4. 3D Curve and Surface Mesh Generation - CurvMesh, SurfMesh
CEETRON Mesh supports automatic 3D curve and surface mesh generation using the CurvMesh and SurfMesh modules. The modules are designed to generate linear, parabolic or cubic elements from a discrete input representation of the underlying geometry. This means that input curves are specified as a series of line segments and input surfaces are specified as a set of triangles. This type of geometry representation is often referred to as “facetted” geometry. Options exist to allow the input geometry to be augmented by specification of normal vector information at line or triangle vertices. An important addition to the facetted geometry is the specification of certain topological constraints such as geometry points and edges in a surface triangulation. These points and edges are termed “preserved” points and edges in the input to CurvMesh and SurfMesh.
The input geometry is often the result of a tessellation produced from an underlying geometry engine such as Parasolid or ACIS. The refinement of the tessellation may generally be controlled by a number of parameters. The parameters fall into two general categories; 1) angular tolerances and 2) size tolerances. It is advised that curve and surface angular tolerances be set to 2*PI/8 radians (45 degrees). This is the maximum angle spanned by a curve chord or surface plane in the tessellation. The size tolerances should be relative to the length of the diagonal of the bounding box of the geometry model. The maximum facet width should be between .25 and .5 (.4 recommended) of the diagonal length. The curve chord height and surface plane height tolerance should be between .0001 and .001 (.0005 recommended) of the diagonal length.
4.1. Curve Mesh Generation - CurvMesh
The CurvMesh module provides for the automatic generation of linear, parabolic or cubic line elements given an input curve geometry as a set of line segments. The input lines consist of a segmentation of the curve geometry into line segments with optional line end point tangent vectors. There are no limits placed on the length of the input lines, they only need to represent the curve geometry to the desired degree of precision. Any point of the input curve may be preserved in the final mesh. The input segmentation must be conformal but not necessarily closed or manifold. This implies that CurvMesh may be used to generate line meshes on tessellations produced by CAD systems, existing finite element meshes, etc. The functions associated with a CurvMesh object are the following.
Begin and end an instance of an object, return object error flag
vis_CurvMeshBegin()
- create an instance of a CurvMesh objectvis_CurvMeshEnd()
- destroy an instance of a CurvMesh objectvis_CurvMeshError()
- return CurvMesh object error flag
Define curve mesh, mesh parameters and generate mesh
vis_CurvMeshDef()
- define number of curve points, lines.vis_CurvMeshInq()
- inquire number of curve points, linesvis_CurvMeshGenerate()
- generate finite element meshvis_CurvMeshGetConnect()
- get internal Connect objectvis_CurvMeshGetInteger()
- get integer mesh generation informationvis_CurvMeshSetParami()
- set mesh generation parametersvis_CurvMeshGetParami()
- get mesh generation parametersvis_CurvMeshSetParamd()
- set mesh generation parametersvis_CurvMeshGetParamd()
- get mesh generation parametersvis_CurvMeshSetPoint()
- define a curve pointvis_CurvMeshSetPointAssoc()
- define a point associationvis_CurvMeshSetPointSizing()
- define a point sizingvis_CurvMeshSetLine()
- define a curve line segmentvis_CurvMeshSetLineAssoc()
- define a line associationvis_CurvMeshSetLineTang()
- define line segment tangentsvis_CurvMeshWrite()
- write curve description to filevis_CurvMeshRead()
- read curve description from file
Instance a CurvMesh object using vis_CurvMeshBegin()
.
Once a CurvMesh object is instanced,
define the number of points and lines which define the curve mesh
using vis_CurvMeshDef()
.
The curve geometry is then input as
a set of points in 3D space and a set of line connectivities.
The points are defined using vis_CurvMeshSetPoint()
.
The line connectivities are defined using vis_CurvMeshSetLine()
.
The function vis_CurvMeshSetParami()
is used to set integer parameters which
affect the mesh generation process.
The function vis_CurvMeshSetParamd()
is used to set real double precision
parameters which control the quality and size of the generated elements.
A global target edge length for elements may be prescribed.
Element size in areas of high curvature may be controlled by a user specified
maximum spanning angle. The overall bound on the minimum edge length may also
be input. Edge lengths may be specified at each input point using the
function vis_CurvMeshSetPointSizing()
. This function is used to input
sizing information for a mesh adaptation or regridding. If the input
curves represent the boundary of a surface mesh, additional sizing information
from the surface mesh due to proximity or curvature may be input in this way.
The user is able to define integer associations at points and lines
which will be assigned to the node or nodes generated on the
respective entity.
These associations are useful for identifying nodes and elements
in the output mesh for the application of boundary conditions.
The function vis_CurvMeshSetPointAssoc()
is used to set associations at
nodes generated at defined points,
while the function vis_CurvMeshSetLineAssoc()
is used to
set associations for nodes generated along the input lines.
if a node is assigned associations from both
point and line associations specified by the user, then point associations
take precedence over line associations.
Finally the function vis_CurvMeshGenerate()
generates the
nodes and finite elements and enters them into a Connect object.
It is strongly suggested that the Connect object be set to store
node coordinates in double precision using vis_ConnectPre()
.
Use vis_CurvMeshGetInteger()
to query for detailed information concerning errors in the input lines,
etc.
The function vis_CurvMeshWrite()
is provided to write a complete description
of the defined input geometry and meshing parameters to a file. The primary
use of this file is to encapsulate cases
in which the CurvMesh module fails
in some respect. This output file can then be
made available to Tech Soft 3D for failure diagnosis of CurvMesh meshing
algorithms.
The following code fragment illustrates the basic framework of using the CurvMesh module to generate parabolic line elements on a curve defined by a set of line segments.
/* declare objects */
vis_CurvMesh *curvmesh;
vis_Connect *connect;
/* define curve data */
Vint numpnts, numlins;
Vdouble xpnt[][3] = { ... };
Vint pres[] = { ... };
Vint ixlin[][3] = { ... };
/* create curvmesh object */
curvmesh = vis_CurvMeshBegin ();
vis_CurvMeshDef (curvmesh,numpnts,numlins);
/* input points and lines */
for (i = 0; i < numpnts; i++) {
vis_CurvMeshSetPoint (curvmesh,i+1,xpnt[i],pres[i]);
}
for (i = 0; i < numlins; i++) {
vis_CurvMeshSetLine (curvmesh,i+1,ixlin[i]);
}
/* create empty connect object to hold generated mesh */
connect = vis_ConnectBegin ();
vis_ConnectPre (connect,SYS_DOUBLE);
vis_ConnectDef (connect,0,0);
/* ask for about 200 generated parabolic lines */
vis_CurvMeshSetParami (curvmesh,VIS_MESH_NUMELEMENTS,200);
vis_CurvMeshSetParami (curvmesh,VIS_MESH_MAXI,3);
/* generate mesh */
vis_CurvMeshGenerate (curvmesh,connect);
/* access generated nodes and elements from Connect */
...
/* delete objects */
vis_ConnectEnd (connect);
vis_CurvMeshEnd (curvmesh);
4.2. Function Descriptions
The currently available CurvMesh functions are described in detail in this section.
-
void vis_CurvMeshEnd(vis_CurvMesh *p)
destroy an instance of a CurvMesh object
See
vis_CurvMeshBegin()
-
Vint vis_CurvMeshError(vis_CurvMesh *p)
return the current value of a CurvMesh object error flag
See
vis_CurvMeshBegin()
-
void vis_CurvMeshGetParami(vis_CurvMesh *p, Vint type, Vint *iparam)
get mesh generation parameters
See
vis_CurvMeshSetParami()
4.3. Surface Mesh Generation - SurfMesh
The SurfMesh module provides for the automatic generation of linear, parabolic or cubic triangular or quadrilateral elements given an input surface geometry. The input surface geometry consists of a tessellation of the surface geometry into triangles. There are no limits placed on the size and shape of the input triangles, they only need to represent the surface geometry to the desired degree of precision. Any edge of any input triangulation may be flagged to be preserved in the final mesh. The input triangulation must be conformal but not necessarily closed. This implies that SurfMesh may be used to generate meshes on tessellations produced by CAD systems, existing finite element meshes, the triangulations produced by isosurfacing algorithms, etc. The functions associated with a SurfMesh object are the following.
Begin and end an instance of an object, return object error flag
vis_SurfMeshBegin()
- create an instance of a SurfMesh objectvis_SurfMeshEnd()
- destroy an instance of a SurfMesh objectvis_SurfMeshError()
- return SurfMesh object error flag
Define surface mesh, mesh parameters and generate mesh
vis_SurfMeshAbort()
- set abort flagvis_SurfMeshDef()
- define number of surface points, tris.vis_SurfMeshInq()
- inquire number of surface points, tris.vis_SurfMeshComputeArea()
- compute areavis_SurfMeshGenerate()
- generate finite element meshvis_SurfMeshGetConnect()
- get internal Connect objectvis_SurfMeshGetInteger()
- get integer mesh generation informationvis_SurfMeshRefine()
- refine a existing finite element meshvis_SurfMeshSetConic()
- set conic sectionsvis_SurfMeshSetEdge()
- set edges to be recoveredvis_SurfMeshSetFunction()
- set a call back functionvis_SurfMeshSetGeomSizing()
- specify sizing within geometric shapevis_SurfMeshSetParami()
- set mesh generation parametersvis_SurfMeshGetParami()
- get mesh generation parametersvis_SurfMeshSetParamd()
- set mesh generation parametersvis_SurfMeshGetParamd()
- get mesh generation parametersvis_SurfMeshSetPoint()
- define a surface pointvis_SurfMeshGetPoint()
- get a surface pointvis_SurfMeshSetPointAssoc()
- define a point associationvis_SurfMeshGetPointAssoc()
- query a point associationvis_SurfMeshSetPointSizing()
- define a point sizingvis_SurfMeshSetTri()
- define a surface trianglevis_SurfMeshGetTri()
- query a surface trianglevis_SurfMeshSetTriAssoc()
- define a triangle associationvis_SurfMeshGetTriAssoc()
- query a triangle associationvis_SurfMeshSetTriBack()
- specify material boundednessvis_SurfMeshSetTriConic()
- specify conic associated with a trianglevis_SurfMeshSetTriFeat()
- define triangle edge featurevis_SurfMeshSetTriHint()
- specify topological location of pointvis_SurfMeshSetTriPair()
- specify paired triangles on periodic facesvis_SurfMeshSetTriTang()
- define triangle edge vertex tangentsvis_SurfMeshGetTriTang()
- query triangle edge vertex tangentsvis_SurfMeshSetTriSizing()
- define triangle face and edge sizingvis_SurfMeshSetTriDepthSizing()
- set sizing within a depth at a trianglevis_SurfMeshSetTriNorm()
- define triangle vertex normalsvis_SurfMeshGetTriNorm()
- query triangle vertex normalsvis_SurfMeshWrite()
- write surface description to filevis_SurfMeshRead()
- read surface description from file
Instance a SurfMesh object using vis_SurfMeshBegin()
.
