGetFloat or vgl_DrawFunGetString depending upon the data type of the queried state variables.6.4 Transformations
The coordinate transformation capabilities of VglTools is patterned after the transformation model used by OpenGL. Modelling and viewing transformations as well as viewport transformations and manipulation of the matrix stack are conceptually identical to OpenGL.6.5 Lighting
VglTools device interface modules can automatically calculate the color of polygon primitives using standard lighting models. The color of point, line and text primitives is not affected by lights.Up to 8 lights may defined to light a scene. Ambient light is generally provided by a single ambient light, it produces no diffuse or specular reflections. Diffuse and specular reflections are generated by defining one or more distant lights. Lights which produce diffuse and specular reflections do not contribute to the ambient light. Diffuse and specular reflections are only produced by polygon primitives for which the polygon normals have been supplied by the user. VglTools does not compute polygon normals automatically.
The current color is assumed to be the base material color for ambient and diffuse lighting effects. Specular reflections are only produced if vgl_DrawFunSpecularity has been called to specify a current material specularity and shininess.
6.6 Graphics Attributes and Primitives
VglTools is designed to draw point, line, polygon and raster text primitives. The geometric form and color can be altered by a number of standard graphics attributes such as point size, line width, line style and polygon transparency. All graphics primitives are 3D in that all input vertex coordinates and normals require 3 components. Color is input as a 3-component RGB or 4-component RGBA value. Texture coordinates may be either 1-component or 2-component.All point, line and polygon primitives come in 6 varieties. The basic function draws a graphics primitive with a constant color with all vertex coordinates expressed in world coordinates, eg. vgl_DrawFunPolyLine. A graphics primitive may be drawn with varying color, called "Gouraud shading", by adding an argument specifying a color for each vertex, eg. vgl_DrawFunPolyLineColor. A special "data" rendering function is provided for those rendering methods which render raw data to a frame buffer, eg. vgl_DrawFunPolyLineData. A fourth function type draws a graphics primitive anchored at a single 3D world coordinate location with all other vertices specified as device coordinate (pixel) offsets from the anchor point, eg. vgl_DrawFunPolyLineDC. This allows primitives to be drawn which are always oriented perpendicular to the view direction and are unaffected by scaling transformations.
The final two function types for drawing graphics primitives are designed to draw large arrays of primitives with varying vertex colors, normals, and texture coordinates. Point, line and polygon graphics primitives can be drawn with full access to vertex attributes such as color, normals and texture coordinates. These functions are designed to get high graphics performance from modern graphics hardware. They should be used whenever possible rather than the older single primitive functions such as vgl_DrawFunPolyLine, vgl_DrawFunPolyLineColor and vgl_DrawFunPolyLineData. The two function types are differentiated by their use of on-board graphics processing memory. The first set of functions delivers graphics primitives atomically from client memory. The specific functions for each of these dimensional classes of primitives are as follows, vgl_DrawFunPolyPointArray, vgl_DrawFunPolyLineArray and vgl_DrawFunPolygonArray. The second set of functions are specifically designed to use graphics card memory in the form of "vertex buffer objects". The drawing functions vgl_DrawFunInitBuffer, vgl_DrawFunTermBuffer, vgl_DrawFunCopyBuffer are required to specifically initialize buffer memory, delete it and copy data from client to buffer memory. Then in addition there are the usual functions to draw points, lines and polygons once the buffer objects have been generated, vgl_DrawFunPolyPointBuffer, vgl_DrawFunPolyLineBuffer and vgl_DrawFunPolygonBuffer.
Vertex color may be entered as float values ranging from (0.,1.) or unsigned char values in the interval (0,255). When entering color as float values two options are available, VGL_COLOR_3F enters red,green,blue components and VGL_COLOR_4F enters red,green,blue,alpha components. If VGL_COLOR_3F is used, the alpha component is set to 1. When unsigned char values are used for color, the alpha value must be explicitly included. The reason for this is to ensure that vertex color data lies on 4 byte boundaries for graphics performance.
Vertex normals may be entered as float values ranging from (-1.,1.) using VGL_NORMAL_3F or signed char values in the interval (-128,127) using VGL_NORMAL_4B. The 4th byte is unused but should be set to 0. The 4 byte format is utilized to ensure graphics performance. Vertex coordinates and texture coordinates are entered as float values.
Text functions are drawn using a raster font. The text drawing functions are vgl_DrawFunText and vgl_DrawFunTextDC.
All device interface modules treat graphics attributes and primitives procedurally. This means that once a particular graphics attribute is set, all subsequent graphics primitives use the current value of the graphics attribute. Color is treated as a special attribute in that its value may be set independently of any graphics primitive or supplied directly in the graphics primitive function call.
6.6.1 Geometric Primitives
VglTools supports a variety of point, line and polygon geometric primitive types. Points are drawn by simply drawing a point at each of n vertices, however lines and polygons may be drawn through n vertices in more than one way. These line and polygon primitive types are illustrated in Figure 7-1.
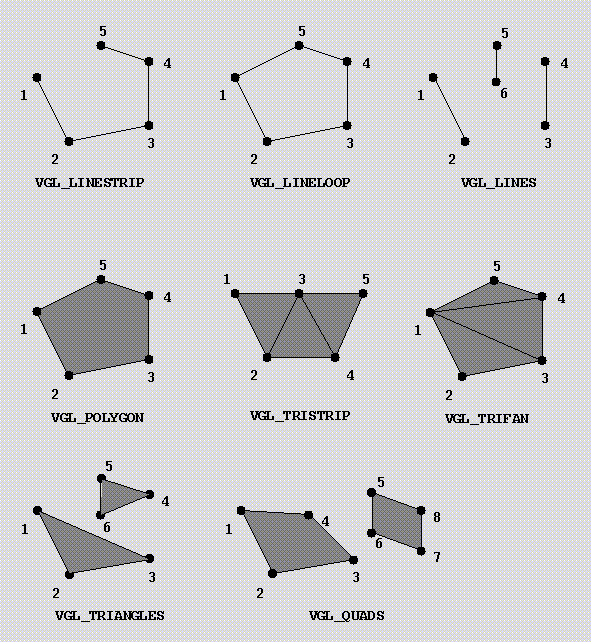
Figure 7-1, Geometric Primitive Types
VGL_LINESTRIP draws a straight line from 1 to 2, then 2 to 3, etc. to n-1 to n until n-1 lines are drawn. VGL_LINELOOP is like VGL_LINESTRIP except that an additional straight line is drawn from n to 1. VGL_LINES draws n/2 lines using n vertices. The first line 1,2 is drawn then 3,4 , etc. VGL_POLYGON draws an n sided polygon using n vertices. VGL_TRISTRIP draws n-2 triangles using n vertices. First triangle 1,2,3 is drawn then 3,2,4 then 3,4,5, etc. VGL_TRIFAN draws n-2 triangles using n vertices. First triangle 1,2,3 is drawn then 1,3,4 then 1,4,5, etc. VGL_TRIANGLES draws n/3 triangles using n vertices. First triangle 1,2,3 is drawn then 4,5,6, etc. VGL_QUADS draws n/4 quadrilaterals using n vertices. First quadrilateral 1,2,3,4 is drawn then 5,6,7,8, etc.6.7 Raster Fonts
Raster fonts are used to draw raster text with vgl_DrawFunText or vgl_DrawFunTextDC. Raster text is drawn in the current font and color.Raster text can be drawn either parallel to the display surface relative to a world coordinate anchor point (using bitmap technology internally) or oriented along a path lying in a specified plane (using texture map technology internally). Orientable raster fonts must be defined using the RasFont module. The current raster font orientation is specified using vgl_DrawFunTextPlane. The world coordinate size of a "raster" in an orientable raster font may be set using vgl_DrawFunTextPixelSize.
A user may define any number of raster fonts indexed by a positive integer. Raster font index 0 is the default, built-in raster font and may not be changed by the user. The RasFont module is used to define raster fonts. Use vgl_DrawFunRasFontDefine to associate a RasFont object with a raster font index. Once defined, a user defined raster font may be selected as the current raster font using vgl_DrawFunRasFontSelect. Generally the default raster font gives the best graphics performance. User defined fonts may be slower. Use vgl_DrawFunGetInteger to return information about the current font.
6.8 Bitmaps
Bitmaps are used to stipple polygons drawn with vgl_DrawFunPolygon, vgl_DrawFunPolygonColor, vgl_DrawFunPolygonDC or vgl_DrawFunPolygonData. The stipple data and parameters are defined using the Bitmap object.A user may define any number of bitmaps indexed by a positive integer. Bitmap index 0 is used to disable polygon stippling.
The Bitmap module is used to define bitmaps. Use vgl_DrawFunBitmapDefine to associate a Bitmap object with a bitmap index. Once defined, a user defined bitmap may be selected as the current bitmap using vgl_DrawFunBitmapSelect. Use vgl_DrawFunGetInteger to return information about the current bitmap.
Polygon stippling is also used to support transparency using a "screen door" technique. If the VGL_BLENDTRANSMODE is not enabled then polygon stippling is used to simulate transparency. In this case if a non zero bitmap index is selected, this bitmap stipple will override any stipple used for screen door transparency. Selecting a zero bitmap will reinstate any stipple used for transparency.
6.9 Texture Maps
Texture maps are used to modify color with texture data with vgl_DrawFunPolygon, vgl_DrawFunPolygonColor or vgl_DrawFunPolygonData. The basic motivation for using textures is to increase image complexity wihtou increasing the number of graphics primitives (eg. polygons).In VglTools, texture data and parameters are defined using a Texture object. A user may define any number of texture maps indexed by a positive integer. Texture map index 0 is used to disable texture mapping. The Texture module is used to define texture maps. Use vgl_DrawFunTextureDefine to associate a Texture object with a texture map index. Once defined, a user defined texture map may be selected as the current texture map using vgl_DrawFunTextureSelect. Use vgl_DrawFunGetInteger to return information about the current texture map.
While texture mapping may be theorically applied to all point, line and polygon primitives, the current release of VglTools supports texture mapping for polygons only. Vertex normals and texture coordinates may be specified in the argument list of the Polygon, PolygonColor and PolygonData drawing functions. If a texture mapping is enabled an no texture coordinates are specified, zero values are assumed for the texture coordinates.
6.10 Pixel Level Operations
VglTools supplies two methods for reading and writing pixel information. The methods use three other modules, Pixelmap, FBuffer and ZBuffer to provide a complete functionality.The first method utilizes a Pixelmap object to store frame buffer or zbuffer information in a device dependent manner. It is optimized for reading and writing an entire buffer as quickly as possible with no alteration of the buffer contents. This is useful for creating and replaying animations of complex scenes. Use vgl_DrawFunPixelmapCreate and vgl_DrawFunPixelmapDestroy to allocate and free the memory required to save a pixelmap. Use vgl_DrawFunPixelmapRead and vgl_DrawFunPixelmapWrite to read/write the contents of a buffer to/from a Pixelmap object. Use vgl_PixelmapSetBuffer to set the buffer type (frame buffer or zbuffer) to be read/written.
The second method of reading and writing frame buffer pixels is device independent and utilizes a FBuffer object. In addition the ZBuffer object can be used to read and write z buffer information in a device independent manner. An FBuffer object can be used to store any selected rectangle of pixels in a frame buffer. Once stored in an FBuffer object the pixels can be written to any other VglTools supported device. FBuffer objects may also be written to disk files. This is useful for saving images or creating hardcopy of generated images. Use vgl_DrawFunFBufferRead and vgl_DrawFunFBufferWrite to read/write the contents of a frame buffer to/from an FBuffer object. Use FBuffer functions to transfer the frame buffer contents to disk, modify the image, etc. Use vgl_DrawFunZBufferRead and vgl_DrawFunZBufferWrite to read/write the contents of a z buffer to/from a ZBuffer object.
6.11 Selection, Extent and Buffer Mode
In normal rendering mode, VglTools rasterizes graphics primitives and writes the results to the frame buffer. VglTools provides three alternate rendering modes, selection mode, extent mode and buffer mode, in which the rasterized primitives are not written to the frame buffer but instead are processed to aid in other graphics related operations such as object picking and extent box calculation. In either selection or extent mode z-buffering is not performed. The user switches between the various rendering modes using vgl_DrawFunRender.
6.11.1 Selection Mode
Selection mode may be used to identify objects lying within a particular region of the graphics screen. Three types of selection regions may be specified, a conventional rectangular region, a non-simple polygonal region, and a region defined by the current clipping planes. The selection region is defined using vgl_DrawFunSelectRegion. All selection regions are defined in terms of device coordinates. In selection mode, each graphics primitive which lies within the selection region is recorded as a "hit" in a set of three hit buffers which are defined using vgl_DrawFunSelectBuffer.There are two possible formats in which hit records are recorded. By default A hit record consists of the current DataIndex attribute at the top of the index stack within the selection region. As an option, a hit record can consist of the number of indices in the index stack followed by the indices in the index stack. In either case the minimum and maximum depth coordinate of the primitive is recorded. The format of the hit record is determined by setting the VGL_SELECTSTACKMODE using vgl_DrawFunSetMode.
The number of hits may be returned using vgl_DrawFunSelectQuery. Once a hit is recorded for the current DataIndex attribute, no further hits are recorded unitl the DataIndex attribute is changed. In this way duplicative consecutive hits are not recorded for a given DataIndex.
The three types of selection regions use two different algorithms for determining if a given primitive hits the selection region. For rectangular regions, the primitive must intersect the rectangle. For polygonal and clipping plane regions, a vertex of the primitive must lie within the polygon or clipping planes.
6.11.2 Extent Mode
Extent mode is used to determine the bounding box of a set of primitives. The bounding box may be computed in world, eye, normalized device or device coordinates. This mode is useful for "fitting" an object within a given viewport or computing world coordinate extent boxes for interference or occlusion testing, etc. No view volume, viewport or depth range clipping is performed in extent mode. Therefore, the extent box returned by vgl_DrawFunExtentQuery will include coordinates outside of the limits of the viewport, window or view volume.
6.11.3 Buffer Mode
Buffer mode is used to render to off-screen frame buffer objects. There is one basic option, render color to an off-screen color buffer. This option performs normal color rendering to an off-screen frame buffer object of a user specified size. By default the size is the same as the window but it can be explicitly set using vgl_DrawFunBufferSize.6.12 Shader Assisted Rendering
By default, the 3D device module OpenGLDev utilize a "fixed function" rendering pipeline. However, there are a number of rendering features, such as order independent transparency (OIT) and sphere point styles, which require that a custom program, a shader, be executed on the graphics processing unit (GPU). Many of the rendering options require additional parameters. These parameters are set using the vgl_DrawFunSetFactors. Currently the following rendering options require that shaders be activated before the rendering mode is enabled. Note that any combination of shader assisted rendering options may be activated at any time. Activate shaders using:vgl_DrawFunSetMode (drawfun,VGL_SHADERMODE,VGL_ON);
- Circle, sphere, number and bitmap point styles. These point styles selected with vgl_DrawFunPointStyle require shaders.
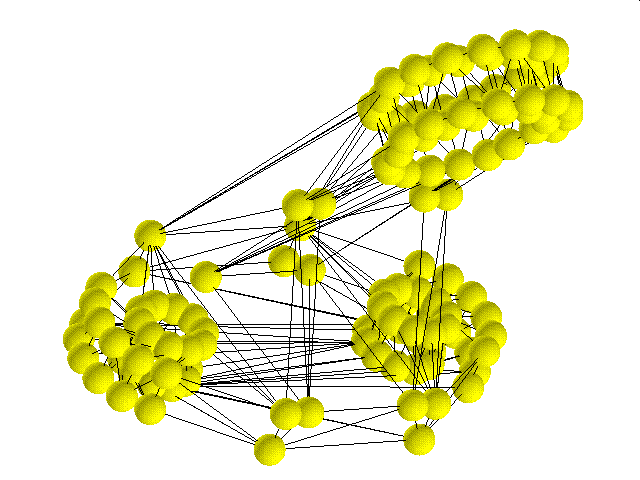
Figure 7-2, Device sized spheres
-
Z Buffer Edge Detection. This rendering feature will accentuate pixels
along edges with respect to the magnitude of z-buffer discontinuity at
the point. The lightness of the pixel will be reduced depending
upon the magnitude of the discontinuity. This feature is very
useful in highlighting the edges of surfaces in general to make them
more visually distinct. It is especially useful for geometries in which
there are predominantly parallel surfaces which would otherwise have
very similar lighting characteristics. Such a model is drawn
without and with z-buffer edge detection in Figures 7-2a and 7-2b
respectively.