Once a SurfMesh object is instanced,
define the number of points and triangles which define the surface geometry
using vis_SurfMeshDef()
.
The surface geometry is then input as
a set of points in 3D space and a set of triangle
connectivities and edge flags.
The points are defined using vis_SurfMeshSetPoint()
.
The triangle connectivities and preserved edge flags
are defined using vis_SurfMeshSetTri()
.
Optional triangle vertex normals may be specified using
vis_SurfMeshSetTriNorm()
.
Optional triangle edge vertex tangents may be specified using
vis_SurfMeshSetTriTang()
.
A user defined callback function may be optionally defined to project
generated points exactly to the underlying geometry
using vis_SurfMeshSetFunction()
.
The node connectivity and edge numbering
convention for input surface triangles is
illustrated in Figure 27-1.
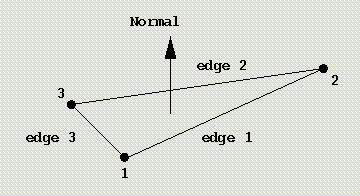
Figure 27-1, Input Surface Triangle Connectivity and Edge Convention
The geometry of the input triangulation is assumed to have the following properties.
The input points lie on the surface geometry.
The surface geometry as represented by the planar triangular facets is non-intersecting.
The input triangulation should represent the underlying geometry to the desired degree of precision. It is highly recommended in this regard that the basic point location and triangle connectivity be augmented with triangle vertex normals and triangle preserved edge tangents. If triangle normals or tangents are not supplied they are approximated from the input tesselation. Everywhere in which surface curvature is present the input linear triangles are converted to quadratic triangles using the normal information which has been supplied. This allows for a more accurate surface approximation. The quadratic triangles are represented by calculating 3 additional points at the midside of each triangle edge. The calculation of a midside point on a triangle edge begins by estimating a midside location from a Hermitian polynomial which constructs a cubic from the edge endpoint locations and normals. Since the computed midside point must be shared by an adjacent triangle, the initial midside point location is iteratively modified to lie on the edge intersection between the surfaces described by adjacent parabolic triangles.
During the quadratic reconstruction of each triangle a continuous check is made for the maintenance of accurate normals by the triangle. It is possible that a quadratic triangle may distort sufficiently that its computed normals become inaccurate or even suffer a reversal of sense. In this case the midside point locations are successively relaxed to the original facetted geometry until the computed normals are accurate.
Any point which is defined using vis_SurfMeshSetPoint()
and is not referenced
by a triangle connectivity is termed an “unconnected” point. Any unconnected
point is entered into the geometry triangulation and is automatically preserved.
The function vis_SurfMeshSetTriHint()
is used to guide the placement
of unconnected points in the triangulation.
If an unconnected point is inserted very close to a preserved edge, it
is important to set the appropriate triangle edge hint for such a point,
otherwise it will project to the triangle very near the
edge. Since all user defined nodes are preserved this means that a very
small triangle must be generated to connect the node somehow to the
edge which is very close to it.
The function vis_SurfMeshSetParami()
is used to set integer parameters which
affect the mesh generation process. Use this function to indicate such
options as whether
triangles or quadrilaterals are to be generated, the order of the generated
elements (linear, parabolic or cubic), etc.
The function vis_SurfMeshSetParamd()
is used to set real double precision
parameters which control the quality and size of the generated elements.
A global target edge length for elements may be prescribed.
Element size in areas of high curvature may be controlled by a user specified
maximum spanning angle. The overall bound on the minimum edge length may also
be input. The minimum small feature length and angle are specified
using vis_SurfMeshSetParamd()
. These parameters are used for the
deletion of small features from the input geometry.
In addition to the global sizing parameters, specific size controls
may be set on specific points using vis_SurfMeshSetPointSizing()
and triangle faces and edges using vis_SurfMeshSetTriSizing()
.
Note that the size assigned to any location on the input geometry
is subject to the minimum of all input sizes.
The user is able to define integer associations at points and triangles
which will be assigned to the node or nodes and elements generated on the
respective entity.
These associations are useful for identifying nodes and elements
in the output mesh for the application of loads, boundary conditions, material
properties, etc.
The function vis_SurfMeshSetPointAssoc()
is used to set associations at
nodes generated at defined points.
The function vis_SurfMeshSetTriAssoc()
is used to
set associations which will be attached to nodes generated along
triangle edges or on triangle faces. These associations will also
be generated as element entity associations along the respective
triangle edges and faces.
It is also used to set element associations on elements generated on a triangle.
In order to be used effectively, identical edge associations should be placed
on every triangle edge bound by preserved input nodes. Similarly,
identical face and element associations should be placed on every
triangle bound by preserved input edges.
Finally the function vis_SurfMeshGenerate()
generates the
nodes and elements and enters them into a Connect object.
It is strongly suggested that the Connect object be set to store
node coordinates in double precision using vis_ConnectPre()
.
The user may query for non-manifold and inconsistent triangles detected in the
input by calling vis_SurfMeshGetInteger()
after vis_SurfMeshGenerate()
is
called.
The function vis_SurfMeshWrite()
is provided to write a complete description
of the defined input surface and meshing parameters to a file. The primary
use of this file is to encapsulate cases
in which the SurfMesh module fails
in some respect. This output file can then be
made available to Tech Soft 3D for failure diagnosis of SurfMesh meshing
algorithms.
An option is provided to compute normals at the generated element nodes.
Enable this option using vis_SurfMeshSetParami()
with
parameter VIS_MESH_COMPUTENORMAL. The computed normals are
entered into an ElemDat object which must be registered with
the Connect object used to receive the output mesh.
Note that since this ElemDat object will manage element node data,
a GridFun object must be registered with it which has been
initialized with vis_ConnectGridFun()
on the output Connect object.
An existing element mesh may be refined using vis_SurfMeshRefine()
.
Mesh refinement is performed on the mesh resulting from the
call to vis_SurfMeshGenerate()
or the last previous call to
vis_SurfMeshRefine()
.
A State object of element edge length
is input and the mesh is refined to satisfy the new element edge lengths.
Only mesh refinement is performed, all previously existing nodes are
retained in the refined mesh.
Any element with a size of zero in the state object will not be refined.
The SurfMesh module provides for a monitor
callback function to be specified which
is called intermittently during the mesh generation process in
vis_SurfMeshGenerate()
. The primary purpose of this function is to
allow the user to interrupt or interrogate
the on-going mesh generation process for any reason. If mesh generation is to
be terminated, call vis_SurfMeshAbort()
. This call will set an internal
flag which will cause vis_SurfMeshGenerate()
or vis_SurfMeshRefine()
to terminate the mesh generation
process and return.
In addition to a monitor function, user defined isotropic and anisotropic
sizing callback functions and geometry projection callback functions may be set.
Set callback functions using vis_SurfMeshSetFunction()
.
The following code fragment illustrates the basic framework of using the SurfMesh module to generate parabolic triangular finite elements on a surface defined by a set of linear triangular facets.
/* declare objects */
vis_SurfMesh *surfmesh;
vis_Connect *connect;
/* define surface triangle data */
Vint numpnts, numtris;
Vdouble xpnt[][3] = { ... };
Vint pres[] = { ... };
Vint ixtri[][3] = { ... };
Vint eftri[][3] = { ... };
Vdouble vtri[][3] = { ... };
/* create surfmesh object */
surfmesh = vis_SurfMeshBegin ();
vis_SurfMeshDef (surfmesh,numpnts,numtris);
/* input points */
for (i = 0; i < numpnts; i++) {
vis_SurfMeshSetPoint (surfmesh,i+1,xpnt[i],pres[i]);
}
/* input triangles and triangle normals */
for (i = 0; i < numtris; i++) {
vis_SurfMeshSetTri (surfmesh,i+1,ixtri[i],eftri[i]);
vis_SurfMeshSetTriNorm (surfmesh,i+1,vtri[i]);
}
/* create empty connect object to hold generated mesh */
connect = vis_ConnectBegin ();
vis_ConnectPre (connect,SYS_DOUBLE);
vis_ConnectDef (connect,0,0);
/* set target edge length to .1 */
vis_SurfMeshSetParamd (surfmesh,VIS_MESH_EDGELENGTH,.1);
/* generate parabolic elements */
vis_SurfMeshSetParami (surfmesh,VIS_MESH_MAXI,3);
/* generate mesh */
vis_SurfMeshGenerate (surfmesh,connect);
/* access generated nodes and elements from Connect */
...
/* delete objects */
vis_ConnectEnd (connect);
vis_SurfMeshEnd (surfmesh);
An example of an input surface triangulation and the output generated triangle mesh is shown in Figures 27-2a and 27-2b. In this case the input surface is a closed surface enclosing a solid body. The output triangle mesh is suitable as an input surface triangulation for a subsequent volume tetrahedralization mesh generation process as provided by a CEETRON Mesh module such as TetMesh.
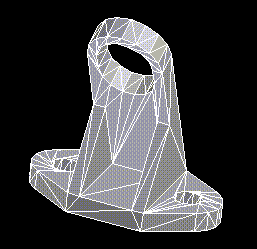
Figure 27-2a, Input Surface Triangles
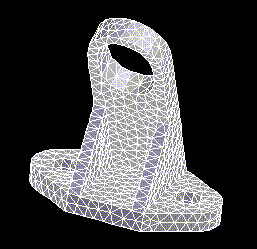
Figure 27-2b, Output Generated Triangles
4.4. Conic Section Geometry
The SurfMesh module supports explicit specification of geometry
which can be represented by conic sections. These sections
include plane, cone, cylinder, torus and sphere.
Each conic section is input using vis_SurfMeshSetConic()
and is assigned
an arbitrary positive integer identifier. Each input triangle which
lies on a particular conic section must be identified as such by using
vis_SurfMeshSetTriConic()
.
All conic sections are presented by a type and sense, an origin, an axis unit vector, a reference unit vector as well as a scalar constant representing a distance, radius or angle (in degrees) and a scalar parameter representing a major radius. The axis represents the local z axis of the coordinte system, and the reference direction represents the local x axis. The sense indicates the direction of the normals to the surface represented by the conic section.
Plane, type SURFMESH_CONIC_PLANE, is positioned normal to the axis a scalar constant distance along the specified axis from the origin. Positive sense normals are in the direction of the axis.
Cone, type SURFMESH_CONIC_CONE, is positioned with its apex at the origin and aligned to the axis. The scalar constant half angle of the cone is measured from the positive direction of the axis. The half angle is greater than zero and less than 90 degrees. Positive sense normals point away from the axis.
Cylinder, type SURFMESH_CONIC_CYLINDER, is aligned to the axis which passes through the origin. The cylinder has a specified scalar constant radius. Positive sense normals point away from the axis.
Torus, type SURFMESH_CONIC_TORUS, is aligned to the axis which passes through the origin. The torus has specified scalar parameter major radius and scalar constant minor radius. The major radius is measured from the origin perpendicular to the axis. The minor radius is measured from the center line defined by the major radius. Positive sense normals point away from the center line of the minor radius.
Sphere, type SURFMESH_CONIC_SPHERE, is aligned to the axis with its center at the the origin. The sphere has a specified scalar constant radius. Positive sense normals point away from the center.
4.5. Mapped Mesh Regions
The SurfMesh module can detect faces bound by preserved edges
which form rectangular regions which are suitable for
mapped meshes to be generated. Mapped meshing of such regions
provides for the generation of highly regular, rectilinear meshes.
The detection of map meshable regions is enabled with
the VIS_MESH_MAPDETECT parameter using the function vis_SurfMeshSetParami()
.
By default mapped mesh region detection is disabled.
A single angle parameter, VIS_MESH_MAPANGLE, can be set using
vis_SurfMeshSetParamd to control orthogonality of the map meshable regions.
The angle parameter is a deviation from 90 degrees for corner edges and a
deviation from 180 degrees for side edges.
If triangular elements are generated in map meshable regions, the elements
will contain a nearly right angle. This is in contrast to the equilateral
triangles which are generated in free meshed regions. If quadrilateral
elements are to be generated, an option exists to quadrilateralize
only map meshable regions and triangulate all other regions.
Set this option with the parameter VIS_MESH_MAPRECTONLY using the function
vis_SurfMeshSetParami()
.
If quadrilateral elements are to be generated, by default
a quad “dominant” mesh is generated in which
triangle elements are used in
transitions, etc. where a quadrilateral is not strictly required due
to topological or severe geometrical constraints.
If an all quadrilateral mesh is meant to be generated then
the quad dominant option must be disabled
using the parameter SURFMESH_QUADDOM in the function
vis_SurfMeshSetParami()
.
4.6. Non-manifold Geometry, Voids and Inclusions
The SurfMesh module supports the surface meshing of non-manifold geometries,
in which more than two input triangles share a common edge.
Such geometries are common when modelling branched shell structures,
assemblies of solids, multiple material solids, regions with baffles, etc.
By default however for historical reasons, the SurfMesh module
assumes that non-manifold
geometry is a modelling error and attempts to remove non-manifold
input triangles from the geometry. Use the SURFMESH_NONMANIFOLD parameter
with the function vis_SurfMeshSetParami()
to enable support for
non-manifold geometries.
The SurfMesh module is often used to triangulate the boundaries
of a solid region for subsequent tetrahedral mesh generation.
In this case it is necessary to be able to distinguish whether a particular
generated triangle is on an external boundary (including the boundary
of a void) of the solid or an internal surface of the solid. Such
internal surfaces would include non-manifold surfaces not on an
external boundary and any surface of an inclusion.
All generated triangles on internal surfaces may be flagged with an element
association. Use the parameter SURFMESH_INTSURFFLAG in function
vis_SurfMeshSetParami()
to specify the element association to be
flagged.
Two procedures exist to facilitate generating triangulations
on the boundaries of solids which are to be subsequently tetrahedralized by
the TetMesh module.
The TetMesh module requires the right-hand rule
connectivity sense of all input triangles to point out of a material region.
This means that all triangles on internal surfaces which bound two material
regions must be “double backed”, that is, there are two coincident triangles
sharing nodes with opposite sense.
The first procedure is an option to automatically detect all internal
triangles and generate double-backed triangles on these internal surfaces.
This option is enabled
using the SURFMESH_INTSURFBACK parameter in function vis_SurfMeshSetParami()
.
There are cases when this automatic detection will fail. For example if
an inclusion is bound by input triangles which all point into the inclusion.
The second procedure requires the user to explicitly flag each input triangle
with its material boundedness with respect to its connectivity sense.
The material boundedness is specified using vis_SurfMeshSetTriBack()
.
This option is normally used when the SurfMesh input is being
driven by a commercial geometry engine.
The function vis_SurfMeshSetTriAssoc()
has options to set an element
association for both the right and left sides of an input triangle.
These element associations are ultimately propagated to TetMesh
to specify the element associations to identify material regions
of the generated tetrahedra.
4.7. Periodic Meshes
The SurfMesh module supports generating periodic meshes
on specified periodic geometry faces. The function vis_SurfMeshSetTriPair()
is used to specify matching pairs of input triangles which mark
a master face and the paired slave face. Each triangle must lie
on a preserved edge attached to a preserved node.
The preserved node on the master triangle is meant to match
the preserved node on the slave triangle.
This implies that each face must contain at least one preserved
node which matches a preserved node on the other face.
A face is a set of triangles bound
by preserved edges. The topology of the master and slave faces
must match exactly and when geometrically oriented must be geometrically
identical to within a small tolerance.
If the periodic faces are planar and rotationally periodic,
the function vis_SurfMeshComputeTriPair()
may be used to
compute the triangle pairs for all geometry faces on the geometrically
specified master and slave planes.
4.8. Function Descriptions
The currently available SurfMesh functions are described in detail in this section.
-
vis_SurfMesh *vis_SurfMeshBegin(void)
create an instance of a SurfMesh object
Create an instance of a SurfMesh object. Memory is allocated for the object private data and the pointer to the data is returned.
Destroy an instance of a SurfMesh object using
void vis_SurfMeshEnd (vis_SurfMesh *surfmesh)
Return the current value of a SurfMesh object error flag using
Vint vis_SurfMeshError (vis_SurfMesh *surfmesh)
- Returns
The function returns a pointer to the newly created SurfMesh object. If the object creation fails, NULL is returned.
-
void vis_SurfMeshEnd(vis_SurfMesh *p)
destroy an instance of a SurfMesh object
-
Vint vis_SurfMeshError(vis_SurfMesh *p)
return the current value of a SurfMesh object error flag
-
void vis_SurfMeshDef(vis_SurfMesh *p, Vint numpnts, Vint numtris)
define number of surface points, tris.
Define the number of points and triangles which define the input surface mesh. Define point coordinates using
vis_SurfMeshSetPoint()
and define triangle connectivities which reference the input points usingvis_SurfMeshSetTri()
.Inquire of defined numpnts and numtris as output arguments using
void vis_SurfMeshInq (vis_SurfMesh *surfmesh, Vint *numpnts, Vint *numtris)
- Errors
VIS_ERROR_VALUE
is generated if numpnts or numtris is less than or equal to zero.
- Parameters
p – Pointer to SurfMesh object.
numpnts – Number of points on input surface mesh
numtris – Number of triangles on input surface mesh
-
void vis_SurfMeshInq(vis_SurfMesh *p, Vint *numpnts, Vint *numtris)
inquire of defined numpnts and numtris as output arguments
-
void vis_SurfMeshSetFunction(vis_SurfMesh *p, Vint funtype, Vfunc *function, Vobject *object)
set a call back function
Set callback functions. By default the callback functions are NULL. A callback is not invoked if it is NULL. If an anisotropic sizing function is set, it takes precedence over any isotropic sizing function which has been set.