Activate this feature using:
vgl_DrawFunSetMode (drawfun,VGL_ZBUFFEREDGEMODE,VGL_ON);
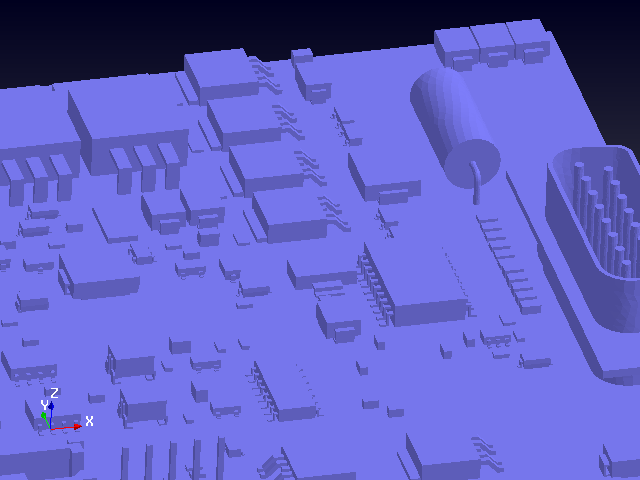
Figure 7-2a, Model Drawn without Z Buffer Edge Detection
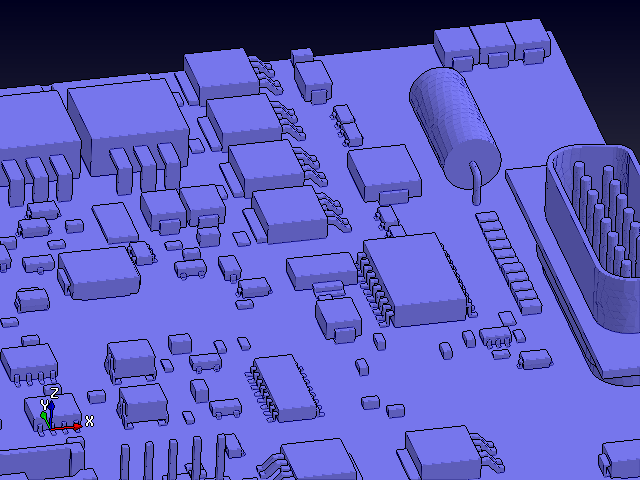
Figure 7-2b, Model Drawn with Z Buffer Edge Detection
-
Depth Peeling. This is a multipass rendering feature which will
closely approximate the proper rendering of transparent surfaces.
Since this is a multipass algorithm, the amount of rendering performed
at each pass, or peel, should be limited as much as possible for
performance reasons. Each peel only requires that the transparent
objects be rendered. Therefore the opaque objects need only be
rendered once, usually first. Then successive depth peels are
performed (up to four), rendering only transparent objects.
A rendering of transparent geometry using depth peeling is
illustrated in Figure 3.
The basic structure of function calls is as follows:
/* ensure that peel mode is off to start */ vgl_DrawFunSetMode (drawfun,VGL_DEPTHPEELMODE,VGL_OFF); vgl_DrawFunClear (drawfun);
Render any opaque objects./* enable peel 1 */ vgl_DrawFunSetMode (drawfun,VGL_DEPTHPEELMODE,1);
Render transparent objects./* enable peel 2 */ vgl_DrawFunSetMode (drawfun,VGL_DEPTHPEELMODE,2);
Render transparent objects./* enable peel 3 */ vgl_DrawFunSetMode (drawfun,VGL_DEPTHPEELMODE,3);
Render transparent objects./* enable last peel */ vgl_DrawFunSetMode (drawfun,VGL_DEPTHPEELMODE,VGL_DEPTHPEELLAST);
Render transparent objects./* turn off peeling */ vgl_DrawFunSetMode (drawfun,VGL_DEPTHPEELMODE,VGL_OFF);
Render any opaque objects.vgl_DrawFunSwap (drawfun);
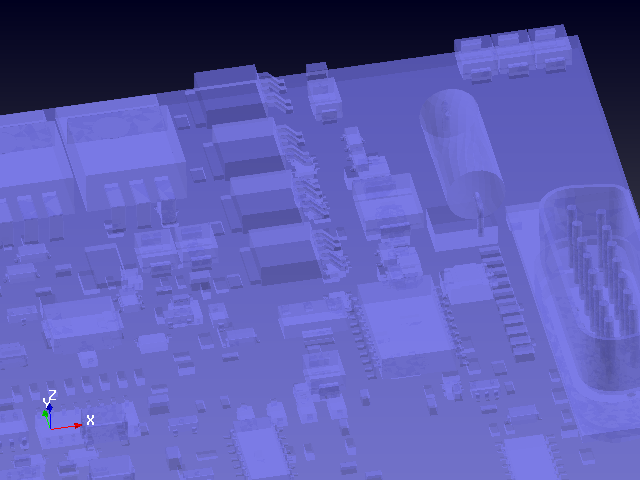
Figure 7-3, Model Drawn with Depth Peeling
-
Order independent transparency (OIT). This is a single pass
rendering technology which caches all fragments into a GPU
resident data structure. When vgl_DrawFunSwap is called
the fragment structure is composited. This algorithm is heavily
dependent on GPU memory and, as a result, may
result in artifacts if memory resources are exhausted.
Activate this feature using:
vgl_DrawFunSetMode (drawfun,VGL_OITMODE,VGL_ON);
GPU memory for this feature must be allocated internally before rendering. The memory required in bytes is 4*3*number_pixels*layers. Where 4*3 bytes is the memory required to store a single fragment. The average depth is set using:vgl_DrawFunSetMode (drawfun,VGL_OITLAYERS,layers);
A GPU memory allocation failure may be queried as follows. A non-zero flag indicates a memory failure.vgl_DrawFunGetInteger (drawfun,VGL_OIT_MEMORYFAIL,&flag);
The count of the current number of fragments encountered and the maximum number of fragments which can be stored may be queried as follows, where icount[0] is the maximum count and icount[1] is the current count.vgl_DrawFunGetInteger (drawfun,VGL_OIT_COUNTERS,icount);
-
Screen Space Ambient Occlusion. This rendering feature will perform
ambient occlusion shading. The algorithm detects tight corners, etc.
where ambient light is occluded due to surrounding geometry. The
computations are performed given screen space coordinates which
are then transformed to physical coordinates to perform the
occlusion calculations. This feature requires the user to compute
a distance factor which defines the boundary of the region about a point
within which geometry would be considered in the occlusion computation.
A reasonable value for this distance would be 5 percent of the
world coordinate extent of the model to be displayed.
This factor is set using vgl_DrawFunSetFactors.
Activate this feature using:
vgl_DrawFunSetMode (drawfun,VGL_SSAOMODE,VGL_ON);
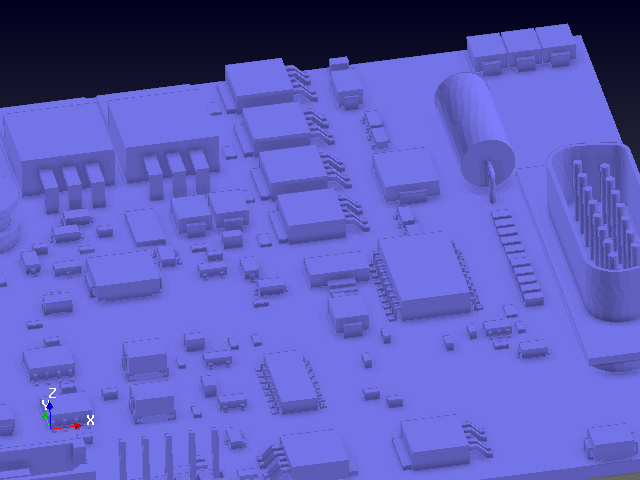
Figure 7-4, Model Drawn with Screen Space Ambient Occlusion
6.13 Graphics Input
VglTools provides a simple set of functions for reading the keyboard and mouse input devices and controlling the style and location of the cursor. Input values are returned using both polling and queuing techniques. Polling immediately returns the value of a device, queuing uses an event queue to save changes in device values and other events so that they can be accessed later.Two functions are used to poll the state of the mouse and selected keyboard keys: vgl_DrawFunPollMouse returns the current pixel location of the mouse and the state of all mouse buttons, vgl_DrawFunPollModifiers returns the current state of the keyboard Control and Shift keys.
Input devices and other window operations create entries in the event queue. Each entry consists of an event token and an associated event value. The functions vgl_DrawFunTestQueue, vgl_DrawFunReadQueue and vgl_DrawFunResetQueue are used to test for entries in the event queue, read events from the event queue and flush all events from the event queue. Events which are currently supported are mouse button events, keyboard events and exposure events. Dialbox motion events are also supported as an X-windows extension. By default VglTools will only recognize exposure events. Use vgl_DrawFunSetMode with VGL_EVENTQUEUEMODE enabled to recognize button and key events.
The style and location of the cursor are controlled by vgl_DrawFunSetCursor and vgl_DrawFunWarpMouse.
6.14 Function Descriptions
The currently available DrawFun object functions are described in detail in this section.DrawFun
NAME
*vgl_DrawFunBegin - create an instance of a DrawFun objectC SPECIFICATION
vgl_DrawFun *vgl_DrawFunBegin ()
ARGUMENTS
None
FUNCTION RETURN VALUE
The function returns a pointer to the newly created DrawFun object. If the object creation fails, NULL is returned.DESCRIPTION
Create an instance of a DrawFun object. Memory is allocated for the object private data and the pointer to the data is returned. By default all function pointers are NULL.Destroy an instance of a DrawFun object using
void vgl_DrawFunEnd (vgl_DrawFun *drawfun)
Return the current value of a DrawFun object error flag using
Vint vgl_DrawFunError (vgl_DrawFun *drawfun)
Make a copy of a DrawFun object. The private data from the fromdrawfun object is copied to the drawfun object. Any previous private data in drawfun is lost.
void vgl_DrawFunCopy (vgl_DrawFun *drawfun,vgl_DrawFun *fromdrawfun)
DrawFun
NAME
vgl_DrawFunSet - set pointer to drawing functionC SPECIFICATION
void vgl_DrawFunSet (vgl_DrawFun *drawfun, Vint type, void (*function)())
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. type Function type being set =DRAWFUN_CLOSEWINDOW Set CloseWindow function =DRAWFUN_CONFIGUREWINDOW Set ConfigureWindow function =DRAWFUN_CONNECTWINDOW Set ConnectWindow function =DRAWFUN_DISCONNECTWINDOW Set DisconnectWindow function =DRAWFUN_OPENWINDOW Set OpenWindow function =DRAWFUN_PARENTWINDOW Set ParentWindow function =DRAWFUN_POSITIONWINDOW Set PositionWindow function =DRAWFUN_QUERYWINDOW Set QueryWindow function =DRAWFUN_SETWINDOW Set SetWindow function =DRAWFUN_VISUALWINDOW Set VisualWindow function =DRAWFUN_BACKCOLORINDEX Set BackColorIndex function =DRAWFUN_BACKCOLORRGB Set BackColor function =DRAWFUN_BUFFERSIZE Set BufferSize function =DRAWFUN_BELL Set Bell function =DRAWFUN_CLEAR Set Clear function =DRAWFUN_DELAY Set Delay function =DRAWFUN_FLUSH Set Flush function =DRAWFUN_RESIZE Set Resize function =DRAWFUN_RENDER Set Render function =DRAWFUN_SETMODE Set SetMode function =DRAWFUN_SETSWITCH Set SetSwitch function =DRAWFUN_SWAP Set Swap function =DRAWFUN_EXTENTQUERY Set ExtentQuery function =DRAWFUN_GETFLOAT Set GetFloat function =DRAWFUN_GETINTEGER Set GetInteger function =DRAWFUN_GETMODE Set GetMode function =DRAWFUN_GETSTRING Set GetString function =DRAWFUN_SELECTBUFFER Set SelectBuffer function =DRAWFUN_SELECTQUERY Set SelectQuery function =DRAWFUN_SELECTREGION Set SelectRegion function =DRAWFUN_CLIP Set Clip function =DRAWFUN_CLIPPLANE Set ClipPlane function =DRAWFUN_DEPTHRANGE Set DepthRange function =DRAWFUN_PROJFRUSTUM Set ProjFrustum function =DRAWFUN_PROJORTHO Set ProjOrtho function =DRAWFUN_PROJPOP Set ProjPop function =DRAWFUN_PROJPUSH Set ProjPush function =DRAWFUN_PROJLOAD Set ProjLoad function =DRAWFUN_VIEWPORT Set Viewport function =DRAWFUN_XFMLOAD Set XfmLoad function =DRAWFUN_XFMMULT Set XfmMult function =DRAWFUN_XFMPUSH Set XfmPush function =DRAWFUN_XFMPOP Set XfmPop function =DRAWFUN_LIGHT Set Light function =DRAWFUN_LIGHTMODEL Set LightModel function =DRAWFUN_BITMAPDEFINE Set BitmapDefine function =DRAWFUN_BITMAPSELECT Set BitmapSelect function =DRAWFUN_RASFONTDEFINE Set RasFontDefine function =DRAWFUN_RASFONTSELECT Set RasFontSelect function =DRAWFUN_TEXTUREDEFINE Set TextureDefine function =DRAWFUN_TEXTURESELECT Set TextureSelect function =DRAWFUN_ATTPOP Set AttPop function =DRAWFUN_ATTPUSH Set AttPush function =DRAWFUN_COLORINDEX Set ColorIndex function =DRAWFUN_COLORRGB Set Color function =DRAWFUN_DATA Set Data function =DRAWFUN_DATAINDEX Set DataIndex function =DRAWFUN_LINESTYLE Set LineStyle function =DRAWFUN_LINEWIDTH Set LineWidth function =DRAWFUN_POINTSIZE Set PointSize function =DRAWFUN_POINTSTYLE Set PointStyle function =DRAWFUN_POINTER Set Pointer function =DRAWFUN_POLYGONMODE Set PolygonMode function =DRAWFUN_POLYGONOFFSET Set PolygonOffset function =DRAWFUN_SPECULARITY Set Specularity function =DRAWFUN_TRANS Set Trans function =DRAWFUN_TRANSINDEX Set TransIndex function =DRAWFUN_INITBUFFER Set InitBuffer function =DRAWFUN_COPYBUFFER Set CopyBuffer function =DRAWFUN_TERMBUFFER Set TermBuffer function =DRAWFUN_POLYPOINT Set PolyPoint function =DRAWFUN_POLYPOINTARRAY Set PolyPointArray function =DRAWFUN_POLYPOINTBUFFER Set PolyPointBuffer function =DRAWFUN_POLYPOINTCOLOR Set PolyPointColor function =DRAWFUN_POLYPOINTDATA Set PolyPointData function =DRAWFUN_POLYPOINTDC Set PolyPointDC function =DRAWFUN_POLYLINE Set PolyLine function =DRAWFUN_POLYLINEARRAY Set PolyLineArray function =DRAWFUN_POLYLINEBUFFER Set PolyLineBuffer function =DRAWFUN_POLYLINECOLOR Set PolyLineColor function =DRAWFUN_POLYLINEDATA Set PolyLineData function =DRAWFUN_POLYLINEDC Set PolyLineDC function =DRAWFUN_POLYGON Set Polygon function =DRAWFUN_POLYGONARRAY Set PolygonArray function =DRAWFUN_POLYGONBUFFER Set PolygonBuffer function =DRAWFUN_POLYGONCOLOR Set PolygonColor function =DRAWFUN_POLYGONDATA Set PolygonData function =DRAWFUN_POLYGONDC Set PolygonDC function =DRAWFUN_POLYARRAY Set PolyArray function =DRAWFUN_POLYBUFFER Set PolyBuffer function =DRAWFUN_POLYELEMARRAY Set PolyElemArray function =DRAWFUN_POLYELEMBUFFER Set PolyElemBuffer function =DRAWFUN_TEXT Set Text function =DRAWFUN_TEXTDC Set TextDC function =DRAWFUN_TEXTPIXELSIZE Set TextPixelSize function =DRAWFUN_TEXTPLANE Set TextPlane function =DRAWFUN_FBUFFERREAD Set FBufferRead function =DRAWFUN_FBUFFERWRITE Set FBufferWrite function =DRAWFUN_ZBUFFERREAD Set ZBufferRead function =DRAWFUN_ZBUFFERWRITE Set ZBufferWrite function =DRAWFUN_PIXELMAPCREATE Set PixelmapCreate function =DRAWFUN_PIXELMAPDESTROY Set PixelmapDestroy function =DRAWFUN_PIXELMAPREAD Set PixelmapRead function =DRAWFUN_PIXELMAPWRITE Set PixelmapWrite function =DRAWFUN_POLLMOUSE Set PollMouse function =DRAWFUN_POLLMODIFIERS Set PollModifiers function =DRAWFUN_WARPMOUSE Set WarpMouse function =DRAWFUN_SETCURSOR Set SetCursor function =DRAWFUN_READQUEUE Set ReadQueue function =DRAWFUN_TESTQUEUE Set TestQueue function =DRAWFUN_RESETQUEUE Set ResetQueue function function Pointer to drawing function
OUTPUT ARGUMENTS
None
ERRORS
VGL_ERROR_ENUM is generated if an improper type is input.DESCRIPTION
Set pointers to drawing functions.Get function as an output argument using
void vgl_DrawFunGet (vgl_DrawFun *drawfun, Vint type, void (**function)())
DrawFun
NAME
vgl_DrawFunSetObj - set pointer to auxiliary objectC SPECIFICATION
void vgl_DrawFunSetObj (vgl_DrawFun *drawfun, Vobject *obj)
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. obj Pointer to auxiliary object
OUTPUT ARGUMENTS
None
DESCRIPTION
Set pointer to drawing function auxiliary object.Get obj as an output argument using
void vgl_DrawFunGetObj (vgl_DrawFun *drawfun, Vobject **obj)
DrawFun
NAME
vgl_DrawFunAPI - set built-in drawing functionsC SPECIFICATION
void vgl_DrawFunAPI (vgl_DrawFun *drawfun, Vint api)
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. api Type of built-in drawing function to return. =DRAWFUN_APIPRINT Set printing drawing functions =DRAWFUN_APIRETURN Set return drawing functions
OUTPUT ARGUMENTS
None
ERRORS
SYS_ERROR_ENUM is generated if an improper api is input.DESCRIPTION
Set built in drawing functions. The DRAWFUN_APIPRINT drawing functions print each graphics primitive to standard output. The DRAWFUN_APIRETURN drawing functions simply return.