The monitor callback function prototype is
The first argument is the SurfMesh object, surfmesh, and the second is a user defined object, object.void function (vis_SurfMesh *surfmesh, Vobject *object)
The sizing callback function prototype is
The first argument is the SurfMesh object, surfmesh, the second is a user defined object, object, the third is the coordinate location x and the fourth is the returned size s.void function (vis_SurfMesh *surfmesh, Vobject *object, Vdouble x[3], Vdouble *s)
The anisotropic sizing callback function prototype is
The first argument is the SurfMesh object, surfmesh, the second is a user defined object, object, the third is the coordinate location x and the fourth is the returned anisotropic scaled orthogonal direction vectors. The first 3 components are the first size scaled direction, the next 3 components are the second size scaled direction, the next 3 components are the third size scaled direction.void function (vis_SurfMesh *surfmesh, Vobject *object, Vdouble x[3], Vdouble s[3][3])
The geometry projection callback function prototype is
The first argument is the SurfMesh object, surfmesh, the second is a user defined object, object. The third argument, enttype isvoid function (vis_SurfMesh *surfmesh, Vobject *object, Vint enttype, Vint entaid, Vdouble uh[], Vdouble xh[3], Vdouble ug[], Vdouble xg[3])
SYS_EDGE
orSYS_FACE
depending upon whether the projection is to be to a specified geometry edge or face. The argument, entaid is a unique integer identifier for the geometry edge or face. This identifier is the node association forSYS_EDGE
orSYS_FACE
specified in the functionvis_SurfMeshSetTriAssoc()
. The arguments, uh and xh are the initial parameter and global coordinate point locations. The arguments, ug and xg are the returned projected parameter and global coordinate point locations.- Parameters
p – Pointer to SurfMesh object.
funtype – Type of callback function to set
x=SYS_FUNCTION_MONITOR Monitor callback =SYS_FUNCTION_SIZING Isotropic sizing callback =SYS_FUNCTION_ASIZING Anisotropic sizing callback =SYS_FUNCTION_GEOPROJ Geometry projection callback
function – Pointer to callback function
object – Pointer to the object to be returned as function argument
-
void vis_SurfMeshSetParami(vis_SurfMesh *p, Vint ptype, Vint iparam)
set mesh generation parameters
Specify mesh generation parameters. These parameters specify the order, shape and connectivity convention to be used for the generated elements.
Parameters with a name following the regular expression SURFMESH_EXPERIMENTAL_(*) are not garantied to be part of the public API in future releases, they can evolve into non-experimental parameters or be deleted.
The parameter
VIS_MESH_MAXI
sets the order of the generated elements by specifying the number of nodes to generate along an edge. A value of 2 generates linear elements, a value of 3 generates parabolic elements and a value of 4 generates cubic elements. By defaultVIS_MESH_MAXI
is set to 2.The parameter
VIS_MESH_SHAPE
sets the shape of the generated elements. Either triangular,VIS_SHAPETRI
, or quadrilateral,VIS_SHAPEQUAD
, elements may be generated. If quadrilateral elements are to be generated it is not guaranteed that all generated elements will be quadrilateral shape, a few isolated triangular elements may be generated. By defaultVIS_MESH_SHAPE
is set toVIS_SHAPETRI
.The parameter
VIS_MESH_INTERREFINE
enables the refinement of the surface edges and faces. By defaultVIS_MESH_INTERREFINE
is set toSYS_ON
.The parameter
VIS_MESH_MAPDETECT
toggles the detection of map meshable regions. By defaultVIS_MESH_MAPDETECT
is set toVIS_OFF
.The parameter
VIS_MESH_MAPRECTONLY
toggles the generation of quadrilateral elements in mapped mesh regions only. This option is useful when quadrilateral element generation is enabled but is to be restricted to mapped mesh regions only. All other regions will be triangulated. By defaultVIS_MESH_MAPRECTONLY
is set toVIS_OFF
.The parameter
VIS_MESH_MIDSIDEPROJ
toggles the projection of midside nodes of parabolic and cubic elements. If this parameter is disabled, then all midside nodes will be placed at the midpoint between corner nodes. If the parameter is enabled then midside nodes will be projected to the underlying geometry and adjusted to satisfy possible quality constraints specified by the parameterVIS_MESH_MIDSIDEQUAL
. By defaultVIS_MESH_MIDSIDEPROJ
is set toVIS_ON
.The parameter
VIS_MESH_MIDSIDEQUAL
toggles the adjustment of midside nodes of parabolic and cubic elements to satisfy specified quality constraints. If this parameter is disabled, then midside nodes are projected to the geometry directly and are not adjusted for element quality constraints. If the parameter is enabled then the midside nodes are adjusted to ensure that all corner node Jacobians are positive and that the Jacobian ratio in any element is less than the specified upper Jacobian ratio bound. This bound is specified usingvis_SurfMeshSetParamd()
. This parameter is ignored ifVIS_MESH_MIDSIDEPROJ
is disabled. By defaultVIS_MESH_MIDSIDEQUAL
is set toVIS_ON
.The parameter
VIS_MESH_COMPUTENORMAL
toggles the computation of normals at the generated element nodes. The computed normals are entered into an ElemDat object which must be registered with the Connect object used to receive the output mesh. By defaultVIS_MESH_COMPUTENORMAL
is set toVIS_OFF
.The parameter
VIS_MESH_REFINESMOOTH
toggles the use of node smoothing during mesh refinement usingvis_SurfMeshRefine()
. By defaultVIS_MESH_REFINESMOOTH
is set toVIS_OFF
.The parameter
SURFMESH_EDGETANGSIZE
toggles the use of the spanning angle sizing due to edge curvature. By defaultSURFMESH_EDGETANGSIZE
is set toVIS_OFF
.The parameter
SURFMESH_GLOBALINTERSECT
toggles the checking and repair of surface intersections in the final mesh. This option is useful for thin walled structures which are to be subsequently volume meshed. By defaultSURFMESH_GLOBALINTERSECT
is set toVIS_OFF
.The parameter
VIS_MESH_FUNSIZINGMIN
is used to control the way final element sizing is computed when a sizing callback is installed. By default, the sizing returned by the sizing callback is used. IfVIS_MESH_FUNSIZINGMIN
is enabled, then the sizing is the minimum of the sizing callback and normal sizing. By defaultVIS_MESH_FUNSIZINGMIN
is set toVIS_OFF
.The parameter
SURFMESH_INTSURFBACK
toggles the identification of internal faces in non-manifold geometry. If this parameter is enabled, then internal surfaces are detected and back to back triangles are generated on them. This is specifically designed for subsequent input to the TetMesh or VolMesh modules. By default internal regions bound by inward pointing triangles are identified as holes. The parameterSURFMESH_INTSURFBACKNOHOLE
can be used to control hole identification. If the user flags all material boundedness withvis_SurfMeshSetTriBack()
these parameters should not be set. By defaultSURFMESH_INTSURFBACK
andSURFMESH_INTSURFBACKNOHOLE
are set toVIS_OFF
.The parameter
SURFMESH_INTSURFBACKNOHOLE
toggles the identification of internal faces in non-manifold geometry as possible holes. If this parameter is enabled, then internal regions with all bounding facets pointing inward are not detected as holes By defaultSURFMESH_INTSURFBACKNOHOLE
is set toVIS_OFF
.The parameter
SURFMESH_NONMANIFOLD
toggles the checking for non-manifold geometry. If this parameter is enabled, then non-manifold geometry is allowed otherwise it is not allowed. By defaultSURFMESH_NONMANIFOLD
is set toVIS_OFF
.The parameter
SURFMESH_SINGDETECT
toggles the checking and marking of surface singularities in the input geometry. This option is useful for detecting singularities such as the apex of conical geometry. The input points at such singularities may not be preserved and the triangle normals at the attached points may not reflect the normal discontinuity present at the point. If a singular point is found, the point is preserved, the triangle normals at the point are recomputed to represent surface normals slightly off the singularity. The mesh size at the point is set to the input minimum edge length. By defaultSURFMESH_SINGDETECT
is set toVIS_OFF
.The parameter
SURFMESH_MATE
controls mesh mating. A value ofSURFMESH_MATE_WELD
merges free edges to any coincident geometry in which no penetrations exist. A value ofSURFMESH_MATE_FREE
merges free edges to any other free edges. Use the functionvis_SurfMeshSetParamd()
to set the mating tolerance parameterSURFMESH_MATELENGTH
. By defaultSURFMESH_MATE
is set toSURFMESH_MATE_NONE
.The parameter
SURFMESH_QUADDOM
controls quad dominant meshing. By defaultSURFMESH_QUADDOM
is set toVIS_ON
.The parameter
SURFMESH_LOOPNOFRONT
can be used to flag certain small edge loops to not be an advancing front source. If set to a non-zero value, any edge loop containing a number of element edges less than or equal to the specified value will not be a front source. Generally this will result in many fewer irregular nodes in a quadrilateral mesh. By defaultSURFMESH_LOOPNOFRONT
is set to 0.The parameter
SURFMESH_LOOPWASHER
can be used to flag certain small edge loops to have a very regular single layer of quadrilateral elements generated around them. If set to a non-zero value, any edge loop containing a number of element edges less than or equal to the specified value will have a single layer washer generated. By defaultSURFMESH_LOOPWASHER
is set to 0.The parameter
SURFMESH_EXPERIMENTAL_EDGEHEAPSIZEFACT
enables experimental developements on the treatment of edges during mesh optimization, by default is set toSYS_ON
.Inquire of defined integer parameters as output arguments using
void vis_SurfMeshGetParami (vis_SurfMesh *surfmesh, Vint ptype, Vint *iparam)
- Errors
VIS_ERROR_ENUM
is generated if an improper ptype is specified.VIS_ERROR_VALUE
is generated if an improper order or shape of generated elements is specified.