6.15 Graphics Function Descriptions
The currently available graphics drawing function API is described in detail in this section. The functions appear in alphabetical order.DrawFun
NAME
vgl_DrawFunAttPop,vgl_DrawFunAttPush - pop and push graphics attribute stackC SPECIFICATION
void vgl_DrawFunAttPop (vgl_DrawFun *drawfun) void vgl_DrawFunAttPush (vgl_DrawFun *drawfun, Vint mask)
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. mask A mask which indicates which graphics attributes to push. The mask is constructed by ORing one or more of the following. =VGL_COLORATT Push color, transparency =VGL_POINTATT Push point size =VGL_LINEATT Push line width and line style =VGL_POLYGONATT Push specularity =VGL_VIEWPORTATT Push viewport, depth range
OUTPUT ARGUMENTS
None
DESCRIPTION
Push and pop selected sets of graphics attributes to and from the attribute stack. When the attribute stack is popped, only those graphics attributes saved with the last call to vgl_DrawFunAttPush are restored. All other attributes remain unchanged. The attribute stack is initially empty. The depth of the attribute stack is at least 32.
DrawFun
NAME
vgl_DrawFunBackColor,vgl_DrawFunBackColorIndex - set background or clear colorC SPECIFICATION
void vgl_DrawFunBackColor (vgl_DrawFun *drawfun, Vfloat c[3]) Vfloat c[3]) void vgl_DrawFunBackColorIndex (vgl_DrawFun *drawfun, Vint index)
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. c Current background color RGB values index Current background color index
OUTPUT ARGUMENTS
None
DESCRIPTION
Set the background or clear color. This color is used by vgl_DrawFunClear to clear the frame buffer. Red, green and blue (RGB) color values are in the interval [0.,1.] where 0. represents zero intensity and 1. represents full intensity. By default the clear color is black. VglTools graphics device interface modules do not support vgl_DrawFunBackColorIndex.DrawFun
NAME
vgl_DrawFunBell - ring bellC SPECIFICATION
void vgl_DrawFunBell (vgl_DrawFun *drawfun)
INPUT ARGUMENTS
drawfun Pointer to DrawFun object.
OUTPUT ARGUMENTS
None
DESCRIPTION
Sound a bell on an interactive graphics display device. This function is ignored by device interface modules not rendering to a graphics window.DrawFun
NAME
vgl_DrawFunClear - clear frame buffer to background colorC SPECIFICATION
void vgl_DrawFunClear (vgl_DrawFun *drawfun)
INPUT ARGUMENTS
drawfun Pointer to DrawFun object.
OUTPUT ARGUMENTS
None
DESCRIPTION
Clear the current frame buffer to the color specified by vgl_DrawFunBackColor or vgl_DrawFunBackColorIndex.
DrawFun
NAME
vgl_DrawFunBitmapDefine - define a bitmap indexC SPECIFICATION
void vgl_DrawFunBitmapDefine (vgl_DrawFun *drawfun, Vint index, vgl_Bitmap *bitmap)
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. index Bitmap index, 1 <= index bitmap Pointer to Bitmap object.
OUTPUT ARGUMENTS
None
ERRORS
VGL_ERROR_VALUE is generated if an index out of range is specified. VGL_ERROR_NULLOBJECT is generated if a NULL Bitmap object is input.DESCRIPTION
Define a bitmap. Use the Bitmap module to build the bitmap description. Bitmap objects are copied by the graphics interface object. If a bitmap had been previously defined for an index, the current definition replaces it. Use vgl_DrawFunBitmapSelect to select the current bitmap index once it has been defined.
DrawFun
NAME
vgl_DrawFunBitmapSelect - select current bitmapC SPECIFICATION
void vgl_DrawFunBitmapSelect (vgl_DrawFun *drawfun, Vint index)
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. index Bitmap index, 0 <= index
OUTPUT ARGUMENTS
None
ERRORS
VGL_ERROR_VALUE is generated if an index out of range is specified.DESCRIPTION
Select a current bitmap index. This bitmap remains in effect until vgl_DrawFunBitmapSelect is called again. Use vgl_DrawFunBitmapDefine to define a bitmap index. A bitmap index of 0 disables polygon stippling. Bitmap index 0 is the default.
DrawFun
NAME
vgl_DrawFunBufferSize - specify color buffer sizeC SPECIFICATION
void vgl_DrawFunBufferSize (vgl_DrawFun *drawfun, Vint xsize, Vint ysize)
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. xsize Horizontal size of off-screen color buffer ysize Vertical size of off-screen color buffer
OUTPUT ARGUMENTS
None
DESCRIPTION
Specify size of off-screen color buffer if in VGL_BUFFER_COLOR rendering mode. By default the size is equal to the window size. Values of xsize or ysize of zero indicate an off-screen color buffer the size of the window. Note that the aspect ratio of the off-screen frame buffer should match that of the window if the projection and modelview matrices are left unchanged. This will ensure that the image is not warped in any way.
DrawFun
NAME
vgl_DrawFunClip - define clipping rectangleC SPECIFICATION
void vgl_DrawFunClip (vgl_DrawFun *drawfun, Vint left, Vint right, Vint bottom, Vint top)
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. left Left device coordinate limit of clipping rectangle right Right device coordinate limit of clipping rectangle bottom Bottom device coordinate limit of clipping rectangle top Top device coordinate limit of clipping rectangle
OUTPUT ARGUMENTS
None
DESCRIPTION
Define a clipping rectangle in device coordinates. If clipping is disabled then the clipping is performed at the viewport boundaries. If clipping is enabled then clipping is performed to the defined clipping rectangle. Set the clipping mode using vgl_DrawFunSetMode. Disabling clipping resets the clipping rectangle to the current viewport.
DrawFun
NAME
vgl_DrawFunCloseWindow - close a graphics window or fileC SPECIFICATION
void vgl_DrawFunCloseWindow (vgl_DrawFun *drawfun)
INPUT ARGUMENTS
drawfun Pointer to DrawFun object.
OUTPUT ARGUMENTS
None
ERRORS
VGL_ERROR_OPERATION is generated if the window is not open.DESCRIPTION
Close a graphics window. A graphics device interface object which draws to an open X window or Microsoft Windows window will free memory resources and close the window. Use vgl_DrawFunOpenWindow to open a graphics window.
DrawFun
NAME
vgl_DrawFunClipPlane - define clipping planeC SPECIFICATION
void vgl_DrawFunClipPlane (vgl_DrawFun *drawfun, Vint index, Vfloat equation[4])
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. index Clipping plane index, 0 <= index <= 5; equation Coefficients A,B,C,D of equation of clipping plane
OUTPUT ARGUMENTS
None
DESCRIPTION
Define a clipping plane. The coefficients of the plane equation, Ax+By+Cz+D = 0 define the clipping plane. The 4 coefficients are entered in equation. The clipping plane is given an index. Up to 6 clipping planes may be defined. When defined, the coefficients of the plane equation are transformed by the inverse of the current modelview matrix. Any subsequent changes to the modelview matrix do not affect the clipping plane. If the dot product of the transformed plane equation with the eye coordinates (transformed only by the modelview matrix) of an input vertex is negative, the vertex is clipped otherwise it is not. By default clipping planes are assumed to be disabled. Use vgl_DrawFunSetSwitch to enable and disable clipping planes.
DrawFun
NAME
vgl_DrawFunColor,vgl_DrawFunColorIndex - set current colorC SPECIFICATION
void vgl_DrawFunColor (vgl_DrawFun *drawfun, Vfloat c[3]) void vgl_DrawFunColorIndex (vgl_DrawFun *drawfun, Vint index)
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. c Current color RGB values index Current color index
OUTPUT ARGUMENTS
None
DESCRIPTION
Set the current color. Red, green and blue (RGB) color values are in the interval [0.,1.] where 0. represents zero intensity and 1. represents full intensity. VglTools graphics device interface modules do not support vgl_DrawFunColorIndex. By default the current color is black.
DrawFun
NAME
vgl_DrawFunConfigureWindow - configure window size and positionC SPECIFICATION
void vgl_DrawFunConfigureWindow (vgl_DrawFun *drawfun, Vint oper, Vint params[])
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. oper Window configuration operation. The information expected in params depends upon the operation. =VGL_WINDOWLOWER Lower the window =VGL_WINDOWORIGIN Specify the window origin =VGL_WINDOWORIGINSIZE Specify the window origin and size =VGL_WINDOWRAISE Raise the window =VGL_WINDOWSIZE Specify the window size =VGL_WINDOWSTATEINIT Inherit the window graphics state =VGL_WINDOWSTATETERM Restore the window graphics state params Configuration parameters
OUTPUT ARGUMENTS
None
DESCRIPTION
Request a position and size of a window associated with a graphics interface device object. The window origin is the lower left hand corner of the drawable portion (client area) of the window. The drawable portion of the window does not include the window borders or title bar. Similarly, the window size is in reference to the size in pixels of the drawable portion of the window.If this function is called before a window is opened, then the requested origin and/or size are enforced when the window is opened. If this function is called after a window is opened, then the specified window configuration is enforced immediately.
The VGL_WINDOWORIGIN operation requires the horizontal and vertical origin of the window in pixels measured from the lower left corner of the screen or parent window. The VGL_WINDOWORIGINSIZE operation requires the horizontal and vertical origin and size of the window in pixels. The VGL_WINDOWSIZE operation just requires the horizontal and vertical size of the window in pixels. By default the location of the lower left hand corner of the window is (100,100) and the size is (400,300).
The VGL_WINDOWSTATEINIT operation forces the device object (for example, OpenGLDev) to inherit the window graphics state. Use VGL_WINDOWSTATETERM to return the window graphics state to the same condition it was upon VGL_WINDOWSTATEINIT. The params argument is unused and may be set to NULL. This function is necessary for embedded graphics applications which must share the graphics state with another application. This function should be called just before drawing to the device object.
DrawFun
NAME
vgl_DrawFunConnectWindow - connect to existing graphics windowC SPECIFICATION
void vgl_DrawFunConnectWindow (vgl_DrawFun *drawfun, Vword window)
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. window Window id or Pixmap if X window. Window HWND or device context HDC if Microsoft Windows
OUTPUT ARGUMENTS
None
ERRORS
VGL_ERROR_OPERATION is generated if the window has already been connected to.DESCRIPTION
Connect a graphics interface module to an existing graphics window or host system file. If the user opts to open and manage the window to which a graphics interface object is to draw, the object must be informed of the window id or handle prior to drawing. Use vgl_DrawFunDisconnectWindow to disconnect from an existing graphics window.By default the window is a Window id in X Windows and a HWND window in Microsoft Windows. If VGL_VISUAL_USECONTEXT is set using vgl_DrawFunVisualWindow, then window is ignored.
DrawFun
NAME
vgl_DrawFunDataIndex - load index valuesC SPECIFICATION
void vgl_DrawFunDataIndex (vgl_DrawFun *drawfun, Vint nprims, Vint nrows, Vint *index)
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. nprims Number of primitives nrows Number of rows in data index vector per primitive index Current index values
OUTPUT ARGUMENTS
None
DESCRIPTION
Load the current data index values. The number of values loaded is nrows*nprims, ie. nrows values for nprims primitives. Any subsequent drawing function which draws graphics primitives such as points, lines or polygons will render nrows indices for each primitive drawn. If the number of primitives drawn exceeds nprims then the last set of nrows indicies will be rendered. A primitive is defined as a single point for point drawing functions, a line segment for line drawing functions and a triangle for polygon drawing functions.
DrawFun
NAME
vgl_DrawFunData - set current dataC SPECIFICATION
void vgl_DrawFunData (vgl_DrawFun *drawfun, Vint nrws, Vfloat d[])
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. nrws Number of values in d vector d Vector of data values
OUTPUT ARGUMENTS
None
DESCRIPTION
Set the current data values. The number of data values, nrws must be in the interval [1,16]. The current data values and index are used by the DataBuf module. VglTools graphics device interface modules do not support vgl_DrawFunData.
DrawFun
NAME
vgl_DrawFunDelay - delay for a specified timeC SPECIFICATION
void vgl_DrawFunDelay (vgl_DrawFun *drawfun, Vfloat seconds)
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. seconds Number of seconds to delay
OUTPUT ARGUMENTS
None
DESCRIPTION
This function suspends execution for a specified time, seconds. It is useful to control the maximum speed of an animation. It is like the "sleep" functions in UNIX and Microsoft Windows. Generally the resolution of the sleep is a fraction of a second.
DrawFun
NAME
vgl_DrawFunDepthRange - define depth range viewport transformationC SPECIFICATION
void vgl_DrawFunDepthRange (vgl_DrawFun *drawfun, Vfloat znear, Vfloat zfar)
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. znear,zfar Normalized coordinates of near and far planes of viewing volume
OUTPUT ARGUMENTS
None
DESCRIPTION
Define a viewport transformation. The specified viewport transformation replaces the current viewport transformation. vgl_DrawFunDepthRange specifies the mapping of the depth bounds of the viewing volume to the dynamic range of the z-buffer. The depth range is specified in a normalized coordinate in the interval [0.,1.]. The znear and zfar values are clamped to the interval [0.,1.] before they are accepted. Initially the depth range is set to znear = 0. and zfar = 1.
DrawFun
NAME
vgl_DrawFunDisconnectWindow - disconnect from existing graphics window or fileC SPECIFICATION
void vgl_DrawFunDisconnectWindow (vgl_DrawFun *drawfun)
INPUT ARGUMENTS
drawfun Pointer to DrawFun object.
OUTPUT ARGUMENTS
None
ERRORS
VGL_ERROR_OPERATION is generated if the window is not connected.DESCRIPTION
This function is used in conjunction with vgl_DrawFunConnectWindow to terminate rendering by an object to an existing graphics window or file. This function releases any necessary memory resources allocated by the object but does not close or destroy the graphics window or file.DrawFun
NAME
vgl_DrawFunExtentQuery - query for current extent boxC SPECIFICATION
void vgl_DrawFunExtentQuery(vgl_DrawFun *drawfun, Vfloat *left, Vfloat *right, Vfloat *bottom, Vfloat *top, Vfloat *znear, Vfloat *zfar)
INPUT ARGUMENTS
drawfun Pointer to DrawFun object.
OUTPUT ARGUMENTS
left,right Coordinates of left and right extent limits. bottom,top Coordinates of bottom and top extent limits. znear,zfar Depth coordinates of near and far extent limits.
DESCRIPTION
Return limits of extent box. The extent box is calculated when the rendering mode is set to VGL_EXTENT_DEVICE or VGL_EXTENT_WORLD using vgl_DrawFunRender. If the extent rendering mode has not been enabled, the extent box is returned as all zeros. The coordinates of the extent box are not clipped by the viewing volume, the viewport or depth range. Note that the device coordinates are returned as floating point values. Device coordinates are expressed in pixels and may contain fractional values.If world coordinates are returned then left and right are the minimum and maximum x coordinate, bottom and top are the minimum and maximum y coordinate and znear and zfar are the minimum and maximum z coordinate.
DrawFun
NAME
vgl_DrawFunFBufferRead,vgl_DrawFunFBufferWrite - read and write frame bufferC SPECIFICATION
void vgl_DrawFunFBufferRead(vgl_DrawFun *drawfun, Vint left, Vint right, Vint bottom, Vint top, vgl_FBuffer *fbuffer) void vgl_DrawFunFBufferWrite(vgl_DrawFun *drawfun, Vint left, Vint right, Vint bottom, Vint top, vgl_FBuffer *fbuffer)
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. left,right Pixel coordinates of left and right limits of rectangle bottom,top Pixel coordinates of bottom and top limits of rectangle fbuffer Pointer to FBuffer object to read into or write from.