- Parameters
p – Pointer to SurfMesh object.
ptype – Type of display parameter to set
x=VIS_MESH_MAXI Order of generated elements =VIS_MESH_SHAPE Shape of generated elements =VIS_MESH_COMPUTENORMAL Compute normals =VIS_MESH_INTERREFINE Interior refinement flag =SURFMESH_EDGETANGSIZE Enable spanning angle on edges =VIS_MESH_FUNSIZINGMIN Size is min'ed with sizing callback =SURFMESH_GLOBALINTERSECT Repair surface intersection =SURFMESH_INTSURFBACK Identify internal surfaces =SURFMESH_INTSURFBACKNOHOLE Do not identify internal holes =SURFMESH_NONMANIFOLD Allow non manifold geometry =VIS_MESH_MAPDETECT Detect mapped mesh regions =VIS_MESH_MAPRECTONLY Quadrilateralize mapped meshes only =VIS_MESH_MIDSIDEPROJ Project midside nodes =VIS_MESH_MIDSIDEQUAL Quality midside node adjustment =VIS_MESH_REFINESMOOTH Smooth node locations during refine =SURFMESH_SINGDETECT Detect surface singularities =SURFMESH_MATE Enable mesh mating. =SURFMESH_QUADDOM Enable quad dominant mesh =SURFMESH_LOOPNOFRONT Specify edge loop front treatment =SURFMESH_LOOPWASHER Specify edge loop washer treatment =SURFMESH_EXPERIMENTAL_EDGEHEAPSIZEFACT Enable experimental behaviour on edge enqueuing
iparam – Specifies the integer value that ptype will be set to.
x=VIS_SHAPETRI Triangle shape =VIS_SHAPEQUAD Quadrilateral shape =VIS_OFF Turn parameter off =VIS_ON Turn parameter on =SURFMESH_MATE_NONE Disable mesh mating. =SURFMESH_MATE_WELD Enable mesh mating weld. =SURFMESH_MATE_FREE Enable mesh mating free edges.
-
void vis_SurfMeshGetParami(vis_SurfMesh *p, Vint type, Vint *iparam)
get mesh generation parameters
-
void vis_SurfMeshSetParamd(vis_SurfMesh *p, Vint ptype, Vdouble dparam)
set mesh generation parameters
Specify mesh generation parameters. These parameters are used to control the quality and size of the generated elements.
The parameter
VIS_MESH_EDGELENGTH
specifies a target edge length of generated elements. The edge length constraint may not be satisfied for elements generated near preserved edges of the surface due to preserved edge sizing. By defaultVIS_MESH_EDGELENGTH
is set to 1.The parameter
VIS_MESH_MINANGLE
specifies a minimum triangle angle. In the absence of geometrical constraints, minimum angles of approximately 30. degrees may often be achieved in practice. However if there are small geometrical features or other geometry constrained regions, a minimum angle of just a few degrees may be difficult to achieve. The maximum possible minimum angle is, of course, 60. degrees. Use this option with caution, it may cause unwanted overrefinement. By defaultVIS_MESH_MINANGLE
is set to 0. degrees.The parameter
VIS_MESH_GROWTHRATE
specifies a maximum growth rate. The growth rate governs the rate at which the size of adjacent elements can grow. The ratio of edge lengths of unconstrained adjacent elements will not exceed the specified growth rate. The growth rate must be greateer than 1.001. By defaultVIS_MESH_GROWTHRATE
is set to 2.0The parameter
VIS_MESH_MAXEDGELENGTH
specifies a maximum edge length of generated elements. The maximum edge length is strictly enforced. By defaultVIS_MESH_MAXEDGELENGTH
is set to 0. and is ignored.The parameter
VIS_MESH_CHORDHEIGHT
specifies a maximum chord height tolerance for elements generated on curved surfaces. The chord height is defined as the distance from the midpoint of an element edge to the input geometry. A value of 0. turns off chord height sizing. The element sizing on curved surfaces is the minimum of the chord height and spanning angle sizings. By defaultVIS_MESH_CHORDHEIGHT
is set to 0.The parameter
VIS_MESH_SPANANGLE
specifies a maximum spanning angle in degrees for elements generated on curved surfaces.
The element sizing on curved surfaces is the minimum of the chord height and spanning angle sizings. By default
VIS_MESH_SPANANGLE
is set to 30. degrees.The parameter
VIS_MESH_MAXEDGEALT
specifies an upper bound on the edge-altitude ratio of an element. The edge-altitude ratio is defined as the maximum edge length over the smallest altitude. A series of operations are performed in an attempt to satisfy the edge-altitude ratio quality. By defaultVIS_MESH_MAXEDGEALT
is set to 100.The parameter
VIS_MESH_MAXANGLE
specifies a maximum triangle angle. By defaultVIS_MESH_MAXANGLE
is set to 135. degrees.The parameter
VIS_MESH_MAPANGLE
specifies an angular tolerance in degrees used to detect mapped mesh regions. The angle parameter is a deviation from 90 degrees for corner edges and a deviation from 180 degrees for side edges. By defaultVIS_MESH_MAPANGLE
is set to 2. degrees.The parameter
VIS_MESH_MINEDGELENGTH
specifies a minimum edge length of generated elements. Use this parameter to limit refinement around small features such as small holes, sharp corners, cone tips, etc. By defaultVIS_MESH_MINEDGELENGTH
is set to .01 of the target edge lengthVIS_MESH_EDGELENGTH
.The parameter
VIS_MESH_MAXQUADANGLE
specifies a maximum angle allowed in a quadrilateral element. Any generated quadrilateral with an angle exceeding this value will be split into two triangle elements. Note that the maximum angle can not be less than 90. degrees. By defaultVIS_MESH_MAXQUADANGLE
is set to 135. degrees.The parameter
VIS_MESH_MAXWARPANGLE
specifies a maximum warp angle allowed in a quadrilateral element.
The warp angle for a quadrilateral element is the maximum deviation from 180 degrees of the two dihedral angles between the two sets of triangles into which a quadrilateral element may be split. Any generated quadrilateral with an angle exceeding this value will be split into two triangle elements. By default
VIS_MESH_MAXWARPANGLE
is set to 15. degrees.The parameters
VIS_MESH_MINFEATLENGTH
andVIS_MESH_MINFEATANGLE
control the deletion of small features from the original input geometry. The parameterVIS_MESH_MINFEATLENGTH
is a minimum small feature size. A recommended value for the minimum small feature size is about 5 percent ofVIS_MESH_MINEDGELENGTH
. The parameterVIS_MESH_MINFEATANGLE
is a minimum small feature angle in degrees. If this parameter is set to a non zero value then the minimum small feature size should also be set. A recommended value for the minimum small feature angle is about 2 degrees. If these parameters are 0., then no small feature deletion is performed. By defaultVIS_MESH_MINFEATLENGTH
andVIS_MESH_MINFEATANGLE
are set to 0.The parameter
VIS_MESH_MAXPROXRATIO
specifies proximity base sizing for closely spaced surfaces. The associated value is a desired aspect ratio of elements generated between surfaces. A ratio greater than one will typically result in one element with the given aspect ratio connecting the closely spaced surfaces. A ratio less than one will typically generate isotropic elements with the reciprocal of the given ratio number of elements between the closely spaced surfaces. By defaultVIS_MESH_MAXPROXRATIO
is set to 0.The parameter
SURFMESH_MATELENGTH
specifies a mesh mating length. This mating length is used only ifSURFMESH_MATE
as set byvis_SurfMeshSetParami()
is on. If the length is zero then a small fraction of the model extent is used. By defaultSURFMESH_MATELENGTH
is set to 0.The
SURFMESH_PRESEDGEANGLE
andSURFMESH_PRESNODEANGLE
parameters specify feature angles in degrees which are used to internally preserve input triangle edges and nodes. TheSURFMESH_PRESEDGEANGLE
parameter is a dihedral angle tolerance. Any manifold edge between two adjacent triangles which form a dihedral angle which exceeds this parameter is preserved. TheSURFMESH_PRESNODEANGLE
parameter is an angle at a node lying on two preserved edges. Any node between two adjacent preserved edges which form an angle which exceeds this parameter is preserved.By default
SURFMESH_PRESEDGEANGLE
andSURFMESH_PRESNODEANGLE
are set to 0. which specifies that no feature edges are nodes will be preserved.Inquire of defined double precision parameters as output arguments using
void vis_SurfMeshGetParamd (vis_SurfMesh *surfmesh, Vint ptype, Vdouble *dparam)
- Errors
VIS_ERROR_ENUM
is generated if an improper ptype is specified.VIS_ERROR_VALUE
is generated if an improper value is specified.
- Parameters
p – Pointer to SurfMesh object.
ptype – Type of display parameter to set
x=VIS_MESH_EDGELENGTH Target edge length =VIS_MESH_MINEDGELENGTH Minimum edge length =VIS_MESH_MAXEDGELENGTH Maximum edge length =VIS_MESH_MAXEDGEALT Maximum edge altitude ratio =VIS_MESH_MAXANGLE Maximum triangle angle =VIS_MESH_MINANGLE Minimum triangle angle =VIS_MESH_CHORDHEIGHT Chord height =VIS_MESH_SPANANGLE Spanning angle =VIS_MESH_GROWTHRATE Maximum growth rate =VIS_MESH_MAPANGLE Mapped mesh region angle tolerance =VIS_MESH_MAXQUADANGLE Maximum quad element angle =VIS_MESH_MAXWARPANGLE Maximum quad warp angle =VIS_MESH_MINFEATLENGTH Minimum feature length =VIS_MESH_MINFEATANGLE Minimum feature angle =VIS_MESH_MAXPROXRATIO Proximity sizing =SURFMESH_MATELENGTH Mesh mating length =SURFMESH_PRESEDGEANGLE Preserved edge feature angle =SURFMESH_PRESNODEANGLE Preserved node feature angle
dparam – Specifies the value that ptype will be set to.
-
void vis_SurfMeshGetParamd(vis_SurfMesh *p, Vint type, Vdouble *param)
get mesh generation parameters
-
void vis_SurfMeshAbort(vis_SurfMesh *p)
set abort flag
Set mesh generation abort flag. During the mesh generation process in
vis_SurfMeshGenerate()
orvis_SurfMeshRefine()
this flag is intermittently checked. If it has been set, the mesh generation process terminates and returns. The abort flag is usually set in the user defined callback function specified byvis_SurfMeshSetFunction()
.- Parameters
p – Pointer to SurfMesh object.
-
void vis_SurfMeshSetPoint(vis_SurfMesh *p, Vint id, Vdouble x[3], Vint pres)
define a surface point
Set a surface point location. The point id must be in the interval 1 <= id <= numpnts where numpnts is defined by
vis_SurfMeshDef()
. Set optional point sizing usingvis_SurfMeshSetPointSizing()
and point associations usingvis_SurfMeshSetPointAssoc()
. Input surface triangles are specified usingvis_SurfMeshSetTri()
.Inquire of defined surface point as output arguments using
void vis_SurfMeshGetPoint (vis_SurfMesh *surfmesh, Vint id, Vdouble x[3], Vint *pres)
- Errors
VIS_ERROR_VALUE
is generated if an improper id is specified.
- Parameters
p – Pointer to SurfMesh object.
id – Point id
x – Point coordinate location
pres – Preserve point flag
-
void vis_SurfMeshGetPoint(vis_SurfMesh *p, Vint id, Vdouble x[3], Vint *pflag)
inquire of defined surface point
-
void vis_SurfMeshSetPointSizing(vis_SurfMesh *p, Vint id, Vdouble h)
define a point sizing
Set a point target edge length. This allows the resulting mesh density to be controlled on a point wise basis.
- Errors
VIS_ERROR_VALUE
is generated if an improper id is specified or if h is less than zero.
- Parameters
p – Pointer to SurfMesh object.
id – Point id
h – Sizing, target edge length
-
void vis_SurfMeshSetPointAssoc(vis_SurfMesh *p, Vint type, Vint id, Vint aid)
define a point association
Set a point association. The association is assigned to the node generated under the specified point. The point, id, is also marked as preserved. The type of associations allowed are those accepted by the Connect module function
vis_ConnectSetNodeAssoc()
. Usevis_SurfMeshSetTriAssoc()
to set associations to be assigned at nodes or at elements generated on an input triangle.It is recommended that for all points coincident with an underlying geometry vertex that the specific association type,
VIS_GEOVERT
be used. The point association value should be a unique integer identifier for the underlying geometry vertex.Inquire of defined surface point association as an output argument using
void vis_SurfMeshGetPointAssoc (vis_SurfMesh *surfmesh, Vint type, Vint id, Vint *aid)
- Errors
VIS_ERROR_ENUM
is generated if an improper type is specified.VIS_ERROR_VALUE
is generated if an improper id is specified.
- Parameters
p – Pointer to SurfMesh object.
type – Type of association
id – Point id
aid – Point association value
-
void vis_SurfMeshGetPointAssoc(vis_SurfMesh *p, Vint type, Vint id, Vint *aid)
inquire of defined surface point association as an output argument
-
void vis_SurfMeshSetTri(vis_SurfMesh *p, Vint id, Vint ix[], Vint eflag[])
define a surface triangle
Set a surface triangle connectivity and edge flags. The triangle id must be in the interval 1 <= id <= numtris where numtris is defined by
vis_SurfMeshDef()
. The triangle connectivity, ix, must contain 3 points.The edge flags, eflag, specify whether or not the edge of an input triangle is to be preserved in the final mesh. For edges which are connected to more than one triangle, the edge flag need only be set for one triangle to which the edge is connected. An edge shared by more than one triangle will be given the highest valued edge flag of all connected triangles. All free edges, connected to only one triangle, are implicitly preserved in the final mesh. Set optional triangle sizing using
vis_SurfMeshSetTriSizing()
and triangle associations usingvis_SurfMeshSetTriAssoc()
. Note that all triangles must be entered sequentially.Inquire of defined triangle ix and eflag as output arguments using
void vis_SurfMeshGetTri (vis_SurfMesh *surfmesh, Vint id, Vint ix[3], Vint eflag[3])
- Errors
VIS_ERROR_VALUE
is generated if an improper id is specified.VIS_ERROR_OPERATION
is generated if an id is entered out of order.VIS_ERROR_VALUE
is generated if an improper eflag is specified.
- Parameters
p – Pointer to SurfMesh object.
id – Triangle id
ix – Triangle connectivity
eflag – Triangle preserve edge flags
=0 Edge not preserved =1 Edge preserved =2 Edge preserved and purged of all unpreserved nodes =3 Edge preserved and not defeatured
-
void vis_SurfMeshGetTri(vis_SurfMesh *p, Vint id, Vint ix[], Vint eflag[])
inquire of defined triangle ix and eflag as output arguments
-
void vis_SurfMeshSetTriSizing(vis_SurfMesh *p, Vint id, Vint enttype, Vint no, Vdouble h)
define triangle face and edge sizing
Set target edge length to be enforced along a triangle edge or within a triangle face. If enttype is
SYS_FACE
, then no is ignored.- Errors
VIS_ERROR_ENUM
is generated if an improper enttype is specified.VIS_ERROR_VALUE
is generated if an improper id, no is specified or if h is less than zero.
- Parameters
p – Pointer to SurfMesh object.
id – Triangle id
enttype – Entity type
=SYS_EDGE Triangle edge =SYS_FACE Triangle face
no – Triangle edge number
h – Sizing, target edge length
-
void vis_SurfMeshSetTriDepthSizing(vis_SurfMesh *p, Vint id, Vdouble d, Vdouble h)
set sizing within a depth at a triangle
Set element size to be enforced within a specified depth of an input triangle.
- Errors
VIS_ERROR_VALUE
is generated if an improper id is specified.VIS_ERROR_VALUE
is generated if either d or h is less than zero.
- Parameters
p – Pointer to SurfMesh object.
id – Triangle id
d – Depth
h – Sizing
-
void vis_SurfMeshSetTriAssoc(vis_SurfMesh *p, Vint type, Vint id, Vint enttype, Vint no, Vint aid)
define a triangle association
Set associations to be assigned to generated nodes or elements on an edge or the face of a surface triangle, id. The type of associations allowed are those accepted by the Connect module function
vis_ConnectSetNodeAssoc()
. Usevis_SurfMeshSetPointAssoc()
to set associations to be assigned to the node generated at an input point.If enttype is
SYS_EDGE
, then node associations are assigned to all nodes generated on the specified triangle edge, no.
This association will also be generated as element entity associations along the triangle edges generated on the specified triangle edge. The input triangle edge is marked as preserved. Since it is generally possible for a preserved edge to be collapsed during the mesh generation process, any connected set of preserved edges on which a particular node association is specified should be bound by preserved nodes. It is recommended that for all triangle edges coincident with an underlying geometry edge that the specific association type,
VIS_GEOEDGE
be used. The association value should be a unique integer identifier for the underlying geometry edge.If enttype is
SYS_FACE
, then node associations are assigned to all nodes generated on the specified triangle. This association will also be generated as element entity associations along the element faces generated on the specified triangle. It is recommended that for all triangles lying on an underlying geometry face that the specific association type,VIS_GEOFACE
be used as a face association. The association value should be a unique integer identifier for the underlying geometry face.If enttype is
SYS_ELEM
, then element associations are assigned to all elements generated on the specified triangle. Since it is generally possible for the edges of adjacent triangles to be swapped during the mesh generation process, any connected set of triangles on which a particular node or element association is specified should be bound by preserved edges. It is recommended that for all triangles lying on an underlying geometry face that the specific association type,VIS_GEOFACE
be used as an element association. The association value should be a unique integer identifier for the underlying geometry face.In addition, the association type,
VIS_GEOBODY
should be used as an element association if the triangle is associated with a geometric body or bounds particular material regions.Inquire of defined triangle association as an output argument using
void vis_SurfMeshGetTriAssoc (vis_SurfMesh *surfmesh, Vint type, Vint id, Vint enttype, Vint no, Vint *aid)
- Errors
VIS_ERROR_ENUM
is generated if an improper type or enttype is specified.VIS_ERROR_VALUE
is generated if an improper id or no is specified.
- Parameters
p – Pointer to SurfMesh object.
type – Type of association
id – Triangle id
enttype – Entity type to which association is assigned
=SYS_EDGE Triangle edge for node association =SYS_FACE Triangle face for node association =SYS_ELEM Triangle for element association
no – Triangle edge or face number
>0 Triangle edge number =0 or 1 Triangle right face =-1 Triangle left face
aid – Triangle association
-
void vis_SurfMeshGetTriAssoc(vis_SurfMesh *p, Vint type, Vint id, Vint enttype, Vint no, Vint *aid)
inquire of defined triangle association as an output argument
-
void vis_SurfMeshSetTriNorm(vis_SurfMesh *p, Vint id, Vdouble v[3][3])
define a triangle vertex normals
Specify triangle vertex normals. The triangle id must be in the interval 1 <= id <= numtris where numtris is defined by
vis_SurfMeshDef()
. The triangle vertex normals, v, must contain normals for the corner nodes of the triangle.Inquire of defined triangle normals as an output argument using
void vis_SurfMeshGetTriNorm (vis_SurfMesh *surfmesh, Vint id, Vdouble v[3][3])
- Errors
VIS_ERROR_VALUE
is generated if an improper id is specified.VIS_ERROR_COMPUTE
is generated if a zero length normal is specified.