OUTPUT ARGUMENTS
None
DESCRIPTION
Read frame buffer contents into a FBuffer object or write contents of a FBuffer object to a frame buffer. The user may specify a subset of the frame buffer to be read from by specifying the limits of a rectangle of the frame buffer in pixels with left, right, bottom and top. If these arguments are set to zero, then the entire frame buffer is assumed. When reading into a FBuffer object, any previous contents are freed and the FBuffer object is configured to contain the rectangle of frame buffer data specified. When writing from a FBuffer object to the frame buffer, the rectangle limits must match the dimensions of the data held in the FBuffer object or the results are undefined. FBuffer objects hold frame buffer information in a device independent format, they may be read and written to any frame buffer.The current frame buffers for reading and writing (drawing) are determined by the VGL_FRONTBUFFERREADMODE and VGL_FRONTBUFFERDRAWMODE modes specified with the vgl_DrawFunSetMode function.
The reading and writing operations supported by these functions are relatively slow compared to the functions which operate on Pixelmap objects. Use Pixelmap objects to maximize performance when entire an frame buffer and/or zbuffer are to be simply written to and read from buffer memory.
DrawFun
NAME
vgl_DrawFunFlush - flush all pending graphics operationsC SPECIFICATION
void vgl_DrawFunFlush (vgl_DrawFun *drawfun)
INPUT ARGUMENTS
drawfun Pointer to DrawFun object.
OUTPUT ARGUMENTS
None
DESCRIPTION
Force any pending graphics operations to be completed, updating the frame buffer. This function should be called before waiting for user input which depends upon a generated image.
DrawFun
NAME
vgl_DrawFunGetFloat - return the value of a selected floating point parameterC SPECIFICATION
void vgl_DrawFunGetFloat (vgl_DrawFun *drawfun, Vint type, Vfloat params[])
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. type Specify the type of parameter to return =VGL_BACKCOLOR Return 3 RGB components of current back ground color. =VGL_COLOR Return 3 RGB components of current color. =VGL_DEPTHRANGE Return 2 values which are the current near and far settings of the depth range. =VGL_MAXDEPTHRANGE Return 2 values which are the maximum allowable near and far limits of the depth range =VGL_MAXWINDOWCENTIMETERS Return 2 values which are the horizontal and vertical sizes of display screen in centimeters =VGL_MODELVIEWMATRIX Return 16 values which are the elements of the current modelview matrix =VGL_POLYGONOFFSET Return 2 values which are the arguments input to PolygonOffset =VGL_PROJECTIONLIMITS Return 6 values which are the arguments input to either ProjOrtho or ProjFrustum =VGL_PROJECTIONMATRIX Return 16 values which are the elements of the current projection matrix =VGL_SECONDS Return current processor time used by current process in seconds. =VGL_SPECULARITY Return 2 values which are the current material specularity and shininess. =VGL_TRANS Return 1 value which is the current material transparency. =VGL_WINDOWCENTIMETERS Return 2 values which are the horizontal and vertical sizes of the current graphics window in centimeters
OUTPUT ARGUMENTS
params Pointer to floating point return values
ERRORS
VGL_ERROR_ENUM is generated if an improper type is specified.DESCRIPTION
This function returns floating point state parameters of an object. Use vgl_DrawFunGetInteger to return integer values and vgl_DrawFunGetMode to return the current modes.
DrawFun
NAME
vgl_DrawFunGetInteger - return the value of a selected integer parameterC SPECIFICATION
void vgl_DrawFunGetInteger (dvgl_DrawFun *drawfun, Vint type, Vint params[])
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. type Specify the type of parameter to return =VGL_BACKCOLORINDEX Return 1 value which is the current back ground color index. =VGL_BITMAPINDEX Return 1 value which is the current bitmap index =VGL_BITMAPNUMBER Return 1 value which is the number of defined bitmaps. =VGL_BITMAPINDICES Return values of all defined bitmap indices =VGL_BUFFER_SUPPORTED Return 1 if Buffer primitives supported =VGL_COLORINDEX Return 1 value which is the current color index. =VGL_DEPTHPEEL_PIXELS Return number of pixels touched by last depth peel. =VGL_DEVICESIZE Return 2 values which are the horizontal and vertical sizes of the device driver in pixels. This value may differ from the window size. =VGL_DOUBLEBUFFER Return 1 boolean value which indicates whether the frame buffer supports double buffering =VGL_LINESTYLE Return 1 value which is the current line style. =VGL_LINEWIDTH Return 1 value which is the current line width. =VGL_MAXWINDOWSIZE Return 2 values which are the horizontal and vertical sizes of display screen in pixels =VGL_MULTISAMPLE_SUPPORTED Return 1 if multisample supported =VGL_NUMRASTERPRIM Return 1 value which is the number of rasterized primitives =VGL_NUMVECTORPRIM Return 1 value which is the number of vector primitives =VGL_OIT_COUNTERS Return 2 values which are the maximum and current fragment counts. =VGL_OIT_MEMORYFAIL Return 1 value which is a flag indicating GPU memory failure =VGL_PLANEDEPTH Return 1 value which is the number of planes in the frame buffer. =VGL_POINTSIZE Return 1 value which is the current point size. =VGL_POINTSTYLE Return 1 value which is the current point style. =VGL_POLYGONMODE Return 1 value which is the argument input to PolygonMode =VGL_PROJECTIONTYPE Return 1 value which is the current projection type. The value is zero (VGL_PROJORTHO) if the projection is orthographic and unity (VGL_PROJFRUSTUM) if the projection is perspective. =VGL_RASFONTINDEX Return 1 value which is the current raster font index =VGL_RASFONTNUMBER Return 1 value which is the number of defined raster fonts. =VGL_RASFONTINDICES Return values of all defined raster font indices =VGL_RASFONT_METRICS Return 4 values which are the current raster font width, height, offset and base. =VGL_RASFONT_SIZE Return 1 value which is the current raster font size. =VGL_RASFONT_SPACING Return 1 value which is the current raster font spacing. =VGL_RENDERMODE Return 1 value which is the current rendermode set by the Render drawing function. =VGL_SHADER_SUPPORTED Return 1 if shader supported. =VGL_TEXTUREINDEX Return 1 value which is the current texture map index =VGL_TEXTURENUMBER Return 1 value which is the number of defined texture maps =VGL_TEXTUREINDICES Return values of all defined texture map indices =VGL_TRANSINDEX Return 1 value which is the current transparency index. =VGL_TRUECOLOR Return 1 boolean value which indicates whether the frame buffer supports a true color visual =VGL_VIEWPORT Return 4 values which are the limits of the current viewport in pixels. =VGL_WINDOWSIZE Return 2 values which are the horizontal and vertical sizes of the current graphics window in pixels =VGL_WINDOWORIGIN Return 2 values which are the horizontal and vertical position of the current graphics window in pixels in screen coordinates =VGL_WINDOWSYSTEM Return 1 boolean value which indicates whether the frame buffer is associated with a graphics window. =VGL_ZBUFFERDEPTH Return 1 value which is the number of planes in the z buffer.
OUTPUT ARGUMENTS
params Pointer to integer return values
ERRORS
VGL_ERROR_ENUM is generated if an improper type is specified.DESCRIPTION
This function returns integer state parameters of an object. Use vgl_DrawFunGetFloat to return floating point values and vgl_DrawFunGetMode to return the current frame buffer modes.
DrawFun
NAME
vgl_DrawFunGetString - return the value of a selected string parameterC SPECIFICATION
void vgl_DrawFunGetString (vgl_DrawFun *drawfun, Vint type, Vchar params[])
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. type Specify the type of parameter to return =VGL_RENDERER Return renderer string. This is a parameter which is normally specific to a hardware platform. =VGL_VENDOR Return vendor string. This is the company responsible for the underlying graphics interface. =VGL_VERSION Return version string of underlying graphics interface. =VGL_SHADER VERSION Return version string of underlying shader language.
OUTPUT ARGUMENTS
params Pointer to character buffer into which to copy a string. Reserve at least a 128 character buffer including terminating NULL character.
ERRORS
VGL_ERROR_ENUM is generated if an improper type is specified.DESCRIPTION
This function copies string parameters of an object into character buffer params. Software renderers return a vendor string of "VKI" and a renderer string of the VglTools module name.
DrawFun
NAME
vgl_DrawFunLight - define a light sourceC SPECIFICATION
void vgl_DrawFunLight (vgl_DrawFun *drawfun, Vint index, Vint type, Vfloat c[3], Vfloat x[3])
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. index Light source index, 0 <= index <= 7; type Light source type =VGL_LIGHT_AMBIENT Ambient light =VGL_LIGHT_DISTANT Distant light c Light color RGB values x World coordinate position of light source
OUTPUT ARGUMENTS
None
DESCRIPTION
Define an ambient or distant light source. If an ambient light source is defined then the light position, x is ignored. Light source positions are transformed by the current modelview matrix. When defined, light sources are assumed to be switched on (see vgl_DrawFunSetSwitch).
DrawFun
NAME
vgl_DrawFunLightModel - define global lighting model parametersC SPECIFICATION
void vgl_DrawFunLightModel (vgl_DrawFun *drawfun, Vint localviewer, Vfloat koffset, Vfloat krate)
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. localviewer Local viewer flag =VGL_OFF Turn localviewer off =VGL_ON Turn localviewer on koffset Light attenuation offset krate Light attenuation rate
OUTPUT ARGUMENTS
None
DESCRIPTION
Define global lighting model parameters. Initially localviewer is off, a distant viewer is assumed. Currently this function is ignored in VglTools.
DrawFun
NAME
vgl_DrawFunLineStyle,vgl_DrawFunLineWidth - set line drawing attributesC SPECIFICATION
void vgl_DrawFunLineStyle (vgl_DrawFun *drawfun, Vint linestyle) void vgl_DrawFunLineWidth (vgl_DrawFun *drawfun, Vint linewidth)
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. linestyle Current line style =VGL_LINESTYLE_SOLID Solid =VGL_LINESTYLE_DOT Dotted =VGL_LINESTYLE_DASH Dashed =VGL_LINESTYLE_DOTDASH Dot dash =VGL_LINESTYLE_LDASH Long dash =VGL_LINESTYLE_DOTLDASH Dot long dash =VGL_LINESTYLE_DOTDOT Dot dot =VGL_LINESTYLE_DOTDOTLDASH Dot dot long dash =VGL_LINESTYLE_LLDASH Long long dash linewidth Current line width, 1 <= linewidth
OUTPUT ARGUMENTS
None
DESCRIPTION
Set the current line style and line width. Initially the line style is VGL_LINESTYLE_SOLID and the line width is unity. A linewidth of less than or equal to zero will be set to unity. The line styles can be scaled by an integer factor using vgl_DrawFunSetMode with mode VGL_LINESTYLEFACTOR. The line styles are represented below:VGL_LINESTYLE_DOT xx xx xx xx VGL_LINESTYLE_DASH xxxx xxxx xxxx xxxx VGL_LINESTYLE_DOTDASH xxxx xx xxxx xx VGL_LINESTYLE_LDASH xxxxxx xxxxxx xxxxxx xxxxxx VGL_LINESTYLE_DOTLDASH xxxxxx xx xxxxxx xx VGL_LINESTYLE_DOTDOT xx xx xx xx xx xx xx xx VGL_LINESTYLE_DOTDOTLDASH xxxxxx xx xx xxxxxx xx xx VGL_LINESTYLE_LLDASH xxxxxxxxxxxx xxxxxxxxxxxx
DrawFun
NAME
vgl_DrawFunOpenWindow - open a graphics window or fileC SPECIFICATION
void vgl_DrawFunOpenWindow (vgl_DrawFun *drawfun, const Vchar title[])
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. title Graphics window title or host file name
OUTPUT ARGUMENTS
None
ERRORS
VGL_ERROR_OPERATION is generated if the window has already been opened up or connected to using vgl_DrawFunOpenWindow or vgl_DrawFunConnectWindow.DESCRIPTION
Open a graphics window or host file for an object to render or write to. A graphics window will be opened with the position and visual attributes previously set by vgl_DrawFunConfigureWindow or vgl_DrawFunPositionWindow and vgl_DrawFunVisualWindow and be given a window title specified by title. A graphics device object which requires a host file for rendering will create a file with pathname title and assume a software frame buffer with dimensions and visual characteristics specified by vgl_DrawFunParentWindow, vgl_DrawFunConfigureWindow or vgl_DrawFunPositionWindow and vgl_DrawFunVisualWindow. Use vgl_DrawFunCloseWindow to close the window or file.
DrawFun
NAME
vgl_DrawFunParentWindow - specify parent windowC SPECIFICATION
void vgl_DrawFunParentWindow (vgl_DrawFun *drawfun, Vword parent)
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. parent Window id of parent window, if X window. Window handle of parent window, if Microsoft Windows window.
OUTPUT ARGUMENTS
None
ERRORS
VGL_ERROR_OPERATION is generated if a parent window has already been specified or the window has already been opened up or connected to using vgl_DrawFunOpenWindow or vgl_DrawFunConnectWindow.DESCRIPTION
Set the window id of the window to be used as the parent window for a graphics interface device object. This function should be called before vgl_DrawFunOpenWindow. By default the parent window is the root window of the display.
DrawFun
NAME
vgl_DrawFunPositionWindow - initial window size and positionC SPECIFICATION
void vgl_DrawFunPositionWindow (vgl_DrawFun *drawfun, Vint xorig, Vint yorig, Vint xsize, Vint ysize)
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. xorig Horizontal origin of window yorig Vertical origin of window xsize Horizontal size of window ysize Vertical size of window
OUTPUT ARGUMENTS
None
DESCRIPTION
Request an initial position and size of a window associated with a graphics interface device object. The window origin is the lower left hand corner of the drawable portion (client area) of the window. The drawable portion of the window does not include the window borders or title bar. Similarly, the window size is in reference to the size in pixels of the drawable portion of the window. Use vgl_DrawFunConfigureWindow to change the window size and/or origin after it is opened.
DrawFun
NAME
vgl_DrawFunPixelmapCreate,vgl_DrawFunPixelmapDestroy - begin and end a PixelmapC SPECIFICATION
void vgl_DrawFunPixelmapCreate (vgl_DrawFun *drawfun, vgl_Pixelmap *pixelmap) void vgl_DrawFunPixelmapDestroy (vgl_DrawFun *drawfun, vgl_Pixelmap *pixelmap)
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. pixelmap Pointer to Pixelmap object.
OUTPUT ARGUMENTS
None
DESCRIPTION
Create and destroy the memory used by a Pixelmap object to store frame buffer or zbuffer contents. To maximize the block transfer rate of buffer contents to and from Pixelmap memory, the internal data structures in the Pixelmap object must be configured in a device dependent way. This means that the contents of Pixelmap objects may not be transferred to the buffer of a device other than that on which it was created. Use vgl_DrawFunPixelmapRead and vgl_DrawFunPixelmapWrite to perform the actual transfer of buffer contents.
DrawFun
NAME
vgl_DrawFunPixelmapRead,vgl_DrawFunPixelmapWrite - read and write to PixelmapC SPECIFICATION
void vgl_DrawFunPixelmapRead (vgl_DrawFun *drawfun, vgl_Pixelmap *pixelmap) vgl_Pixelmap *pixelmap) void vgl_DrawFunPixelmapWrite (vgl_DrawFun *drawfun, vgl_Pixelmap *pixelmap)
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. pixelmap Pointer to Pixelmap object.
OUTPUT ARGUMENTS
None
DESCRIPTION
Read and write buffer contents to a Pixelmap object. Use vgl_DrawFunPixelmapRead to read frame buffer contents into a Pixelmap object. Use vgl_DrawFunPixelmapWrite to write the contents of a Pixelmap object to a buffer. Pixelmap objects are optimized for block transfer efficiency, only entire an frame buffer or zbuffer may be read and written.The current front or back device buffers for reading and writing (drawing) are determined by the VGL_FRONTBUFFERREADMODE and VGL_FRONTBUFFERDRAWMODE modes specified with the vgl_DrawFunSetMode function.
DrawFun
NAME
vgl_DrawFunPointSize - set point sizeC SPECIFICATION
void vgl_DrawFunPointSize (vgl_DrawFun *drawfun, Vint pointsize)
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. pointsize Current point size, 1 <= pointsize <= 8
OUTPUT ARGUMENTS
None
DESCRIPTION
Set the current point size. The application of the point size depends upon the point style set using vgl_DrawFunPointStyle. By default, points are drawn as square regions of pixels. The pointsize indicates the number of pixels along an edge of the square. If the point style is a device sized sphere, the point size is the diameter of the sphere. A unit point size draws a single pixel for each point. Initially the point size is unity.