- Parameters
p – Pointer to SurfMesh object.
id – Triangle id
v – Triangle vertex normals
-
void vis_SurfMeshGetTriNorm(vis_SurfMesh *p, Vint id, Vdouble v[3][3])
inquire of defined triangle normals as an output argument
-
void vis_SurfMeshSetTriTang(vis_SurfMesh *p, Vint id, Vint no, Vdouble v[2][3])
define triangle edge vertex tangents
Specify triangle edge vertex tangents at preserved edges. The triangle id must be in the interval 1 <= id <= numtris where numtris is defined by
vis_SurfMeshDef()
. The triangle edge number, no, must be in the interval 1 <= no <= 3. Two triangle edge tangents appear in v for each edge. For example the edge tangents for triangle edge 3 would contain the tangent located at triangle vertex 3 followed by the tangent located at triangle vertex 1. The tangent vector must be in the direction of the triangle connectivity along the edge. In general a preserved edge will have a set of tangents for each element connected to it. Note that for the case of a manifold edge with two triangles, the tangents from each triangle will have opposite sign.Inquire of defined triangle tangents as an output argument using
void vis_SurfMeshGetTriTang (vis_SurfMesh *surfmesh, Vint id, Vint no, Vdouble v[2][3])
- Errors
VIS_ERROR_VALUE
is generated if an improper id or no is specified.VIS_ERROR_COMPUTE
is generated if a zero length tangent is specified.
- Parameters
p – Pointer to SurfMesh object.
id – Triangle id
no – Triangle edge number
v – Triangle edge vertex tangents
-
void vis_SurfMeshGetTriTang(vis_SurfMesh *p, Vint id, Vint no, Vdouble v[2][3])
inquire of defined triangle tangents as an output argument
-
void vis_SurfMeshSetTriHint(vis_SurfMesh *p, Vint id, Vint enttype, Vint no, Vint nid)
specify topological location of point
Specify a topological entity on which an unconnected point, nid, is assumed to lie. If the entity type is
SYS_EDGE
, then the point is inserted in the closest connected triangle edge. The search region is bounded by preserved nodes. The triangle edge hint should be a preserved edge. If the triangle id is set to zero, and the entity type isSYS_EDGE
, then a global search is done over all preserved triangle edges and the point is inserted in the closest edge.If the entity type is
SYS_FACE
, then the point is inserted in the closest connected triangle face. The search region is bounded by preserved edges. If a hint is not given for an unconnected point, a global search is done over all triangle faces and the point is inserted in the closest triangle. Note that unconnected point coordinates are not changed, the geometry is modified to include the point coordinate.- Errors
VIS_ERROR_ENUM
is generated if an improper enttype is specified.VIS_ERROR_VALUE
is generated if an improper id, no or nid is specified.
- Parameters
p – Pointer to SurfMesh object.
id – Triangle id
enttype – Entity type on which unconnected point is assumed to lie.
=SYS_EDGE Triangle edge =SYS_FACE Triangle face
no – Triangle edge number
nid – Point id
-
void vis_SurfMeshSetTriPair(vis_SurfMesh *p, Vint enttype, Vint idm, Vint nom, Vint ids, Vint nos, Vint sense)
specify paired triangles on periodic faces
Set paired triangles on the master and slave faces of a pair of periodic faces. Paired faces must have identical topology and congruent geometry. The specified triangles must have an edge on a preserved edge containing a preserved node of the face. The preserved nodes must be paired so that the two periodic faces can be oriented properly with respect to one another. The triangle node numbers indicate the position of the node in the associated triangle connectivity. They must be 1 <= nom <= 3 and 1 <= nos <= 3.
- Errors
VIS_ERROR_ENUM
is generated if an improper enttype is specified.VIS_ERROR_VALUE
is generated if an improper idm, nom, ids, nos or sense is specified.
- Parameters
p – Pointer to SurfMesh object.
enttype – Entity type
=SYS_FACE Triangle face
idm – Triangle id on master face
nom – Triangle node number on preserved node of master face
ids – Triangle id on slave face
nos – Triangle node number on preserved node of slave face
sense – Sense
=1 Faces not reflected =-1 Faces reflected
-
void vis_SurfMeshSetTriFeat(vis_SurfMesh *p, Vint id, Vint no, Vint feat, Vint flag, Vint nlay, Vdouble rate)
define triangle edge feature
Specify specialized mesh feature treatment adjacent to a specified edge. The triangle edge must reference a preserved edge, otherwise the feature specification is ignored. The feature treatment will affect all triangle edges connected to the preserved edge bound by preserved nodes. The feat types
SURFMESH_FEAT_EDGEWASHER
andSURFMESH_FEAT_EDGELAYER
are similar. Both will generate nlaylayers of elements adjacent to the specified preserved edge.
The value
SURFMESH_FEAT_EDGEWASHER
will sample the edge size of the specified triangle edge and set it on all triangle edges attached to the preserved edge bound by preserved nodes. This ensures that the layers will be of constant radial size. The valueSURFMESH_FEAT_EDGELAYER
does not coerce the edge sizes to be uniform. It is possible to generate variable size layers if the edge sizes along the preserved edge vary.- Errors
VIS_ERROR_VALUE
is generated if an improper id or no is specified.VIS_ERROR_VALUE
is generated if an improper feat, flag or rate
- Parameters
p – Pointer to SurfMesh object.
id – Triangle id
no – Triangle edge number
feat – Edge feature type
=SURFMESH_FEAT_EDGEWASHER Generate constant size layers =SURFMESH_FEAT_EDGELAYER Generate variable size layers
flag – Front suppression flag
=SYS_ON Suppress front source =SYS_OFF Do not suppress front source
nlay – Number of layers
rate – Layer size expansion rate -1. <= rate <= 1.
-
void vis_SurfMeshSetTriBack(vis_SurfMesh *p, Vint id, Vint right, Vint left)
specify material boundedness
Specify the material boundedness of an input triangle by explicitly flagging which triangle back sides face material. This function is only required for output meshes which represent solid bodies that are to be volumetrically meshed by a VisTools/Mesh volumetric meshing object.
By default material is assumed to exist only on the back side of the right-handed normal to a triangle. All triangle normals which bound material on only one side (external triangles and internal triangles which bound voids) should point out of the material region. The default material boundedness is correct for this case and it is not required that this function be called for this type of triangle. If for any reason an input triangle facing a void does not have the proper orientation, then this function should be called to explicitly specify which side of the triangle faces the material region. All internal triangles which bound two material regions should have their material boundedness specified.
- Errors
VIS_ERROR_VALUE
is generated if an improper id is specified.
- Parameters
p – Pointer to SurfMesh object.
id – Triangle id
right – Material in back of right handed normal
left – Material in back of left handed normal
-
void vis_SurfMeshSetTriConic(vis_SurfMesh *p, Vint id, Vint conicid)
specify conic associated with a triangle
Specify the conic section associated with an input triangle, id. The conic section must be defined using
vis_SurfMeshSetConic()
.- Errors
VIS_ERROR_VALUE
is generated if an impropera id is specified.
- Parameters
p – Pointer to SurfMesh object.
id – Triangle id
conicid – Associated conic section identifier
-
void vis_SurfMeshSetConic(vis_SurfMesh *p, Vint conicid, Vint type, Vint sense, Vdouble xo[3], Vdouble axis[3], Vdouble ref[3], Vdouble c, Vdouble r)
set conic sections
Define a conic section. Each section is given a positive identifier, conicid.
- Errors
VIS_ERROR_VALUE
is generated if an improper conicid, type or sense is specified.
- Parameters
p – Pointer to SurfMesh object.
conicid – Conic section identifier
type – Conic section type
x=SURFMESH_CONIC_PLANE Plane =SURFMESH_CONIC_CONE Cone =SURFMESH_CONIC_CYLINDER Cylinder =SURFMESH_CONIC_TORUS Torus =SURFMESH_CONIC_SPHERE Sphere
sense – Surface normal sense
x=1 Positive direction =-1 Negative direction
xo – Origin
axis – Axis unit vector
ref – Reference direction unit vector
c – Surface constant
r – Surface parameter
-
void vis_SurfMeshSetEdge(vis_SurfMesh *p, Vint trimflag, Vint nix, Vint ix[], Vint midsflag, Vdouble x[][3])
set edges to be recovered
Set a series of edges to be recovered in final mesh. The series of edges is specified as a connected set of nix points in vector ix. The points, ix are marked as preserved. The edge connectivity,ix, must contain at least 2 points. If the series of edges are meant to form a closed loop then ix[0] must equal ix[nix-1] and nix >= 4. If the series of edges forms a closed loop it is possible to specify that the surface outside of the loop is to be trimmed away by setting a nonzero trimflag. The outside surface relative to the trimming loop is determined by the sense of the loop. All surface to the right as one traverses the loop lies on the outside. Note that a trimmed closed loop which has a clockwise sense in the plane of the surface would result in a hole being created by the loop.
The midside node locations, x, may be optionally specified. If midsflag is enabled then nix-1 midside node locations must be entered in x. These midside node locations will be enforced exactly in the final mesh. If linear elements are being generated then the specified midside node locations are unused. If midsflag is not enabled, then x is ignored and may be entered as NULL.
It is possible that an edge between the two specified points may not be able to be recovered. This may occur if a preserved edge intersects the edge between two points or the curvature of the surface is very high between two points. In this case use
vis_SurfMeshGetInteger()
to query for the number of unrecovered edges.- Errors
VIS_ERROR_VALUE
is generated if an improper nix is specified.VIS_ERROR_OPERATION
is generated if trimflag is set and the edge set does not form a closed loop, ie. ix[0] does not equal ix[nix-1];VIS_ERROR_OPERATION
is generated if two adjacent points are repeated in ix.