DrawFun
NAME
vgl_DrawFunPointStyle - set point styleC SPECIFICATION
void vgl_DrawFunPointStyle (vgl_DrawFun *drawfun, Vint pointstyle)
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. pointstyle Current point style. =VGL_POINTSTYLE_DOT Square dot =VGL_POINTSTYLE_CIRCLE Device sized filled circle =VGL_POINTSTYLE_SPHERE Device sized sphere =VGL_POINTSTYLE_SPHEREWORLD World sized sphere =VGL_POINTSTYLE_UINT Unsigned int center register =VGL_POINTSTYLE_UINTLL Unsigned int lower left register =VGL_POINTSTYLE_PLUS9 Plus =VGL_POINTSTYLE_ASTERISK9 Asterisk =VGL_POINTSTYLE_CROSS9 Cross =VGL_POINTSTYLE_BOX9 Box =VGL_POINTSTYLE_CROSSBOX9 CrossBox =VGL_POINTSTYLE_CIRCLE9 Circle =VGL_POINTSTYLE_TRIUP9 Upward Triangle =VGL_POINTSTYLE_TRIRIGHT9 Rightward Triangle =VGL_POINTSTYLE_TRIDOWN9 Downward Triangle =VGL_POINTSTYLE_TRILEFT9 Leftward Triangle =VGL_POINTSTYLE_DIAMOND9 Diamond =VGL_POINTSTYLE_TBOXBITS9 T Box with bits =VGL_POINTSTYLE_TDIAMONDBITS9 T Diamond with bits =VGL_POINTSTYLE_CROSSCIRCLE9 Crossed Circle =VGL_POINTSTYLE_PLUSCIRCLE9 Plussed Circle
OUTPUT ARGUMENTS
None
DESCRIPTION
Set the current point style. The sizing of each point style depends upon the style. All styles except VGL_POINTSTYLE_DOT require shaders to be enabled.The VGL_POINTSTYLE_DOT style draws a device sized square reqion of pixels The width and height of the square in pixels is set by the current point size set by vgl_DrawFunPointSize.
The VGL_POINTSTYLE_CIRCLE style draws a device sized filled circle The diameter of the circle in pixels is set by the current point size set by vgl_DrawFunPointSize.
The VGL_POINTSTYLE_SPHERE style draws a device sized sphere. The diameter of the sphere in pixels is set by the current point size set by vgl_DrawFunPointSize.
The VGL_POINTSTYLE_SPHEREWORLD style draws a world sized sphere. The diameter of the sphere is set using the function vgl_DrawFunSetFactors with type VGL_WORLDSIZE.
The VGL_POINTSTYLE_UINT and VGL_POINTSTYLE_UINTLL styles draw unsigned integers. The unsigned integers are installed in the vertex data vectors in vgl_DrawFunPolyPointArray and vgl_DrawFunPolyPointBuffer. The size is constant.
The VGL_POINTSTYLE_PLUS9 through VGL_POINTSTYLE_PLUSCIRCLE9 styles draw 9 by 9 pixel bitmaps. The size is constant.
DrawFun
NAME
vgl_DrawFunInitBuffer - create a vertex bufferC SPECIFICATION
void vgl_DrawFunInitBuffer (vgl_DrawFun *drawfun, Vint size, Vint *vboid)
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. size Size of vertex buffer in bytes.
OUTPUT ARGUMENTS
vboid Vertex buffer identifier
DESCRIPTION
Create a vertex buffer of length size bytes. The vertex buffer identifier, vboid, is returned. If the creation of the vertex buffer fails, then a vertex buffer identifier of zero is returned, otherwise the a positive integer is returned.
DrawFun
NAME
vgl_DrawFunTermBuffer - delete a vertex bufferC SPECIFICATION
void vgl_DrawFunTermBuffer (vgl_DrawFun *drawfun, Vint vboid)
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. vboid Vertex buffer identifier
OUTPUT ARGUMENTS
None
DESCRIPTION
Delete vertex buffer vboid.
DrawFun
NAME
vgl_DrawFunCopyBuffer - copy vertex data to a vertex bufferC SPECIFICATION
void vgl_DrawFunCopyBuffer (vgl_DrawFun *drawfun, Vint vboid, Vint npts, Vfloat x[][3], Vint cflag, void *c, Vint vflag, void *v, Vint tflag, Vfloat *t, Vint dflag, Vfloat *d, Vint *offset)
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. vboid Vertex buffer identifier npts Number of vertex points x Vertex world coordinates cflag Vertex color flag =0 No color =VGL_COLOR_4B RGBA unsigned char =VGL_COLOR_3F RGB float =VGL_COLOR_4F RGBA float c Vertex colors vflag Vertex normal flag =0 No normals =VGL_NORMAL_4B signed char =VGL_NORMAL_3F float v Vertex normals tflag Vertex texture coordinate flag =0 No texture coordinates =VGL_1DTEXTURE 1-component texture =VGL_2DTEXTURE 2-component texture t Vertex texture coordinates dflag Vertex data flag, number of data components per vertex d Vertex data
OUTPUT ARGUMENTS
offset Offset into vertex buffer
DESCRIPTION
Copy vertex data at npts vertices from client memory to vertex buffer vboid. The vertex data consists of world coordinate locations x with optional color, c, normals, v, texture coordinates, t and data, d. An offset in the vboid is returned. If there is insufficient room in the vertex buffer to contain the data to be copied, an offset of -1 is returned.
DrawFun
NAME
vgl_DrawFunPolygon,vgl_DrawFunPolygonColor - draw polygonsC SPECIFICATION
void vgl_DrawFunPolygon (vgl_DrawFun *drawfun, Vint polygontype, Vint npts, Vfloat x[][3], Vint vflag, Vfloat v[]) void vgl_DrawFunPolygonColor (vgl_DrawFun *drawfun, Vint polygontype, Vint npts, Vfloat x[][3], Vfloat c[][3], Vint vflag, Vfloat v[])
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. polygontype Type of polygon to draw =VGL_POLYGON Draw a single polygon =VGL_QUADS Draw a set of quadrilaterals =VGL_TRIANGLES Draw a set of triangles =VGL_TRISTRIP Draw a triangle strip =VGL_TRIFAN Draw a triangle fan npts Number of vertices in polygon primitive x Vertex world coordinates c Vertex color RGB values vflag Normal vector and vertex texture coordinate flag. OR the following flags. A zero means no normals or texture coordinates are present. =VGL_NOSHADE No normals present =VGL_FLATSHADE Normals per tri, quad or poly =VGL_VERTEXSHADE Normals per vertex =VGL_1DTEXTURE 1 texture coordinate per vertex =VGL_2DTEXTURE 2 texture coordinates per vertex v Normal vectors and texture coordinates. Normal vectors for each facet or vertex appear first followed by texture coordinates for each vertex.
OUTPUT ARGUMENTS
None
DESCRIPTION
Draw filled polygons through npts vertices at world coordinate locations x. vgl_DrawFunPolygon draws polygons using the current color, vgl_DrawFunPolygonColor draws polygons specifying the color at each vertex. The current color is undefined after vgl_DrawFunPolygonColor. Polygons are drawn using the current transparency and specularity, All polygons must be convex to ensure proper rendering. A triangle strip draws npts-2 triangles. If flat shading is used with a triangle strip, npts-2 normals are required, one for each triangle drawn.If no normals are present and lighting is enabled, the normals used for lighting effects are undefined. Lighting should be diabled in this case.
If texture coordinates are specified then 1 or 2 texture coordinates are required for each vertex. If no texture coordinates are specified while texture mapping is enabled, zeros are assumed.
DrawFun
NAME
vgl_DrawFunPolyPointArray,vgl_DrawFunPolyLineArray,vgl_DrawFunPolygonArray,vgl_DrawFunPolyArray - draw arrays of primitivesC SPECIFICATION
void vgl_DrawFunPolyPointArray (vgl_DrawFun *drawfun, Vint npts, Vfloat x[][3], Vint cflag, void *c, Vint vflag, void *v, Vint tflag, Vfloat *t, Vint dflag, Vfloat *d) void vgl_DrawFunPolyLineArray (vgl_DrawFun *drawfun, Vint polylinetype, Vint npts, Vfloat x[][3], Vint cflag, void *c, Vint vflag, void *v, Vint tflag, Vfloat *t, Vint dflag, Vfloat *d) void vgl_DrawFunPolygonArray (vgl_DrawFun *drawfun, Vint polygontype, Vint npts, Vfloat x[][3], Vint cflag, void *c, Vint vflag, void *v, Vint tflag, Vfloat *t, Vint dflag, Vfloat *d) void vgl_DrawFunPolyArray (vgl_DrawFun *drawfun, Vint polytype, Vint npts, Vfloat x[][3], Vint cflag, void *c, Vint vflag, void *v, Vint tflag, Vfloat *t, Vint dflag, Vfloat *d)
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. polytype Type of primitive to draw =VGL_POINTS Draw a series of points =VGL_LINESTRIP Draw a series of line segments =VGL_LINELOOP Draw a close loop of line segments =VGL_LINES Draw a set of unconnected lines =VGL_POLYGON Draw a single polygon =VGL_QUADS Draw a set of quadrilaterals =VGL_TRIANGLES Draw a set of triangles =VGL_TRISTRIP Draw a triangle strip =VGL_TRIFAN Draw a triangle fan npts Number of vertex points x Vertex world coordinates cflag Vertex color flag =0 No color =VGL_COLOR_4B RGBA unsigned char =VGL_COLOR_3F RGB float =VGL_COLOR_4F RGBA float c Vertex colors vflag Vertex normal flag =0 No normals =VGL_NORMAL_4B signed char =VGL_NORMAL_3F float v Vertex normals tflag Vertex texture coordinate flag =0 No texture coordinates =VGL_1DTEXTURE 1-component texture =VGL_2DTEXTURE 2-component texture t Vertex texture coordinates dflag Vertex data flag, number of data components per vertex d Vertex data
OUTPUT ARGUMENTS
None
DESCRIPTION
Draw polygons through npts vertices at world coordinate locations x with optional color, c, normals, v, texture coordinates, t and data, d. Points are drawn using the current point size and point style. Polylines are drawn using the current line style and line width. Polygons are drawn using the currently selected Bitmap.
DrawFun
NAME
vgl_DrawFunPolyPointBuffer,vgl_DrawFunPolyLineBuffer,vgl_DrawFunPolygonBuffer,vgl_DrawFunPolyBuffer - draw vertex buffers of primitivesC SPECIFICATION
void vgl_DrawFunPolyPointBuffer (vgl_DrawFun *drawfun, Vint vboid, Vint offset, Vint npts, Vint cflag, Vint vflag, Vint tflag, Vint dflag) void vgl_DrawFunPolyLineBuffer (vgl_DrawFun *drawfun, Vint vboid, Vint offset, Vint polylinetype, Vint npts, Vint cflag, Vint vflag, Vint tflag, Vint dflag) void vgl_DrawFunPolygonBuffer (vgl_DrawFun *drawfun, Vint vboid, Vint offset, Vint polygontype, Vint npts, Vint cflag, Vint vflag, Vint tflag, Vint dflag) void vgl_DrawFunPolyBuffer (vgl_DrawFun *drawfun, Vint vboid, Vint offset, Vint polytype, Vint npts, Vint cflag, Vint vflag, Vint tflag, Vint dflag)
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. vboid Vertex buffer identifier offset Offset into vertex buffer polytype Type of primitive to draw =VGL_POINTS Draw a series of points =VGL_LINESTRIP Draw a series of line segments =VGL_LINELOOP Draw a close loop of line segments =VGL_LINES Draw a set of unconnected lines =VGL_POLYGON Draw a single polygon =VGL_QUADS Draw a set of quadrilaterals =VGL_TRIANGLES Draw a set of triangles =VGL_TRISTRIP Draw a triangle strip =VGL_TRIFAN Draw a triangle fan npts Number of vertex points cflag Vertex color flag =0 No color =VGL_COLOR_4B RGBA unsigned char =VGL_COLOR_3F RGB float =VGL_COLOR_4F RGBA float vflag Vertex normal flag =0 No normals =VGL_NORMAL_4B signed char =VGL_NORMAL_3F float tflag Vertex texture coordinate flag =0 No texture coordinates =VGL_1DTEXTURE 1-component texture =VGL_2DTEXTURE 2-component texture dflag Vertex data flag, number of data components per vertex
OUTPUT ARGUMENTS
None
DESCRIPTION
Draw polygons through npts vertices using graphics primitive data stored in vertex buffer vboid starting at offset with optional color, normals, texture coordinates and data. The vertex buffer must have been previously created using vgl_DrawFunInitBuffer and the graphics data copied to vertex buffer memory using vgl_DrawFunCopyBuffer. Points are drawn using the current point size and point style. Polylines are drawn using the current line style and line width. Polygons are drawn using the currently selected Bitmap.
DrawFun
NAME
vgl_DrawFunPolyElemArray - draw indexed arrays of primitivesC SPECIFICATION
void vgl_DrawFunPolyElemArray (vgl_DrawFun *drawfun, Vint polytype, Vint npts, Vuint ix[], Vfloat x[][3], Vint cflag, void *c, Vint vflag, void *v, Vint tflag, Vfloat *t, Vint dflag, Vfloat *d)
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. polytype Type of primitive to draw =VGL_POINTS Draw a series of points =VGL_LINESTRIP Draw a series of line segments =VGL_LINELOOP Draw a close loop of line segments =VGL_LINES Draw a set of unconnected lines =VGL_POLYGON Draw a single polygon =VGL_QUADS Draw a set of quadrilaterals =VGL_TRIANGLES Draw a set of triangles =VGL_TRISTRIP Draw a triangle strip =VGL_TRIFAN Draw a triangle fan npts Number of vertex points ix Primitive vertex npts indices x Vertex world coordinates cflag Vertex color flag =0 No color =VGL_COLOR_4B RGBA unsigned char =VGL_COLOR_3F RGB float =VGL_COLOR_4F RGBA float c Vertex colors vflag Vertex normal flag =0 No normals =VGL_NORMAL_4B signed char =VGL_NORMAL_3F float v Vertex normals tflag Vertex texture coordinate flag =0 No texture coordinates =VGL_1DTEXTURE 1-component texture =VGL_2DTEXTURE 2-component texture t Vertex texture coordinates dflag Vertex data flag, number of data components per vertex d Vertex data
OUTPUT ARGUMENTS
None
DESCRIPTION
Draw primitives through npts vertices with vertex attributes referenced in the ix index array. Each zero based index references vertex attributes in world coordinate locations x with optional color, c, normals, v, texture coordinates, t and data, d. Points are drawn using the current point size and point style. Polylines are drawn using the current line style and line width. Polygons are drawn using the currently selected Bitmap.
DrawFun
NAME
vgl_DrawFunPolyElemBuffer - draw indexed vertex buffers of primitivesC SPECIFICATION
void vgl_DrawFunPolyElemBuffer (vgl_DrawFun *drawfun, Vint elemvboid, Vint elemoffset, Vint vboid, Vint offset, Vint polytype, Vint npts, Vint cflag, Vint vflag, Vint tflag, Vint dflag)
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. elemvboid Index Vertex buffer identifier elemoffset Offset into index vertex buffer vboid Vertex buffer identifier offset Offset into vertex buffer polytype Type of primitive to draw =VGL_POINTS Draw a series of points =VGL_LINESTRIP Draw a series of line segments =VGL_LINELOOP Draw a close loop of line segments =VGL_LINES Draw a set of unconnected lines =VGL_POLYGON Draw a single polygon =VGL_QUADS Draw a set of quadrilaterals =VGL_TRIANGLES Draw a set of triangles =VGL_TRISTRIP Draw a triangle strip =VGL_TRIFAN Draw a triangle fan npts Number of vertex points cflag Vertex color flag =0 No color =VGL_COLOR_4B RGBA unsigned char =VGL_COLOR_3F RGB float =VGL_COLOR_4F RGBA float vflag Vertex normal flag =0 No normals =VGL_NORMAL_4B signed char =VGL_NORMAL_3F float tflag Vertex texture coordinate flag =0 No texture coordinates =VGL_1DTEXTURE 1-component texture =VGL_2DTEXTURE 2-component texture dflag Vertex data flag, number of data components per vertex
OUTPUT ARGUMENTS
None
DESCRIPTION
Draw primitives through npts vertices with vertex attributes referenced in the elemvboid index buffer starting at elemoffset. Each zero based index references vertex attributes stored in vertex buffer vboid starting at offset with optional color, normals, texture coordinates and data. The vertex buffer must have been previously created using vgl_DrawFunInitBuffer and the graphics data copied to vertex buffer memory using vgl_DrawFunCopyBuffer. Points are drawn using the current point size and point style. Polylines are drawn using the current line style and line width. Polygons are drawn using the currently selected Bitmap.
DrawFun
NAME
vgl_DrawFunPolygonData - draw a data polygonC SPECIFICATION
void vgl_DrawFunPolygonData (vgl_DrawFun *drawfun, Vint polygontype, Vint npts, Vfloat x[][3], Vint nrws, Vfloat d[], Vint vflag, Vfloat v[])
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. polygontype Type of polygon to draw =VGL_POLYGON Draw a single polygon =VGL_POLYHEDRON Draw a single polyhedron =VGL_QUADS Draw a set of quadrilaterals =VGL_TRIANGLES Draw a set of triangles =VGL_TRISTRIP Draw a triangle strip =VGL_TRIFAN Draw a triangle fan npts Number of vertices in polygon primitive x Vertex world coordinates nrws Number of values in d vector d Vector of data values vflag Normal vector and vertex texture coordinate flag. OR the following flags. A zero means no normals or texture coordinates are present. =VGL_NOSHADE No normals present =VGL_FLATSHADE Normals per tri, quad or poly =VGL_VERTEXSHADE Normals per vertex =VGL_1DTEXTURE 1 texture coordinate per vertex =VGL_2DTEXTURE 2 texture coordinates per vertex v Normal vectors and texture coordinates. Normal vectors for each facet or vertex appear first followed by texture coordinates for each vertex.
OUTPUT ARGUMENTS
None
DESCRIPTION
Draw filled polygons through npts vertices at world coordinate locations x. All polygons must be convex to ensure proper rendering. The number of data values, nrws must be in the interval [1,16]. vgl_DrawFunPolygonData is used by the DataBuf module. VglTools graphics device interface modules do not support vgl_DrawFunPolygonData. A triangle strip draws npts-2 triangles. If flat shading is used with a triangle strip, npts-2 normals are required, one for each triangle drawn. A polyhedron must consist of npts = 4, 5, 6 or 8 corresponding to a tetrahedron, pyramid, pentahedron or hexahedron respectively.If flat shading is used with a polyhedron a single normal is required. If no normals are present and lighting is enabled, the normals used for lighting effects are undefined. Lighting should be diabled in this case.
If texture coordinates are specified then 1 or 2 texture coordinates are required for each vertex. If no texture coordinates are specified while texture mapping is enabled, zeros are assumed.
DrawFun
NAME
vgl_DrawFunPolygonDC - draw a device oriented polygonC SPECIFICATION
void vgl_DrawFunPolygonDC (vgl_DrawFun *drawfun, Vint polygontype, Vint npts, Vfloat x[3], Vint dc[][3])
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. polygontype Type of polygon to draw =VGL_POLYGON Draw a single polygon =VGL_QUADS Draw a set of quadrilaterals =VGL_TRIANGLES Draw a set of triangles =VGL_TRISTRIP Draw a triangle strip =VGL_TRIFAN Draw a triangle fan npts Number of vertices in polygon primitive x World coordinate anchor point dc Vertex device coordinate offsets
OUTPUT ARGUMENTS
None
DESCRIPTION
Draw filled polygons through npts vertices with dc device coordinate offsets from anchor point x. vgl_DrawFunPolygonDC draws polygons which are rendered relative to a world coordinate anchor point perpendicular to the viewing direction. An offset in device coordinates is specified for each polygon vertex. The device coordinate z component is ignored. Polygons are drawn using the current color, transparency and specularity, All polygons must be convex to ensure proper rendering. A triangle strip draws npts-2 triangles.
DrawFun
NAME
vgl_DrawFunPolygonMode - specify polygon drawing modeC SPECIFICATION
void vgl_DrawFunPolygonMode (vgl_DrawFun *drawfun, Vint rend)
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. rend Number of dimensions to reduce polygon rendering =0 Draw filled polygons =1 Draw polygons as lines =2 Draw polygons as points at vertices =3 Do not draw polygons
OUTPUT ARGUMENTS
None
DESCRIPTION
Specify the drawing mode for polygon primitives. The input parameter, rend, specifies the number of dimensions to reduce the rendering of polygons. Note that if rend = 3, then polygon primitives are not drawn. By default, rend = 0.
DrawFun
NAME
vgl_DrawFunPolygonOffset - specify polygon depth adjustmentC SPECIFICATION
void vgl_DrawFunPolygonOffset (vgl_DrawFun *drawfun, Vfloat factor, Vfloat units)
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. factor Polygon depth slope factor. units Polygon depth constant offset
OUTPUT ARGUMENTS
None
DESCRIPTION
Control the depth adjustment applied to polygons when VGL_POLYGONDEPTHMODE is enabled. The factor is a depth adjustment which is proportional to the slope of the polygon in the depth direction. The units is a constant depth unit, multiplied by an implementation dependent value, added to the depth coordinate of each polygon pixel. The default factor is 1., the default bias is 1.
DrawFun
NAME
vgl_DrawFunPolyLine,vgl_DrawFunPolyLineColor - draw polylinesC SPECIFICATION
void vgl_DrawFunPolyLine (vgl_DrawFun *drawfun, Vint polylinetype, Vint npts, Vfloat x[][3]) void vgl_DrawFunPolyLineColor (vgl_DrawFun *drawfun, Vint polylinetype, Vint npts, Vfloat x[][3], Vfloat c[][3])
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. polylinetype Type of polyline to draw =VGL_LINESTRIP Draw a series of line segments =VGL_LINELOOP Draw a close loop of line segments =VGL_LINES Draw a set of unconnected lines npts Number of vertices in polyline primitive x Vertex world coordinates c Vertex color RGB values
OUTPUT ARGUMENTS
None
DESCRIPTION
Draw polylines through npts vertices at world coordinate locations x. vgl_DrawFunPolyLine draws polylines using the current color, vgl_DrawFunPolyLineColor draws polylines specifying the color at each vertex. The current color is undefined after vgl_DrawFunPolyLineColor. Polylines are drawn using the current line style and line width. VGL_LINESTRIP draws a connected set of straight line segments from the first vertex through each intermediate vertex to the last vertex in order. VGL_LINELOOP adds an additional line segment connecting the last vertex back to the first vertex. VGL_LINES draws an unconnected set of lines between pairs of vertices.
DrawFun
NAME
vgl_DrawFunPolyLineData - draw a data polylineC SPECIFICATION
void vgl_DrawFunPolyLineData (vgl_DrawFun *drawfun, Vint polylinetype, Vint npts, Vfloat x[][3], Vint nrws, Vfloat d[])
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. polylinetype Type of polyline to draw =VGL_LINESTRIP Draw a series of line segments =VGL_LINELOOP Draw a close loop of line segments =VGL_LINES Draw a set of unconnected lines npts Number of vertices in polyline primitive x Vertex world coordinates nrws Number of values in d vector d Vector of data values
OUTPUT ARGUMENTS
None
DESCRIPTION
Draw polylines through npts vertices at world coordinate locations x. The number of data values, nrws must be in the interval [1,16]. vgl_DrawFunPolyLineData is used by the DataBuf module. VglTools graphics device interface modules do not support vgl_DrawFunPolyLineData.DrawFun
NAME
vgl_DrawFunPolyLineDC - draw a device oriented polylineC SPECIFICATION
void vgl_DrawFunPolyLineDC (vgl_DrawFun *drawfun, Vint polylinetype, Vint npts, Vfloat x[3], Vint dc[][3])
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. polylinetype Type of polyline to draw =VGL_LINESTRIP Draw a series of line segments =VGL_LINELOOP Draw a close loop of line segments =VGL_LINES Draw a set of unconnected lines npts Number of vertices in polyline primitive x World coordinate anchor point dc Vertex device coordinate offsets
OUTPUT ARGUMENTS
None
DESCRIPTION
Draw polylines through npts vertices with dc device coordinate offsets from anchor point x. vgl_DrawFunPolyLineDC draws polylines which are rendered relative to a world coordinate anchor point perpendicular to the viewing direction. An offset in device coordinates is specified for each polyline vertex. The device coordinate z component is ignored. Polylines are drawn using the current line style and line width.
DrawFun
NAME
vgl_DrawFunPolyPoint,vgl_DrawFunPolyPointColor - draw pointsC SPECIFICATION
void vgl_DrawFunPolyPoint (vgl_DrawFun *drawfun, Vint npts, Vfloat x[][3]) void vgl_DrawFunPolyPointColor (vgl_DrawFun *drawfun, Vint npts, Vfloat x[][3], Vfloat c[][3])
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. npts Number of vertex points x Vertex world coordinates c Vertex color RGB values
OUTPUT ARGUMENTS
None
DESCRIPTION
Draw points at npts vertices at world coordinate locations x. vgl_DrawFunPolyPoint draws points using the current color, vgl_DrawFunPolyPointColor draws points specifying the color at each vertex. The current color is undefined after vgl_DrawFunPolyPointColor. Points are drawn using the current point size and point style.
DrawFun
NAME
vgl_DrawFunPolyPointData - draw data pointsC SPECIFICATION
void vgl_DrawFunPolyPointData (vgl_DrawFun *drawfun, Vint npts, Vfloat x[][3], Vint nrws, Vfloat d[])
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. npts Number of vertex points x Vertex world coordinates nrws Number of values in d vector d Vector of data values
OUTPUT ARGUMENTS
None
DESCRIPTION
Draw points at npts vertices at world coordinate locations x. The number of data values, nrws must be in the interval [1,16]. vgl_DrawFunPolyPointData is used by the DataBuf module. VglTools graphics device interface modules do not support vgl_DrawFunPolyPointData.DrawFun
NAME
vgl_DrawFunPolyPointDC - draw device oriented pointsC SPECIFICATION
void vgl_DrawFunPolyPointDC (vgl_DrawFun *drawfun, Vint npts, Vfloat x[3], Vint dc[][3])
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. npts Number of vertex points x World coordinate anchor point dc Vertex device coordinate offsets
OUTPUT ARGUMENTS
None
DESCRIPTION
Draw points at npts vertices with dc device coordinate offsets from anchor point x. vgl_DrawFunPolyPointDC draws points which are rendered relative to a world coordinate anchor point perpendicular to the viewing direction. An offset in device coordinates is specified for each point vertex. The device coordinate z component is ignored. Points are drawn using the current point size.
DrawFun
NAME
vgl_DrawFunPollModifiers - poll shift and control button stateC SPECIFICATION
void vgl_DrawFunPollModifiers (vgl_DrawFun *drawfun, Vint *cntl, Vint *shft)
INPUT ARGUMENTS
drawfun Pointer to DrawFun object.
OUTPUT ARGUMENTS
cntl Boolean value indicating Control key depressed shft Boolean value indicating Shift key depressed
DESCRIPTION
This function returns the current state of the Control and Shift modifier keys.DrawFun
NAME
vgl_DrawFunPollMouse - poll mouse location and button stateC SPECIFICATION
void vgl_DrawFunPollMouse (vgl_DrawFun *drawfun, Vint *px, Vint *py, Vint *but1, Vint *but2, Vint *but3)
INPUT ARGUMENTS
drawfun Pointer to DrawFun object.
OUTPUT ARGUMENTS
px,py Location of mouse in pixels but1 Boolean value indicating left mouse button depressed but2 Boolean value indicating middle mouse button depressed but3 Boolean value indicating right mouse button depressed
DESCRIPTION
This function returns the current state of the mouse.
DrawFun
NAME
vgl_DrawFunProjFrustum,vgl_DrawFunProjOrtho - define projection transformationC SPECIFICATION
void vgl_DrawFunProjFrustum (vgl_DrawFun *drawfun, Vfloat left, Vfloat right, Vfloat bottom, Vfloat top, Vfloat nearz, Vfloat farz) void vgl_DrawFunProjOrtho (vgl_DrawFun *drawfun, Vfloat left, Vfloat right, Vfloat bottom, Vfloat top, Vfloat nearz, Vfloat farz;
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. left,right Coordinates of left and right vertical clipping planes bottom,top Coordinates of bottom and top horizontal clipping planes nearz,farz Distances to near and far depth clipping planes
OUTPUT ARGUMENTS
None
DESCRIPTION
Define a projection transformation. The specified projection transformation replaces the current projection transformation. The points (left,bottom,-nearz) and (right,top,-farz) define the points on the near clipping plane that are mapped to the lower left and upper right corners of the current viewport (see vgl_DrawFunViewport). The value -farz specifies the location of the far clipping plane. Use vgl_DrawFunProjOrtho to define a parallel projection and vgl_DrawFunProjFrustum to define a perspective projection. If a perspective projection is specified, both nearz and farz must be positive. The projection transformation maps world coordinates which have been transformed by the current modelview matrix to a viewing volume in virtual device coordinates in the interval [-1.,1.]. The virtual device coordinates are mapped to device coordinates with the viewport transformation specified by vgl_DrawFunViewport and vgl_DrawFunDepthRange
DrawFun
NAME
vgl_DrawFunProjPop,vgl_DrawFunProjPush - pop and push projection matrix stackC SPECIFICATION
void vgl_DrawFunProjPop (vgl_DrawFun *drawfun) void vgl_DrawFunProjPush (vgl_DrawFun *drawfun)
INPUT ARGUMENTS
drawfun Pointer to DrawFun object.
OUTPUT ARGUMENTS
None
DESCRIPTION
Push and pop the projection matrix stack. vgl_DrawFunProjPush pushes the projection matrix stack, duplicating the top matrix. vgl_DrawFunProjPop pops the projection matrix stack, replacing the top matrix with the one below it. The projection matrix stack initially contains the identity matrix. The depth of the projection matrix stack is at least 32. Use vgl_DrawFunProjOrtho and vgl_DrawFunProjFrustum to load projection matrices onto the stack.
DrawFun
NAME
vgl_DrawFunProjLoad - load the current projection matrixC SPECIFICATION
void vgl_DrawFunProjLoad (vgl_DrawFun *drawfun, Vfloat m4x4[4][4])
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. m4x4 A 4 by 4 matrix stored in column major order
OUTPUT ARGUMENTS
None
DESCRIPTION
vgl_DrawFunProjLoad loads m4x4 onto the top of the projection matrix stack replacing the current matrix.
DrawFun
NAME
vgl_DrawFunQueryWindow - query for graphics window id or handleC SPECIFICATION
void vgl_DrawFunQueryWindow (vgl_DrawFun *drawfun, Vword *window)
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. window Window id, if X window. Window handle, if Microsoft Windows
OUTPUT ARGUMENTS
None
DESCRIPTION
Query for the current X Window id or Microsoft Windows window handle. to which a graphics interface object is currently rendering.
DrawFun
NAME
vgl_DrawFunRasFontDefine - define a raster font indexC SPECIFICATION
void vgl_DrawFunRasFontDefine (vgl_DrawFun *drawfun, Vint index, vgl_RasFont *rasfont)
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. index Raster font index, 1 <= index rasfont Pointer to RasFont object.
OUTPUT ARGUMENTS
None
ERRORS
VGL_ERROR_VALUE is generated if an index out of range is specified. VGL_ERROR_NULLOBJECT is generated if a NULL rasfont object is input.DESCRIPTION
Define a raster font. Use the RasFont module to build the raster font description. The RasFont module has a number of built-in fonts and also allows any X windows or Microsoft Windows font to be loaded. RasFont objects are copied by the graphics interface object. If a raster font had been previously defined for an index, the current definition replaces it. Use vgl_DrawFunRasFontSelect to select the current raster font index once it has been defined.
DrawFun
NAME
vgl_DrawFunRasFontSelect - select current raster fontC SPECIFICATION
void vgl_DrawFunRasFontSelect (vgl_DrawFun *drawfun, Vint index)
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. index Raster font index, 0 <= index
OUTPUT ARGUMENTS
None
ERRORS
VGL_ERROR_VALUE is generated if an index out of range is specified.DESCRIPTION
Select a current raster font index. This font remains in effect until vgl_DrawFunRasFontSelect is called again. Use vgl_DrawFunRasFontDefine to define a raster font index. Raster font index 0 is the default.
DrawFun
NAME
vgl_DrawFunReadQueue,vgl_DrawFunResetQueue,vgl_DrawFunTestQueue - event queueC SPECIFICATION
void vgl_DrawFunReadQueue (vgl_DrawFun *drawfun, Vint *dev, Vint *val) void vgl_DrawFunResetQueue (vgl_DrawFun *drawfun) void vgl_DrawFunTestQueue (vgl_DrawFun *drawfun, Vint *dev)
INPUT ARGUMENTS
drawfun Pointer to DrawFun object.
OUTPUT ARGUMENTS
dev Type of event token =VGL_EVENT_NONE No event =VGL_EVENT_UNRECOGNIZED Unrecognized event =VGL_EVENT_LEFTMOUSE Left mouse button =VGL_EVENT_MIDDLEMOUSE Middle mouse button =VGL_EVENT_RIGHTMOUSE Right mouse button =VGL_EVENT_KEYPRESS Key press =VGL_EVENT_KEYRELEASE Key release =VGL_EVENT_EXPOSE Exposure event val Value of event token.
DESCRIPTION
Perform event queue operations. vgl_DrawFunReadQueue reads and removes the topmost event from the event queue, returning the type of event, dev and its value, val. For mouse button events, val is 0 if the button was released and 1 if it it was depressed. For key events, val is the ASCII value of the key. For expose and unrecognized events, val is undefined. vgl_DrawFunResetQueue empties the event queue. vgl_DrawFunTestQueue returns the type of event from the top of the event queue without removing it.By default, the key and mouse button events are not recognized. Use vgl_DrawFunSetMode with VGL_EVENTQUEUEMODE enabled to recognize key and button events.