- Parameters
p – Pointer to SurfMesh object.
trimflag – Toggle trimming of surface outside of edge set
nix – Number of points on edge set, nix >= 2
ix – Edge set connectivity
midsflag – Flag to specify midside node locations, x
=SYS_OFF Midside node locations not specified =SYS_ON Midside node locations specified
x – Midside node locations
-
void vis_SurfMeshSetGeomSizing(vis_SurfMesh *p, Vint type, Vdouble xo[3], Vdouble xa[3], Vdouble xb[3], Vdouble d[3], Vdouble h)
specify sizing within geometric shape
Set element size to be enforced within a geometric shape.
- Errors
VIS_ERROR_ENUM
is generated if an improper type is specified.
- Parameters
p – Pointer to SurfMesh object.
type – Geometry type
x=VIS_MESH_GEOMSPHERE Ellipsoid =VIS_MESH_GEOMCYLINDER Ellipsoidal cylinder =VIS_MESH_GEOMBOX Box
xo – Coordinates of geometry origin
xa – Coordinates of point along x’ axis
xb – Coordinates of point in x’y’ plane
d – Half length of geometric shape along each axis
h – Sizing
-
void vis_SurfMeshGetConnect(vis_SurfMesh *p, vis_Connect **connect)
get internal Connect object
Get the internal Connect object which contains the user input nodes and triangles. The Connect object must not be changed by the user.
-
void vis_SurfMeshWrite(vis_SurfMesh *p, Vint type, const Vchar *path)
write surface description to file
Write a geometry description to a file which has been input to SurfMesh. The contents of the file is intended for internal use only. This function should be called after all geometry input and meshing parameters have been set to ensure that an accurate geometry description including correct meshing parameters is written. The binary file format is preferred for accuracy and generally results in smaller file sizes. The primary use of this function is to create a file of the complete surface description in the case that the SurfMesh module fails to properly generate a mesh. This file can then be transmitted to Visual Kinematics for debugging purposes. It is suggested that a file extension of .srf be used for ASCII files and .bsrf be used for BINARY files.
- Errors
SYS_ERROR_ENUM
is generated if an improper type is specified.SYS_ERROR_FILE
is generated if the file can not be opened.SYS_ERROR_OPERATION
is generated if the input surface has not been defined.
- Parameters
p – Pointer to SurfMesh object.
type – File type
x=SYS_ASCII ASCII format =SYS_BINARY Binary format
path – File path
-
void vis_SurfMeshRead(vis_SurfMesh *p, Vint type, const Vchar *path)
read surface description from file
Read a surface description from a file which has been previously written by
vis_SurfMeshWrite()
. The format of the file is not published and is intended for internal use only.- Errors
SYS_ERROR_ENUM
is generated if an improper type is specified.SYS_ERROR_FILE
is generated if the file can not be opened.SYS_ERROR_FORMAT
is generated if the file contents are not correctly formatted or if a file written by a later version of SurfMesh is read.
- Parameters
p – Pointer to SurfMesh object.
type – File type
x=SYS_ASCII ASCII format =SYS_BINARY Binary format
path – File path
-
void vis_SurfMeshComputeArea(vis_SurfMesh *p, Vdouble *area)
compute area
Compute area of input tesseleation surface.
- Parameters
p – Pointer to SurfMesh object.
area – [out] Computed area
-
void vis_SurfMeshGenerate(vis_SurfMesh *p, vis_Connect *connect)
generate finite element mesh
Generate interior nodes and elements. The generated nodes and elements are appended to any existing nodes and elements in the input Connect object. Use
vis_SurfMeshGetInteger()
to query for detailed information about the mesh generation process including errors.- Errors
VIS_ERROR_OPERATION
is generated if the mesh generation fails.
-
void vis_SurfMeshRefine(vis_SurfMesh *p, vis_State *state, vis_Connect *connect)
refine a existing finite element mesh
Refine a previously generated or refined mesh. The input State object must be an element scalar state of target element edge lengths. The number of elements in State must be exactly equal to the number of elements resulting from the last call to
vis_SurfMeshGenerate()
orvis_SurfMeshRefine()
. If an element edge length is zero, it is ignored. The refinement process does not coarsen the mesh, all previously existing element corner nodes are preserved and by default their coordinate locations are unaltered. Usevis_SurfMeshSetParami()
with parameterVIS_MESH_REFINESMOOTH
to control smoothing node locations.The generated nodes and elements are appended to any existing nodes and elements in the input Connect object. Use
vis_SurfMeshGetInteger()
is query for detailed information about the mesh generation process including errors.- Errors
VIS_ERROR_OPERATION
is generated if the input State object is not an element scalar state with the proper number of element entities.VIS_ERROR_OPERATION
is generated if the mesh generation fails.
-
void vis_SurfMeshGetInteger(vis_SurfMesh *p, Vint type, Vint iparam[])
get integer mesh generation information
Query for integer mesh generation information. This function is useful for determining where errors in the mesh generation procedure occurred during a call to
vis_SurfMeshGenerate()
.The query type
SURFMESH_NUMNONMAN
returns the number of non-manifold triangles detected in the input. The querySURFMESH_NONMAN
will return the list of non-manifold triangles detected.The query type
SURFMESH_NUMINCONS
returns the number of inconsistent triangles detected in the input. Inconsistent triangles are adjacent triangles, sharing an unpreserved edge, which have their triangle connectivity senses in opposite directions. The querySURFMESH_INCONS
will return the list of inconsistent triangles detected.The query type
SURFMESH_NUMFREEEDGE
returns the number of triangles with free edges detected in the input. The querySURFMESH_FREEEDGE
will return the list of free edge triangles detected.The query type
SURFMESH_NUMNEGJAC
returns the number of generated elements with negative corner Jacobians. This check is only performed if it is enabled usingvis_SurfMeshSetParami()
.The query type
SURFMESH_NUMINTERSECT
returns the number of triangles intersected by other triangles, ie. other triangle edges, detected in the input. The querySURFMESH_INTERSECT
will return the list of intersected triangles.The query type
SURFMESH_NUMINTERSECTPAIR
returns the number of pairs of intersecting triangles detected in the input. This is similar to theSURFMESH_NUMINTERSECT
query except that pairs of intersecting triangles are returned. The querySURFMESH_INTERSECTPAIR
will return the list of pairs of intersecting triangles. Note that the array of triangles returned needs large enough to hold 2 times the number of triangle pairs triangles.The query type
SURFMESH_NUMUNPURGED
returns the number of unpurged points remaining. This query is only valid if a triangle edge has been flagged invis_SurfMeshSetTri()
to be purged of unpreserved points. This purging is not always possible and this query returns the number of points which failed to be purged. The querySURFMESH_UNPURGED
will return the list of unpurged points detected.The query type
SURFMESH_NUMUNREC
returns the number of unrecovered edges remaining. This query is only valid if a n edge has been flagged invis_SurfMeshSetEdge()
to be recovered in the final mesh. This edge recovery is not always possible and this query returns the number of edges which failed to be recovered. The querySURFMESH_UNREC
will return the list of endpoints of the unrecovered edges. There are two endpoints returned for each unrecovered edge.The query type
SURFMESH_NUMPENTRATE
returns the number of input triangles which penetrate one another ifSURFMESH_MATE
has been set toSURFMESH_MATE_WELD
. Normally penetrating (a subset of intersecting) triangles are allowed, but when this type of mating is enabled and a penetration is detected, the meshing process will terminate. The querySURFMESH_PENETRATE
will return the list of penetrating triangles.The query type
SURFMESH_NUMUNCONNECT
returns the number of input points which are not connected to any input triangles. The querySURFMESH_UNCONNECT
will return the list of unconnected points.The query type
VIS_MESH_PROGRESS
returns information about the current state of the meshing process. This function is most useful when called from the monitor function. The following four integer values are returned.iparam[0] Meshing phase Phase 1 - Geometry loading and verification Phase 2 - Geometry healing and defeaturing Phase 3 - Size map computation Phase 4 - Initial triangle mesh Phase 5 - Triangle mesh smoothing and cleanup Phase 6 - Quadrilateral morph of triangle mesh Phase 7 - Quadrilateral meshing smoothing and cleanup Phase 101 - Refine Pre-processing Phase 102 - Refine point insertion Phase 103 - Refine Mesh quality improvement iparam[1] Current number of nodes iparam[2] Current number of elements iparam[3] Estimate percent completion
- Errors
VIS_ERROR_ENUM
is generated if an improper type is specified.
- Parameters
p – Pointer to SurfMesh object.
type – Type of integer information to query
x=SURFMESH_NUMNONMAN Number of non-manifold triangles =SURFMESH_NONMAN Non-manifold triangles =SURFMESH_NUMINCONS Number of inconsistent triangles =SURFMESH_INCONS Inconsistent triangles =SURFMESH_NUMFREEEDGE Number of free edge triangles =SURFMESH_FREEEDGE Free edge triangles =SURFMESH_NUMNEGJAC Number of negative Jacobians =SURFMESH_NUMINTERSECT Number of intersected triangles =SURFMESH_INTERSECT Intersected triangles =SURFMESH_NUMINTERSECTPAIR No. of intersecting triangle pairs =SURFMESH_INTERSECTPAIR Intersecting triangle pairs =SURFMESH_NUMUNPURGED Number of unpurged points =SURFMESH_UNPURGED Unpurged points =SURFMESH_NUMUNREC Number of unrecovered edges =SURFMESH_UNREC Endpoints of unrecovered edges =SURFMESH_NUMPENETRATE Number of penetrating triangles =SURFMESH_PENETRATE Penetrating triangles =SURFMESH_NUMUNCONNECT Number of unconnected points =SURFMESH_UNCONNECT Unconnected points =VIS_MESH_PROGRESS Current meshing progress
iparam – [out] Returned integer information