DrawFun
NAME
vgl_DrawFunRender - set rendering modeC SPECIFICATION
void vgl_DrawFunRender (vgl_DrawFun *drawfun, Vint rendermode)
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. rendermode Rendering mode =VGL_BUFFER_COLOR Render to off-screen buffer =VGL_EXTENT_WORLD World coordinate extent =VGL_EXTENT_EYE Eye coordinate extent =VGL_EXTENT_DEVICE Device coordinate extent =VGL_RENDER Normal rendering to frame buffer =VGL_SELECT Selection mode
OUTPUT ARGUMENTS
None
ERRORS
VGL_ERROR_ENUM is generated if an improper rendermode is input. VGL_ERROR_OPERATION is generated if selection mode is entered and vgl_DrawFunSelectBuffer has not been called.DESCRIPTION
Specify the rendering mode of the system. By default rendermode is VGL_RENDER. This is the normal rendering mode in which all graphics primitives are rasterized and written to the hardware frame buffer, etc.The VGL_BUFFER_COLOR rendering mode will render color to an off-screen frame buffer. For VGL_BUFFER_COLOR mode, use vgl_DrawFunBufferSize to specify an optional size of the off-screen frame buffer.
The VGL_EXTENT_EYE and VGL_EXTENT_DEVICE rendering modes specify that all subsequent graphics primitives are transformed and an extent box bounds calculation is performed in the appropriate coordinate system. The VGL_EXTENT_EYE mode performs the modelview transformation on input graphics primitives. The VGL_EXTENT_DEVICE mode performs the modelview, projection and viewport transformations on input graphics primitives. The VGL_EXTENT_WORLD mode does not perform any transformation on the graphics primitives to perform extent box bounds calculations. The frame buffer is not modified while the rendering mode is set to perform extent box calculations. Use vgl_DrawFunExtentQuery to return the extent box. If the extent box is queried before any primitives are drawn, then zeros are returned. Z-buffering is not performed in extent mode.
The VGL_SELECT rendering mode specifies that all subsequent graphics primitives are rasterized and checked to see if any portion of the primitive lies within the selection region specified by vgl_DrawFunSelectRegion. The selection buffers used to record hit information are specified by vgl_DrawFunSelectBuffer. Z-buffering is not performed in selection mode. The number of hits is set to zero. The index stack is initialized and a 0 is pushed onto the index stack. After the rendering mode is returned to VGL_RENDER, the number of hits may be returned using vgl_DrawFunSelectQuery.
DrawFun
NAME
vgl_DrawFunResize - resize a frame bufferC SPECIFICATION
void vgl_DrawFunResize (vgl_DrawFun *drawfun)
INPUT ARGUMENTS
drawfun Pointer to DrawFun object.
OUTPUT ARGUMENTS
None
DESCRIPTION
Inform an object to resize its frame buffer. For objects drawing to graphics windows, this function should be called in response to a window resize event to make sure that the underlying rendering mechanisms conform to the new window configuration. This function does not explicitly change the size of windows on the display device. Use vgl_DrawFunConfigureWindow to force a window to change its size.
DrawFun
NAME
vgl_DrawFunSelectBuffer - specify selection buffersC SPECIFICATION
void vgl_DrawFunSelectBuffer (vgl_DrawFun *drawfun, Vint size, Vint *indexlist, Vfloat *mindepth, Vfloat *maxdepth)
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. size Size of selection buffers indexlist Pointer to vector of hit indices. mindepth Pointer to vector of minimum depth of hit primitives. maxdepth Pointer to vector of maximum depth of hit primitives.
OUTPUT ARGUMENTS
None
ERRORS
VGL_ERROR_VALUE is generated if a size less than or equal to zero is specified.DESCRIPTION
Specify the size of and pointers to three buffers used to record hit information. Each buffer must be able to contain size hits. The indexlist buffer records the current DataIndex of each primitive which lies within the selection region. The minimum and maximum depth coordinate of each hit primitive within the selection region is recorded in the mindepth and maxdepth buffers. If mindepth is NULL then minimum depths are not recorded. If maxdepth is NULL then maximum depths are not recorded. This call must be made before selection mode is initiated using vgl_DrawFunRender.If a hit has been generated for the current DataIndex, no subsequent hits will be recorded until the value of the DataIndex changes. Use vgl_DrawFunSelectRegion to specify the selection region. Query for the number of hits using vgl_DrawFunSelectQuery.
DrawFun
NAME
vgl_DrawFunSelectQuery - query number of selection hitsC SPECIFICATION
void vgl_DrawFunSelectQuery (vgl_DrawFun *drawfun, Vint *hits)
INPUT ARGUMENTS
drawfun Pointer to DrawFun object.
OUTPUT ARGUMENTS
hits Number of hits recorded.
ERRORS
VGL_ERROR_OVERFLOW is generated if the number of hits exceeds size, specified by vgl_DrawFunSelectBuffer, during rendering.DESCRIPTION
Query for the number of hits which occur when the rendering mode is set to VGL_SELECT using vgl_DrawFunRender. The number of hits will not exceed the size of the hit buffers specified using vgl_DrawFunSelectBuffer.The number of hits is initialized to zero when the rendering mode is set to VGL_SELECT using vgl_DrawFunRender. The number of hits is not defined until the selection mode is exited by setting the rendering mode to VGL_RENDER using vgl_DrawFunRender.
DrawFun
NAME
vgl_DrawFunSelectRegion - specify selection regionC SPECIFICATION
void vgl_DrawFunSelectRegion (vgl_DrawFun *drawfun, Vint type, Vint iparam[])
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. type Type of selection region. =VGL_REGION_POLYGON Any vertex in a polygonal region =VGL_REGION_RECTANGLE Rectangular selection region =VGL_REGION_CLIPPLANE Any vertex inside clipping planes iparam Integer parameters used to define selection region.
OUTPUT ARGUMENTS
None
ERRORS
VGL_ERROR_ENUM is generated if an improper selection region type is specified.DESCRIPTION
Specify selection region. If the selection region is polygonal, then iparam[0] contains the number of points in the polygon followed by iparam[1] containing the x device coordinate of the first point in the polygon, iparam[2] contains the y device coordinate of the first point, iparam[3] contains the x device coordinate of the second point, etc. If VGL_REGION_POLYGON is specified, a hit is recorded for a given DataIndex if one or more vertices of any primitive corresponding to a given DataIndex lies within the polygonal region The polygonal region may be convex or concave and need not be a simple polygon, ie. the polygon borders may cross.If the selection region is a rectangle, then iparam[0] through iparam[3] contain the left, right, bottom and top device coordinates of the rectangle. A hit is recorded if one or more rasterized pixels of a primitive lie within the rectangular region.
If the selection region is VGL_REGION_CLIPPLANE, then iparam is ignored (it may be set to NULL), and the selection region is assumed to be the set of active clipping planes as defined by vgl_DrawFunClipPlane and vgl_DrawFunSetSwitch. Hits are determined by checking vertices against the clipping planes only. A hit is recorded for a given DataIndex if one or more vertices of any primitive corresponding to a given DataIndex lies within all active clipping planes.
Use vgl_DrawFunSelectQuery to return the number of hits. Use vgl_DrawFunSelectBuffer to specify the buffers used to record hit information.
DrawFun
NAME
vgl_DrawFunSetCursor - set the active cursor styleC SPECIFICATION
void vgl_DrawFunSetCursor (vgl_DrawFun *drawfun, Vint type)
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. type Cursor type =VGL_CURSOR_ARROW Left pointing arrow cursor =VGL_CURSOR_CROSSHAIR Crosshair cursor =VGL_CURSOR_DEFAULT Default cursor =VGL_CURSOR_DOLLY Dolly cursor =VGL_CURSOR_FAR Far plane cursor =VGL_CURSOR_HAND Hand cursor =VGL_CURSOR_LLANGLE Lower left angle cursor =VGL_CURSOR_LRANGLE Lower right angle cursor =VGL_CURSOR_MAGNIFYGLASS Magnifying glass cursor =VGL_CURSOR_NEAR Near plane cursor =VGL_CURSOR_PENCIL Pencil cursor =VGL_CURSOR_PIRATE Skull and cross bones cursor =VGL_CURSOR_QUESTION Question mark cursor =VGL_CURSOR_ROTATION Rotation cursor =VGL_CURSOR_TRANSLATION Translation cursor =VGL_CURSOR_ULANGLE Upper left angle cursor =VGL_CURSOR_URANGLE Upper right angle cursor =VGL_CURSOR_WATCH Watch cursor =VGL_CURSOR_ZOOM Zoom cursor
OUTPUT ARGUMENTS
None
DESCRIPTION
Set current cursor style.
DrawFun
NAME
vgl_DrawFunSetFactors - set factors to control shader modesC SPECIFICATION
void vgl_DrawFunSetFactors (vgl_DrawFun *drawfun, Vint type, Vfloat factors[])
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. type The type of factor to set. =VGL_ZBUFFEREDGESCALE Z Buffer edge lightness scaling =VGL_SSAODIST Occlusion region radius =VGL_WORLDSIZE World size of points.
OUTPUT ARGUMENTS
None
DESCRIPTION
Set various factors used as parameters to shader algorithms. These factors include scaling factors for z-buffer edge detection, etc.The VGL_ZBUFFEREDGESCALE factor is used to control the amount of lightness reduction as a function of z-buffer depth discontinuity. By default VGL_ZBUFFEREDGESCALE is set to 10000.
The VGL_SSAODIST factor is a physical distance used to limit the geometry considered for ambient light occlusion at a point. Any geometry more than this distance from a point is ignored as occluding geometry. A reasonable value for this parameter would be 5 percent of the model world coordinate extent. By default VGL_SSAODIST is set to 1.
The VGL_WORLDSIZE factor is a world coordinate size of world-sized points. For example the VGL_POINTSTYLE_SPHEREWORLD point style will draw spheres with a diameter of the current world size.
DrawFun
NAME
vgl_DrawFunSetMode - set current graphics modeC SPECIFICATION
void vgl_DrawFunSetMode (vgl_DrawFun *drawfun, Vint mode, Vint flag)
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. mode The graphics mode to turn on or off =VGL_BACKFACECULLMODE Back face culling =VGL_BLENDTRANSMODE Alpha blended transparency =VGL_CLIPMODE Clipping rectangle =VGL_COLORWRITEMODE Color buffer writing =VGL_DBUFFERMODE Double buffering =VGL_DATAINDEXMAXROWS Set maximum dataindex rows =VGL_DEPTHPEELMODE Depth peel number or mode =VGL_EVENTQUEUEMODE Button and key events =VGL_FRONTBUFFERDRAWMODE Front buffer drawing =VGL_FRONTBUFFERREADMODE Front buffer reading =VGL_FRONTFACECULLMODE Front face culling =VGL_FRONTFACEFLIPMODE Front face flip sense =VGL_LEFTBUFFERDRAWMODE Left buffer drawing =VGL_LIGHTMODE Lighting calculations =VGL_LINECULLMODE Line back pointing fulling =VGL_LINEDRAWMODE Line drawing =VGL_LINESTYLEFACTOR Line style integer scale factor =VGL_LINETRANSMODE Line, point, text transparency =VGL_LINEARRAYLIGHTMODE LineArray light mode =VGL_MULTISAMPLEMODE Multi-sampled mode =VGL_OITMODE Order independent transparency mode =VGL_OITAVERAGELAYERS Set average OIT layers per pixel =VGL_POINTARRAYLIGHTMODE PointArray light mode =VGL_POLYGONDEPTHMODE Polygon depth alteration =VGL_RASFONTDRAWMODE RasFont draw mode =VGL_SELECTSTACKMODE Select index stack mode =VGL_STENCILMODE Stencil buffering =VGL_STENCILWRITEMASK Stencil write mask =VGL_STENCILFUNCMODE Stencil function =VGL_STENCILFUNCREF Stencil function reference value =VGL_STENCILFUNCMASK Stencil function mask =VGL_STENCILOPFAIL Stencil operation fail =VGL_STENCILOPZFAIL Stencil operation z-buffer fail =VGL_STENCILOPZPASS Stencil operation z-buffer pass =VGL_TEXTANCHORMODE Text anchor mode =VGL_TEXTDIRECTIONMODE Text direction mode =VGL_TEXTPIXELSIZEMODE Text pixel size mode =VGL_TWOSIDELIGHTMODE Two sided lighting =VGL_UPDATEMODE Color and Z buffer initialization =VGL_XORMODE XOR drawing =VGL_ZBUFFERMODE Z-buffering =VGL_ZBUFFERFUNCMODE Z-buffer depth function =VGL_ZBUFFERWRITEMODE Z-buffer writing flag Mode flag =VGL_OFF Turn mode off =VGL_ON Turn mode on =VGL_RIGHT Text anchor right baseline. Text direction right =VGL_LEFT Text anchor left baseline. Text direction left =VGL_BOTTOM Text anchor bottom center. Text direction bottom =VGL_TOP Text anchor top center. Text direction top =VGL_CENTER Text anchor center baseline =VGL_BOTTOMRIGHT Text anchor bottom right =VGL_BOTTOMLEFT Text anchor bottom left =VGL_TOPRIGHT Text anchor top right =VGL_TOPLEFT Text anchor top left =VGL_LESS Function less than =VGL_LEQUAL Function less than or equal =VGL_GREATER Function greater than =VGL_GEQUAL Function greater than or equal =VGL_EQUAL Function equal =VGL_NOTEQUAL Function not equal =VGL_NEVER Function never =VGL_ALWAYS Function always =VGL_OP_ZERO Operation set to zero =VGL_OP_KEEP Operation keep =VGL_OP_REPLACE Operation replace =VGL_OP_INCR Operation increment =VGL_OP_DECR Operation decrement =VGL_OP_INVERT Operation invert =VGL_DEPTHPEELLAST Last depth peel.
OUTPUT ARGUMENTS
None
DESCRIPTION
Enable or disable a graphics mode or set a graphics mode value. Initially z-buffering is off, double buffering is on if supported by a device, front buffer drawing is off (drawing is to the back buffer), front buffer reading is on, XOR drawing is off, lighting calculations are off, clipping rectangle is off, button and key events are off, back face culling is off and polygon depth alteration is on. Text anchor mode is set to VGL_BOTTOMLEFT. Some graphics modes such as VGL_DBUFFERMODE may not be modifiable depending upon the underlying graphics hardware.The VGL_BLENDTRANSMODE is used to enable alpha blending for transparent polygon rendering. If disabled transparency is rendered using a "screen door" technique. By default VGL_BLENDTRANSMODE is set to off.
The VGL_DATAINDEXMAXROWS is used to enable data index rendering and set the maximum number of data indices to rendered at any pixel. A value of zero disables data index rendering. By default VGL_DATAINDEXMAXROWS is set to 0.
The VGL_DEPTHPEELMODE is used to enable depth peeling for accurate rendering of transparent objects. A scene is rendered repeatedly, once for each peel. The value of flag starts at one and increments for each peel. A final, specialized peel with a flag of VGL_DEPTHPEELLAST may be rendered if there is a residual of the scene yet to rendered in an approximate way. The user can query for the number of pixels touched by the previous peel using vgl_DrawFunGetInteger to determine if the peeling can be terminated. By default VGL_DEPTHPEELMODE is set to 0.
The VGL_COLORWRITEMODE is used to enable or disable writing to the color buffer. By default VGL_COLORWRITEMODE is set to on.
The VGL_FRONTFACEFLIPMODE is used to enable or disable the flipping of the definition of a front face. If disabled a front face has a right-hand sense, if enabled it has a left-hand sense. By default VGL_FRONTFACEFLIPMODE is set to off.
The VGL_TWOSIDELIGHTMODE is used to enable two sided lighting of polygons. In two sided lighting the normals of back-facing polygons have their normals reversed before lighting is evaluated. By default VGL_TWOSIDELIGHTMODE is set to off.
The VGL_LINECULLMODE is used to enable back "facing" line culling. This mode requires that shaders are enabled. and that normals are defined at the vertices of line primitives using either vgl_DrawFunPolyLineArray or vgl_DrawFunPolyLineBuffer. A line is assumed to be back facing if the first line endpoint normal is back pointing. By default VGL_LINECULLMODE is set to off.
The VGL_LINEDRAWMODE is used with software rendering modules such as RendBuf, DataBuf, GDIDev and X11Dev. If enabled, visible lines are drawn by a native line drawing function rather than being rasterized in software. By default VGL_LINEDRAWMODE is set to off.
The VGL_LINESTYLEFACTOR is used to set an integer line style factor. The current line style is expanded by this factor. By default VGL_LINESTYLEFACTOR is set to 1.
The VGL_LINETRANSMODE is used to apply transparency to line, point and text primitives. If disabled, transparency will effect only polygon primitives, if enabled transparency effects line, point and text primitives as well as polygon primitives. By default VGL_LINETRANSMODE is set to off.
The VGL_LINEARRAYLIGHTMODE is used to apply lighting to line primitives specified by the PolyLineArray drawing function. By default VGL_LINEARRAYLIGHTMODE is set to off.
The VGL_POINTARRAYLIGHTMODE is used to apply lighting to point primitives specified by the PolyPointArray drawing function. By default VGL_POINTARRAYLIGHTMODE is set to off.
The VGL_MULTISAMPLEMODE is used to toggle multi-sampling if a multi-sampled buffer has been selected. By default VGL_MULTISAMPLEMODE is set to on.
The VGL_OITMODE is used to enable a one-pass, order independent transparency feature. By default VGL_OITMODE is set to off.
Use VGL_OITAVERAGELAYERS to set the average number of layers per pixel to be used to allocate the internal OIT data structure. By default =VGL_OITAVERAGELAYERS is set to 16.
The VGL_RASFONTDRAWMODE is used to enable or disable drawing raster fonts explicitly by pixel. If enabled then raster fonts are drawn with pixel level graphics primitives rather than bitmaps. By default VGL_RASFONTDRAWMODE is set to off.
The VGL_SELECTSTACKMODE is used to enable or disable the method used to record hits during selection mode. If disabled then only the data index at the top of the index stack is placed in the hit buffer. If enabled the entire data index stack is placed in the hit buffer. By default VGL_SELECTSTACKMODE is set to off.
The VGL_STENCILMODE is used to enable or disable stencil buffer testing. By default VGL_STENCILMODE is set to off.
The VGL_STENCILWRITEMASK is used to control which planes of the stencil buffer are written to. By default VGL_STENCILWRITEMASK is set to all 1's.
The VGL_STENCILFUNCMODE specifies the stencil test function. Possible values are VGL_NEVER, VGL_ALWAYS, VGL_EQUAL, VGL_NOTEQUAL, VGL_LESS, VGL_LEQUAL, VGL_GREATER, VGL_GEQUAL, By default VGL_STENCILFUNCMODE is set to VGL_ALWAYS.
The VGL_STENCILFUNCREF specifies the stencil test function reference value. By default VGL_STENCILFUNCREF is set to zero.
The VGL_STENCILFUNCMASK specifies the stencil test function mask. By default VGL_STENCILFUNCMASK is set to all 1's.
The VGL_STENCILOPFAIL specifies the operation when the stencil test fails. Possible values are VGL_OP_KEEP, VGL_OP_ZERO, VGL_OP_REPLACE, VGL_OP_INCR, VGL_OP_DECR and VGL_OP_INVERT. By default VGL_STENCILOPFAIL is set to all VGL_KEEP.
The VGL_STENCILOPZFAIL specifies the operation when the stencil test passes and the z-buffer test fails.. Possible values and default are the same as VGL_STENCILOPFAIL.
The VGL_STENCILOPZPASS specifies the operation when the stencil test passes and the z-buffer test passes.. Possible values and default are the same as VGL_STENCILOPFAIL.
The VGL_TEXTANCHORMODE is used to set the anchor position of all drawn text. By default VGL_TEXTANCHORMODE is set to VGL_BOTTOMLEFT.
The VGL_TEXTDIRECTIONMODE is used to set the direction of text drawn with a non-orientable raster font. By default VGL_TEXTDIRECTIONMODE is set to VGL_RIGHT.
The VGL_TEXTPIXELSIZEMODE is used to enable or disable the text pixel size set by the drawing function vgl_DrawFunTextPixelSize. By default VGL_TEXTPIXELSIZE is set to VGL_OFF.
The VGL_ZBUFFERMODE is used to enable or disable z-buffer testing. By default VGL_ZBUFFERMODE is set to off.
The VGL_ZBUFFERWRITEMODE is used to enable or disable writing to the z buffer. By default VGL_ZBUFFERWRITEMODE is set to on.
The VGL_UPDATEMODE is used to enable or disable the frame buffer and zbuffer update feature. When enabled the current contents of the frame buffer the zbuffer are copied and stored by the device driver. Each time the Clear drawing function is called the saved contents are copied to the frame buffer and zbuffer. Any drawing which then occurs creates a scene which "updates" the scene contained in the saved buffers. The contents of the saved buffers are not changed only the contents of the current frame buffer and zbuffer. This allows for very quick updating of complex scenes. By default VGL_UPDATEMODE is set to off.
The VGL_ZBUFFERFUNCMODE is used to select the comparison function used to compare incoming z-buffer depth values to the current value stored in the z-buffer to test for visibility. Possible modes are VGL_LESS, VGL_LEQUAL, VGL_GREATER, VGL_GEQUAL, By default VGL_ZBUFFERFUNCMODE is set to VGL_LESS.
The VGL_LEFTBUFFERDRAWMODE is used to enable or disable writing to the left front or left back buffer when using a stereoscopic frame buffer. If VGL_LEFTBUFFERDRAWMODE is disabled then drawing will be directed to the right front or right back buffer. Control drawing to the front or back buffer using VGL_FRONTBUFFERDRAWMODE. By default VGL_LEFTBUFFERDRAWMODE is set to on.
Return modes as output arguments using
void vgl_DrawFunGetMode (vgl_DrawFun *drawfun, Vint mode, Vint *flag)
DrawFun
NAME
vgl_DrawFunSetSwitch - enable, disable lights, clipping planesC SPECIFICATION
void vgl_DrawFunSetSwitch (vgl_DrawFun *drawfun, Vint type, Vint index, Vint flag)
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. type Type of entity to enable/disable =VGL_LIGHT Light =VGL_CLIPPLANE Clipping plane index Index, 0 <= index flag Switch =VGL_OFF Turn off =VGL_ON Turn on
OUTPUT ARGUMENTS
None
DESCRIPTION
Enable or disable lights and clipping planes. For light entities, 0 <= index <= 7. Use vgl_DrawFunLight to define lights. For clipping plane entities, 0 <= index <= 5. Use vgl_DrawFunClipPlane to define clipping planes.
DrawFun
NAME
vgl_DrawFunSetWindow - set an object as current graphics window or fileC SPECIFICATION
void vgl_DrawFunSetWindow (vgl_DrawFun *drawfun)
INPUT ARGUMENTS
drawfun Pointer to DrawFun object.
OUTPUT ARGUMENTS
None
DESCRIPTION
If several graphics device interface objects are being used, only one at a time may be rendering to a graphics window on a single display. Therefore the user must specify the object which is the current renderer to the display.
DrawFun
NAME
vgl_DrawFunSpecularity - set current specularityC SPECIFICATION
void vgl_DrawFunSpecularity (vgl_DrawFun *drawfun, Vfloat intensity, Vfloat shininess)
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. intensity Current intensity of specular reflectance. shininess Current specular exponent
OUTPUT ARGUMENTS
None
DESCRIPTION
Set the current specular intensity and shininess. These properties affect the material properties of all subsequent polygon graphics primitives. The specular intensity ranges in the interval [0.,1.] the shininess must have values in the interval [0.,128.]. Initially the intensity is 0. and the shininess is 0.
DrawFun
NAME
vgl_DrawFunSwap - swap frame buffersC SPECIFICATION
void vgl_DrawFunSwap (vgl_DrawFun *drawfun)
INPUT ARGUMENTS
drawfun Pointer to DrawFun object.
OUTPUT ARGUMENTS
None
DESCRIPTION
Swap buffers in a double buffered graphics window or write a software frame buffer to a file for objects rendering to a host file. If a graphics window only supports a single buffered visual, then this function has no effect. All pending graphics functions are completed before the buffers are swapped.
DrawFun
NAME
vgl_DrawFunText - draw raster textC SPECIFICATION
void vgl_DrawFunText (vgl_DrawFun *drawfun, Vfloat x[3], Vtchar text[])
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. x World coordinate anchor point text Text string
OUTPUT ARGUMENTS
None
DESCRIPTION
Draw a text string anchored at world coordinate location x. The string is rendered using a raster font in the current color.
DrawFun
NAME
vgl_DrawFunTextDC - draw raster text with a device oriented offsetC SPECIFICATION
void vgl_DrawFunTextDC (vgl_DrawFun *drawfun, Vfloat x[3], Vint dc[3], Vtchar text[])
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. x World coordinate anchor point dc Device coordinate offset text Text string
OUTPUT ARGUMENTS
None
DESCRIPTION
Draw a text string with a device coordinate offset, dc anchored at world coordinate location x. The string is rendered using a raster font in the current color.
DrawFun
NAME
vgl_DrawFunTextPlane - specify raster font planeC SPECIFICATION
void vgl_DrawFunTextPlane (vgl_DrawFun *drawfun, Vfloat path[3], Vfloat plane[3])
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. path Raster font path plane Raster font plane
OUTPUT ARGUMENTS
None
DESCRIPTION
Specify raster font orientation. The path and plane vectors need not be normalized or orthogonal. The raster text string is drawn along the path vector in the plane defined by the path and plane vectors. The path and plane vectors can not be parallel. By default the path vector is (1.,0.,0.) and the plane vector is (0.,1.,0.)
DrawFun
NAME
vgl_DrawFunTextPixelSize - specify raster font pixel sizeC SPECIFICATION
void vgl_DrawFunTextPixelSize (vgl_DrawFun *drawfun, Vfloat pixelsize)
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. pixelsize World coordinate size of a pixel or raster in raster font
OUTPUT ARGUMENTS
None
DESCRIPTION
Specify raster font world coordinate pixel size. By default, the world coordinate pixel size of an orientable raster font is set using vgl_RasFontSetPixelSize. This pixel size may be overridden using vgl_DrawFunTextPixelSize by enabling the VGL_TEXTPIXELSIZEMODE mode using vgl_DrawFunSetMode By default the pixel size is 1.
DrawFun
NAME
vgl_DrawFunTextureDefine - define a texture map indexC SPECIFICATION
void vgl_DrawFunTextureDefine (vgl_DrawFun *drawfun, Vint index, vgl_Texture *texture)
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. index Texture map index, 1 <= index texture Pointer to Texture object.
OUTPUT ARGUMENTS
None
ERRORS
VGL_ERROR_VALUE is generated if an index out of range is specified. VGL_ERROR_NULLOBJECT is generated if a NULL texture object is input.DESCRIPTION
Define a texture map. Use the Texture module to build the texture map description. Texture objects are copied by the graphics interface object. If a texture map had been previously defined for an index, the current definition replaces it. Use vgl_DrawFunTextureSelect to select the current texture map index once it has been defined.
DrawFun
NAME
vgl_DrawFunTextureSelect - select current texture mapC SPECIFICATION
void vgl_DrawFunTextureSelect (vgl_DrawFun *drawfun, Vint index)
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. index Texture map index, 0 <= index
OUTPUT ARGUMENTS
None
ERRORS
VGL_ERROR_VALUE is generated if an index out of range is specified.DESCRIPTION
Select a current texture map index. This texture map remains in effect until vgl_DrawFunTextureSelect is called again. Use vgl_DrawFunTextureDefine to define a texture map index. A texture map index of 0 disables texture mapping. Texture map index 0 is the default.
DrawFun
NAME
vgl_DrawFunTrans,vgl_DrawFunTransIndex - set current transparencyC SPECIFICATION
void vgl_DrawFunTrans (vgl_DrawFun *drawfun, Vfloat t) void vgl_DrawFunTransIndex (vgl_DrawFun *drawfun, Vint index)
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. t Current transparency factor index Current transparency index
OUTPUT ARGUMENTS
None
DESCRIPTION
Set the current transparency. The transparency may be set by either specifying a transparency factor in the interval [0.,1.] or a transparency index in the interval [0,8]. A Transparency factor of 0. means perfectly opaque, a factor of 1. means perfectly transparent. Transparency indices are mapped linearly to the transparency factor interval of [0.,1.]. A transparency index of 0 is perfectly opaque, an index of 8 is perfectly transparent. Initially the transparency index is 0.
DrawFun
NAME
vgl_DrawFunViewport - define viewport transformationC SPECIFICATION
void vgl_DrawFunViewport (vgl_DrawFun *drawfun, Vint left, Vint right, Vint bottom, Vint top)
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. left Device coordinate of left plane of viewing volume right Device coordinate of right plane of viewing volume bottom Device coordinate of bottom plane of viewing volume top Device coordinate of top plane of viewing volume
OUTPUT ARGUMENTS
None
DESCRIPTION
Define a viewport transformation. The specified viewport transformation replaces the current viewport transformation. vgl_DrawFunViewport specifies the mapping of the horizontal and vertical bounds of the viewing volume to a rectangular area in device coordinates. Note that the window device coordinate limits are [0,width-1] and [0,height-1] where width and height are the dimensions of the window in pixels. The left, right, bottom and top values are clamped to window device limits before they are accepted. Initially the viewport is set to the device limits of the window.
DrawFun
NAME
vgl_DrawFunVisualWindow - specify a frame buffer visualC SPECIFICATION
void vgl_DrawFunVisualWindow (vgl_DrawFun *drawfun, Vint visflag)
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. visflag ORed flags indicating frame buffer configuration =VGL_VISUAL_DEFAULT Set default visual =VGL_VISUAL_MULTISAMPLE Set multi-sampled buffer =VGL_VISUAL_SINGLEBUFFER Set single buffer =VGL_VISUAL_USECONTEXT Use current grapbics context =VGL_VISUAL_STEREO Set stereo pixel format
OUTPUT ARGUMENTS
None
DESCRIPTION
Specify the configuration of the color frame buffer. By default visflag is zero, the frame buffer will be double buffered, truecolor and monoscopic if possible.The VGL_VISUAL_DEFAULT option will select the default visual under X windows.
The VGL_VISUAL_MULTISAMPLE option will select a multi-sampled buffer for anti-aliasing effects.
The VGL_VISUAL_USECONTEXT option is used when the application is designed to create the graphics window and graphics context. In this case the "window" argument in the call to vgl_DrawFunConnectWindow is ignored and the current graphics context is queried. In Microsoft Windows this is a HGLRC. In X Windows this is a GLXContext.
Use VGL_VISUAL_STEREO to select a stereoscopic visual.
This function should be called before vgl_DrawFunOpenWindow or vgl_DrawFunConnectWindow.
DrawFun
NAME
vgl_DrawFunWarpMouse - move mouse to specified locationC SPECIFICATION
void vgl_DrawFunWarpMouse (vgl_DrawFun *drawfun, Vint px, Vint py)
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. px,py Pixel coordinates to move mouse
OUTPUT ARGUMENTS
None
DESCRIPTION
Move mouse to specified pixel coordinates in graphics window.
DrawFun
NAME
vgl_DrawFunXfmLoad,vgl_DrawFunXfmMult - load and multiply the current matrixC SPECIFICATION
void vgl_DrawFunXfmLoad (vgl_DrawFun *drawfun, Vfloat m4x4[4][4]) void vgl_DrawFunXfmMult (vgl_DrawFun *drawfun, Vfloat m4x4[4][4])
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. m4x4 A 4 by 4 matrix stored in column major order
OUTPUT ARGUMENTS
None
DESCRIPTION
vgl_DrawFunXfmLoad loads m4x4 onto the top of the stack replacing the current matrix. vgl_DrawFunXfmMult pre multiplies m4x4 by the current matrix. The result replaces the current matrix.
DrawFun
NAME
vgl_DrawFunXfmPop,vgl_DrawFunXfmPush - pop and push transformation stackC SPECIFICATION
void vgl_DrawFunXfmPop (vgl_DrawFun *drawfun) void vgl_DrawFunXfmPush (vgl_DrawFun *drawfun)
INPUT ARGUMENTS
drawfun Pointer to DrawFun object.
OUTPUT ARGUMENTS
None
DESCRIPTION
Push and pop the modelview transformation stack. vgl_DrawFunXfmPush pushes the matrix stack, duplicating the top matrix. vgl_DrawFunXfmPop pops the matrix stack, replacing the top matrix with the one below it. The depth of the modelview matrix stack is at least 32. The modelview transformation stack initially contains the identity matrix.
DrawFun
NAME
vgl_DrawFunZBufferRead,vgl_DrawFunZBufferWrite - read and write z bufferC SPECIFICATION
void vgl_DrawFunZBufferRead(vgl_DrawFun *drawfun, Vint left, Vint right, Vint bottom, Vint top, vgl_ZBuffer *zbuffer) void vgl_DrawFunZBufferWrite(vgl_DrawFun *drawfun, Vint left, Vint right, Vint bottom, Vint top, vgl_ZBuffer *zbuffer)
INPUT ARGUMENTS
drawfun Pointer to DrawFun object. left,right Pixel coordinates of left and right limits of rectangle bottom,top Pixel coordinates of bottom and top limits of rectangle zbuffer Pointer to ZBuffer object to read into or write from.
OUTPUT ARGUMENTS
None
DESCRIPTION
Read z buffer contents into a ZBuffer object or write contents of a ZBuffer object to a z buffer. The user may specify a subset of the z buffer to be read from by specifying the limits of a rectangle of the z buffer in pixels with left, right, bottom and top. If these arguments are set to zero, then the entire z buffer is assumed. When reading into a ZBuffer object, any previous contents are freed and the ZBuffer object is configured to contain the rectangle of z buffer data specified. When writing from a ZBuffer object to the z buffer, the rectangle limits must match the dimensions of the data held in the ZBuffer object or the results are undefined. ZBuffer objects hold z buffer information in a device independent format, they may be read and written to any z buffer.The current z buffers for reading and writing (drawing) are determined by the VGL_FRONTBUFFERREADMODE and VGL_FRONTBUFFERDRAWMODE modes specified with the vgl_DrawFunSetMode function.